Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Python given two numbers n and k, place the n queens on the n x n board so that there are exactly k pairs of
Python
given two numbers n and k, place the n queens on the n x n board so that there are exactly k pairs of queens which attack each other. For example, if n=4 and k=6, you can put all queens on one row
This code needs to be modified. It is implemented with something like
depth_first_tree_search(NQueensProblem(8))
This is from
https://github.com/aimacode/aima-python
-----------
class NQueensProblem(Problem): """The problem of placing N queens on an NxN board with none attacking each other. A state is represented as an N-element array, where a value of r in the c-th entry means there is a queen at column c, row r, and a value of -1 means that the c-th column has not been filled in yet. We fill in columns left to right. >>> depth_first_tree_search(NQueensProblem(8)) """ def __init__(self, N): super().__init__(tuple([-1] * N)) self.N = N def actions(self, state): """In the leftmost empty column, try all non-conflicting rows.""" if state[-1] != -1: return [] # All columns filled; no successors else: col = state.index(-1) return [row for row in range(self.N) if not self.conflicted(state, row, col)] def result(self, state, row): """Place the next queen at the given row.""" col = state.index(-1) new = list(state[:]) new[col] = row return tuple(new) def conflicted(self, state, row, col): """Would placing a queen at (row, col) conflict with anything?""" return any(self.conflict(row, col, state[c], c) for c in range(col)) def conflict(self, row1, col1, row2, col2): """Would putting two queens in (row1, col1) and (row2, col2) conflict?""" return (row1 == row2 or # same row col1 == col2 or # same column row1 - col1 == row2 - col2 or # same \ diagonal row1 + col1 == row2 + col2) # same / diagonal def goal_test(self, state): """Check if all columns filled, no conflicts.""" if state[-1] == -1: return False return not any(self.conflicted(state, state[col], col) for col in range(len(state))) def h(self, node): """Return number of conflicting queens for a given node""" num_conflicts = 0 for (r1, c1) in enumerate(node.state): for (r2, c2) in enumerate(node.state): if (r1, c1) != (r2, c2): num_conflicts += self.conflict(r1, c1, r2, c2) return num_conflicts
I havve changed def __init__
to
def __init__(self, N, K): super().__init__(tuple([-1] * N)) self.N = N self.K = K
so it can now except two parameters
Step by Step Solution
There are 3 Steps involved in it
Step: 1
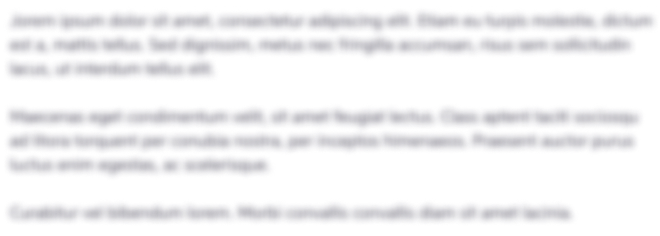
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started