Question
PYTHON, CGI scripting. Please kindlly refer to both scripts below to answer the above exercise on CGI scripting... SCRIPT 1: player.py This script models
PYTHON, CGI scripting.
Please kindlly refer to both scripts below to answer the above exercise on CGI scripting...
"""
SCRIPT 1: player.py
This script models the 2-player board game mancala. """
from random import randint from board import Board
class Player(object): """ A player makes successive moves in the game. """
def __init__(self, brd, nbr, lvl): """ A player gets access to the board and is identified with a number nbr, which is either zero or one, corresponding to the row on the board. """ self.brd = brd # the game board self.idn = nbr # the row on the board identifies the player self.ply = lvl # level of difficulty
def __str__(self): """ Returns the string representation of the player. """ result = 'Player ' + str(self.idn) \ + ' plays at level ' + str(self.ply) \ + ' at board: ' + str(self.brd) return result
def __repr__(self): """ Returns the string representation. """ return str(self)
def prompt4hole(self): """ Prints the board and prompts the player for the index of a hole. If the move is valid, then returns the index. Otherwise the player is asked to retry. """ while True: print(self.brd) try: hole = int(input('enter 1, 2, 3, 4, 5, 6 : ')) if self.brd.valid(self.idn, hole): return hole else: print(hole, 'is an invalid choice, please retry.') except ValueError: print('Invalid input, please retry.')
def randomhole(self, verbose=True): """ Generates a random index of a hole, retries if not valid, and if verbose, prints the board and the index of the hole before returning the index. """ while True: hole = randint(1, 6) if self.brd.valid(self.idn, hole): if verbose: # print(self.brd) print('Player', self.idn, 'chooses hole '+str(hole)+'.') return hole
def play(self, verbose=True, seeExtra=True): """ Selects a hole and goes through the moves. If verbose, then extra information about the moves is shown. if seeExtra, then we see the intermediate boards at the extra turns of the computer player. """ while not self.brd.emptyside(): if(self.ply == 0): hole = self.randomhole() elif(self.ply > 0): (hole, val) = self.bestmove(0, self.ply, verbose) if verbose: print('-> the best move is hole', hole) else: hole = self.prompt4hole() stones = self.brd.pick(self.idn, hole-1) if verbose: print('Player', self.idn, 'picks up', stones, 'stone(s).') (row, idx) = self.brd.drop(self.idn, hole-1, stones, self.idn) #if verbose: # print('Board after dropping stones : ' + str(self.brd)) if idx in range(6): self.brd.copy(row, idx, self.idn) if verbose: if(self.idn == row) and (self.brd.holes[row][idx] == 1): print('Player', self.idn, 'got stones from opponent.') # print('Board after moving stones : ' + str(self.brd)) break else: if verbose: print('Player', self.idn, 'gets an extra turn.') if self.ply != -1 or verbose: if seeExtra: print(self.brd)
def bestmove(self, level, maxlvl, verbose=True): """ Evaluates the six possible moves and returns the best choice as the first element in the tuple. The second element in the tuple on return is the value of the best choice. """ bestval = None for hole in range(1, 7): if self.brd.valid(self.idn, hole): backup = self.brd.deepcopy() if verbose: print('evaluating choice', hole) stones = self.brd.pick(self.idn, hole-1) (row, idx) = self.brd.drop(self.idn, hole-1, stones, self.idn) if idx in range(6): self.brd.copy(row, idx, self.idn) oppidn = (self.idn + 1) % 2 val = self.brd.stores[self.idn] - self.brd.stores[oppidn] if level bestval: (besthole, bestval) = (hole, val) if verbose: print('value at hole', hole, 'is', val) print('best value :', bestval, 'at hole', besthole) self.brd.holes = backup.holes # restore the previous board self.brd.stores = backup.stores # but leave the outer brd return (besthole, bestval)
def final(brd): """ Determines the winner of the game. """ if brd.emptyside(): brd.finish() print('The final board : ' + str(brd)) if brd.stores[0] > brd.stores[1]: print('Player 0 wins.') elif brd.stores[0]
def randomplay(level, verbose=True): """ Both players make random moves. A coin flip decides who begins. """ brd = Board() print(brd) zero = Player(brd, 0, level) one = Player(brd, 1, level) cnt = randint(0, 1) while not brd.emptyside(): if cnt == 0: zero.play(verbose) print(brd) if brd.emptyside(): break one.play(verbose) else: one.play(verbose) print(brd) if brd.emptyside(): break zero.play(verbose) print(brd) ans = input('Continue to the next round ? (y) ') if ans != 'y': break final(brd)
def interactiveplay(level, verbose=True): """ The user plays agains player zero. A coin flip decides who begins. """ brd = Board() zero = Player(brd, 0, level) one = Player(brd, 1, -1) cnt = randint(0, 1) if cnt == 0: print(brd) while not brd.emptyside(): if cnt == 0: zero.play(verbose) if brd.emptyside(): break one.play(verbose) print(brd) else: one.play(verbose) if brd.emptyside(): break print(brd) zero.play(verbose) # print(brd) # ans = input('Continue to the next round ? (y) ') # if ans != 'y': # break final(brd)
def main(): """ Runs through the mancala game. """ print('Welcome to our mancala game!') ans = input('Run with two computer players ? (y) ') lvl = int(input('Give the level of difficulty (>= 0) : ')) vrb = input('Verbose mode ? (y) ') verbose = (vrb == 'y') if ans == 'y': randomplay(lvl, verbose) else: interactiveplay(lvl, verbose)
if __name__ == "__main__": main()
""" SCRIPT 2: board.py
""" In the game of mancala stones are picked up and dropped in successive holes, in counter clock wise fashion. """ class Board(object): """ Models the board used for the mancala game. The board has two rows and two stores. Each row has six holes. To each row corresponds a store. The holes are indexed from 0 to 5, while the corresponding moves are labeled from 1 to 6. """ def __init__(self, **parameters): """ Initializes the board and the stores. Without parameters, every hole in the board gets 4 stones and the stores of the player one and two are set to zero. The user can specify the number of stones in rowone and rowtwo by giving a list of numbers following the keywords rowone and rowtwo. The values of the stores can be set with the keywords storeone and storetwo. """ self.holes = [] if 'rowone' in parameters: self.holes.append(parameters['rowone']) else: self.holes.append([4 for _ in range(6)]) if 'rowtwo' in parameters: self.holes.append(parameters['rowtwo']) else: self.holes.append([4 for _ in range(6)]) self.stores = [] if 'storeone' in parameters: self.stores.append(parameters['storeone']) else: self.stores.append(0) if 'storetwo' in parameters: self.stores.append(parameters['storetwo']) else: self.stores.append(0)
def __str__(self): """ Returns the string representation of the board. """ result = ' %2d' % self.stores[0] for item in self.holes[0]: result = result + ' %2d' % item result = result + ' ' for item in self.holes[1]: result = result + ' %2d' % item result = result + ' %2d' % self.stores[1] # result = result + ' ' # for k in range(1, 7): # result = result + ' %2d' % k return result
def __repr__(self): """ Returns the string representation. """ return str(self)
def deepcopy(self): """ Returns a new object which is a deep copy. """ from copy import deepcopy copyone = deepcopy(self.holes[0]) copytwo = deepcopy(self.holes[1]) return Board(rowone=copyone, storeone=self.stores[0], \ rowtwo=copytwo, storetwo=self.stores[1])
def valid(self, row, move): """ A move on a row is valid if move is an integer in range(1, 7) and if there is a stone in the corresponding hole. Returns True if move is valid, returns False otherwise. """ if row not in [0, 1]: return False else: if move not in range(1, 7): return False else: return (self.holes[row][move-1] > 0)
def pick(self, row, hole): """ Returns all stones out of the hole with index hole from the row with index row. The number of stones afterwards at the hole of the row will be zero. """ result = self.holes[row][hole] self.holes[row][hole] = 0 return result
def instore(self, player, idx): """ Drops a stone in the store if idx 5 and player == 1. The index on return is not in range(6) if a stone was dropped in a store, otherwise the index on return is the index of the hole where a stone was dropped. """ if(idx
def drop(self, row, hole, stones, player): """ Drops a sequence of stones lifted from the hole at the row. Returns the row and the hole where the last stone was dropped. """ idx = hole for cnt in range(stones): idx = (idx+1 if row == 1 else idx-1) if idx in range(6): self.holes[row][idx] += 1 else: idx = self.instore(player, idx) if cnt
def copy(self, row, hole, player): """ Given in row and hole the position of the last dropped stone, which is not a store, checks if the stones on the opposite row can be moved to the store. """ if player == row: if self.holes[row][hole] == 1: opprow = (row + 1) % 2 self.stores[row] += self.holes[opprow][hole] self.holes[opprow][hole] = 0
def emptyside(self): """ Returns True if all holes on one side are empty. """ if(sum(self.holes[0]) == 0 or sum(self.holes[1]) == 0): return True else: return False
def finish(self): """ Moves all stones to the store on the right. """ self.stores[0] += sum(self.holes[0]) self.stores[1] += sum(self.holes[1]) for k in range(6): self.holes[0][k] = 0 self.holes[1][k] = 0
def test(brd): """ Prompts the user for an index and executes the move on the board. """ move = int(input('Enter 1, 2, 3, 4, 5, 6 : ')) player = int(input('Give a row, either 0 or 1 : ')) if not brd.valid(player, move): print('The move is not valid.') else: print('The move is valid.') stones = brd.pick(player, move-1) (row, idx) = brd.drop(player, move-1, stones, player) print('Last stone in row', row, 'and hole', idx+1) if idx in range(6): if(player == row) and (brd.holes[row][idx] == 1): print('The player gets stones from opposite row.') brd.copy(row, idx, player) else: print('The player gets an extra turn.') print('The board after the move :') print(brd)
def main():
""" Runs some tests on the board. There are three tests: (1) Check if the counterclock wise drops are correct, with the skipping of the store of the opponent. (2) Check if the player will get an extra turn if the last stone lands in the store of the player. (3) Check if the player gets the stones from the opposite row if the last stone lands in an empty hole. """ print('The initial board :') brd = Board() print(brd) test(brd) print('A test board :') brd = Board(rowone = [5, 0, 3, 2, 6, 7], storeone = 2, \ rowtwo = [0, 8, 2, 3, 0, 5], storetwo = 3) print(brd) test(brd)
if __name__ == "__main__": main()
KEYWORD: Python, cgi scripting
The goal of this exercise is to make a simple web interface to play the game of mancala. The user (referred to as player 1) plays aeainst (player 0 the computer). The first thing is that user can select the difficulty evel. The default walue for the selection equals two. To set this default use selected in Koption value 2 the game of mancala Welcome to the game of mancala. Select the difficulty level 20 Submit The levels range from 0 to 5. The submit acknowledges the selection, prints the board the game of mancala Playing mancala at level2. 0 444 44 4 4 4 4 4 40 1 2 3 4 5 6 submit The board is represented as an HTML table. The numbers in each tabled element are right aligned (use d align hto) and for numbers less than 10, a nonbreaking space (represented as ) is inserted. An input element of size 2 allows the user to submit the choice of the hole. The user always starts the game. Typing 3 in the input elementgives the user an extra turn. the game of mancala Playing mancala at level 2. You get an extra turn. 0 4 444 44 440 5 55 1 2 3 4 5 6 Submit If the user hits submit without entering a number, then the message Enter a number between 1 and 6 ppears. If the enters a character, tside the index to empty hole, then the wrong input is repeated, as shown belowi the game of mancala Playing mancala at level 2. Your input "a" is not valid. Enter a number between 1 and 6. 266 7 005 440 0 6 6 2 1 2 3 4 5 6 Submit After each submit of the index of ah the board gets adjusted and the computer play computes the next move. The next board the user then sees is after the move of the computer The string representation of the board is passed from one form to the next via a hidden field of an input element, The difficulty level can be passed as a hidden field, for example, with the statement print cinput type "hidden" name "level" lue "%s'' str (level)) where level is the name of the variable that holds the integer value for the difficulty level At the end of the game the final board is shown preceded by the message Game over You win. in ease the user wins. If the user lost, then the message should be Game over. You lose In case of a draw, the message is The same ended n a draw. Some important points The solution consists of one file webmancala.py The goal of this exercise is to make a simple web interface to play the game of mancala. The user (referred to as player 1) plays aeainst (player 0 the computer). The first thing is that user can select the difficulty evel. The default walue for the selection equals two. To set this default use selected in Koption value 2 the game of mancala Welcome to the game of mancala. Select the difficulty level 20 Submit The levels range from 0 to 5. The submit acknowledges the selection, prints the board the game of mancala Playing mancala at level2. 0 444 44 4 4 4 4 4 40 1 2 3 4 5 6 submit The board is represented as an HTML table. The numbers in each tabled element are right aligned (use d align hto) and for numbers less than 10, a nonbreaking space (represented as ) is inserted. An input element of size 2 allows the user to submit the choice of the hole. The user always starts the game. Typing 3 in the input elementgives the user an extra turn. the game of mancala Playing mancala at level 2. You get an extra turn. 0 4 444 44 440 5 55 1 2 3 4 5 6 Submit If the user hits submit without entering a number, then the message Enter a number between 1 and 6 ppears. If the enters a character, tside the index to empty hole, then the wrong input is repeated, as shown belowi the game of mancala Playing mancala at level 2. Your input "a" is not valid. Enter a number between 1 and 6. 266 7 005 440 0 6 6 2 1 2 3 4 5 6 Submit After each submit of the index of ah the board gets adjusted and the computer play computes the next move. The next board the user then sees is after the move of the computer The string representation of the board is passed from one form to the next via a hidden field of an input element, The difficulty level can be passed as a hidden field, for example, with the statement print cinput type "hidden" name "level" lue "%s'' str (level)) where level is the name of the variable that holds the integer value for the difficulty level At the end of the game the final board is shown preceded by the message Game over You win. in ease the user wins. If the user lost, then the message should be Game over. You lose In case of a draw, the message is The same ended n a draw. Some important points The solution consists of one file webmancala.pyStep by Step Solution
There are 3 Steps involved in it
Step: 1
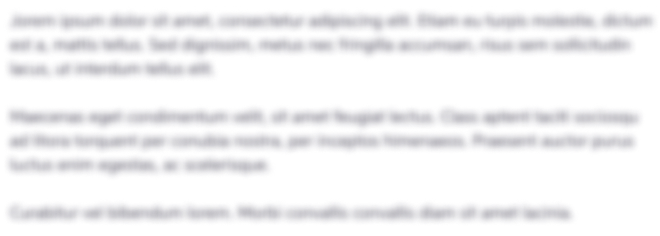
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started