Question
Python beginner code: In this assignment, you will work with bags. Begin with the useBag.py starter file. This file contains comment instructions that tell you
Python beginner code:
In this assignment, you will work with bags.
Begin with the "useBag.py" starter file. This file contains comment instructions that tell you where to add your code to do various tasks that use and manipulate bags.
Make sure you read the "Guidance" document.
Make sure your output matches the "Expected Output" document.
Files:
File: useBag.py
This program exercises bags.
The following files must be in the same folder: abstractcollection.py abstractbag.py arraybag.py arrays.py ball.py linkedbag.py node.py """
#
# Replace
# IMPORTANT NOTE: # We can use a simple "for" loop to traverse a collection. # We will call this "regular iteration" or, for brevity, simply "iteration". # During a regular iteration, you cannot add or remove an item from a framework collection! # Breaking this rule will cause an immediate exception to occur. # The exception will display a message and terminate (crash) your program! # The displayed message is: "Illegal modification of backing store". # Note that you can update item attributes during iteration. # Later in this course, we will learn about special "list iterators". # Unlike regular iterators, list iterators allow modification (add and remove) during iteration.
from arraybag import ArrayBag from ball import Ball from linkedbag import LinkedBag from node import Node
# Part 1: # This function takes a bag that has red and blue balls in it # and moves the balls to two other bags: one to hold the red # balls and one to hold the blue balls. Every red ball is inflated # to twice its radius before being added to its new bag. # # Preconditions: # bag - an ArrayBag containing zero or more red and blue Ball objects. # # Postconditions: # returns - a bag containing the doubled radius red balls and # a bag containing the blue balls. # The original bag should be emptied. def distributeBag(bag): redBag = ArrayBag() blueBag = ArrayBag()
# Move the balls to the appropriate bags:
#
# Return the 2 bags: return (redBag, blueBag)
# Part 2: # This function prints the items in a bag, each on a separate line. def printBag(bag): #
# Part 3: # This function removes duplicate items from a bag. # Postconditions: # Any item that appears more than once will be reduced to a single occurrence. # Example: If there were 3 of item A, there will only be 1 of item A remaining. def removeDuplicates(bag): #
# Test 1: print("Test 1:") bag1 = ArrayBag([Ball("red", 10), Ball("red", 11), Ball("red", 12), Ball("blue", 15), Ball("blue", 15), Ball("blue", 15)]) print("Original mixed bag:") printBag(bag1) redBag, blueBag = distributeBag(bag1) print("Red bag:") printBag(redBag) print("Blue bag:") printBag(blueBag) print("Final mixed bag:") printBag(bag1)
# Test 2: print("Test 2:") bag2 = ArrayBag([Ball("red", 201), Ball("red", 201), Ball("red", 202), Ball("red", 202)]) print("Original mixed bag:") printBag(bag2) redBag, blueBag = distributeBag(bag2) print("Red bag:") printBag(redBag) print("Blue bag:") printBag(blueBag) print("Final mixed bag:") printBag(bag2)
# Test 3: print("Test 3:") bag3 = ArrayBag() print("Original mixed bag:") printBag(bag3) redBag, blueBag = distributeBag(bag3) print("Red bag:") printBag(redBag) print("Blue bag:") printBag(blueBag) print("Final mixed bag:") printBag(bag3)
# Test 4: print("Test 4:") bag4 = LinkedBag(["apple", "apple", "banana", "kiwi", "cantaloupe", "pear", "banana", "orange", "orange", "cantaloupe", "apple", "lemon", "lime", "lime"]) print("Original bag with duplicates:") printBag(bag4) removeDuplicates(bag4) print("Bag after removing duplicates:") printBag(bag4)
File: ball.py the Ball class """
class Ball:
# Class variable DEFAULT_RADIUS = 1
# Constructor: def __init__(self, color, radius = DEFAULT_RADIUS): self.color = color; self.radius = radius;
# Special methods: def __eq__(self, other): if self is other: return True if (type(self) != type(other)) or \ (self.color != other.color) or \ (self.radius != other.radius): return False return True
def __str__(self): return (self.color + " ball, radius " + str(self.radius))
# Accessor methods: def getColor(self): return self.color
def getRadius(self): return self.radius
# Mutator methods: def setColor(self, color): self.color = color
def setRadius(self, radius): self.radius = radius
File: testBall.py
Tests the Ball class. """
from ball import Ball
ball1 = Ball("blue") ball2 = Ball("blue", 1) ball3 = Ball("red", 2) ball4 = Ball("red", 5)
print("ball1:", ball1) print("ball2:", ball2) print("ball3:", ball3) print("ball4:", ball4) print()
print("Check if ball1 is equal to ball2:") print(ball1 == ball2) print()
print("Check if ball3 is equal to ball4:") print(ball3 == ball4) print()
print("Change size of ball2:") ball2.setRadius(3) print(ball2) print()
print("Check if ball1 is NOT equal to ball2:") print(ball1 != ball2) print()
print("Change color of ball4:") ball4.setColor("purple") print(ball4) print()
Activity 3 - Guidance ================================= IMPORTANT NOTE: We can use a simple "for" loop to traverse a collection. We will call this "regular iteration" or, for brevity, simply "iteration". During a regular iteration, you cannot add or remove an item from a framework collection! Breaking this rule will cause an immediate exception to occur. The exception will display a message and terminate (crash) your program! The displayed message is: "Illegal modification of backing store". Note that you can update item attributes during iteration. Later in this course, we will learn about special "list iterators". Unlike regular iterators, list iterators allow modification (add and remove) during iteration. Part 1 ====== Read the important note at the beginning of this guidance document. distributeBag() function ------------------------ Read the function header to understand what this function does. Pay particular attention to its preconditions and postconditions. You need to add your code to this function where indicated. Do not change any of its existing code. Note that this function's return statement returns 2 objects: The red bag and the blue bag objects. Returning multiple objects in a single return statement is a unique Python feature. The balls --------- The balls in the bags are objects of class Ball. The Ball class is provided in the assignment Starter Files folder. Also provided there is a ball test program, which makes it easy to see how to use a ball. A red ball's color is the string "red". A blue ball's color is the string "blue". Moving the balls ---------------- Moving the balls means they need to end up in the right bag. It also means they should not be in the starting bag after your code completes. For each test case, a reference to the starting bag is passed in as a function parameter. Changes you make to this bag parameter result in changes to the bag used at the point of call. This is because parameters in Python are passed by reference, rather than by copied value. Do NOT hard-code a separate block of code for each starter file test case. Instead, your single solution should work for ANY test cases. You should use a for loop on the original mixed bag. Do NOT attempt to remove the balls from the mixed bag as you see them during this iteration. You must wait until after all the ball references have been added to the unicolor bags. Then, the mixed bag can be emptied (cleared) by a single function call. Using references is a shallow copy of the balls. This is OK here because you will be removing the ball references from the mixed bag. This means there will not be any duplicate references in different bags to worry about. Keep it simple -------------- Do not over think/over complicate this. Solutions are simple and easy to understand. There is more than one possible coding solution. Part 2 ====== printBag() function ------------------- This function takes a bag as a paramater. Look at the expected output document to see what it is supposed to print. Notice that it must print "empty" if the bag is empty. If the bag is not empty, it must iterate through the given bag. Each item must be printed on a separate line. Notice that it must print a blank line after the last line. Part 3 ====== Read the important note at the beginning of this guidance document. Here are some hints: - bag.remove(item) removes a single occurrence of item each time it is called. - You can nest one loop inside another loop. - Your outer loop can be a "while True:" loop. - Your inner loop can be a "for item in bag:" iterator loop. - You can use a "break" statement to exit a loop. - You can use a "return" statement to exit a function. - You can use a variable named "count". You will have to figure out how to put all of this together so it works. Your code can be created in as few as 9 lines of code.
Expected output:
Test 1: Original mixed bag: red ball, radius 10 red ball, radius 11 red ball, radius 12 blue ball, radius 15 blue ball, radius 15 blue ball, radius 15 Red bag: red ball, radius 20 red ball, radius 22 red ball, radius 24 Blue bag: blue ball, radius 15 blue ball, radius 15 blue ball, radius 15 Final mixed bag: empty Test 2: Original mixed bag: red ball, radius 201 red ball, radius 201 red ball, radius 202 red ball, radius 202 Red bag: red ball, radius 402 red ball, radius 402 red ball, radius 404 red ball, radius 404 Blue bag: empty Final mixed bag: empty Test 3: Original mixed bag: empty Red bag: empty Blue bag: empty Final mixed bag: empty Test 4: Original bag with duplicates: lime lime lemon apple cantaloupe orange orange banana pear cantaloupe kiwi banana apple apple Bag after removing duplicates: lime lemon orange pear cantaloupe kiwi banana apple
May you make sure it compiles correctly?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
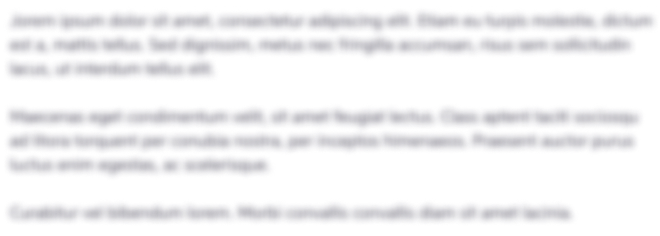
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started