Python - I am not sure if I am doing this right. I am trying to build a Food Factory Base Class. See my script below and the screen shot of the output. I can't see what I am doing wrong. I have yellow highlighted the areas in the assignment that I am confused about. Any help is truly appreciated.
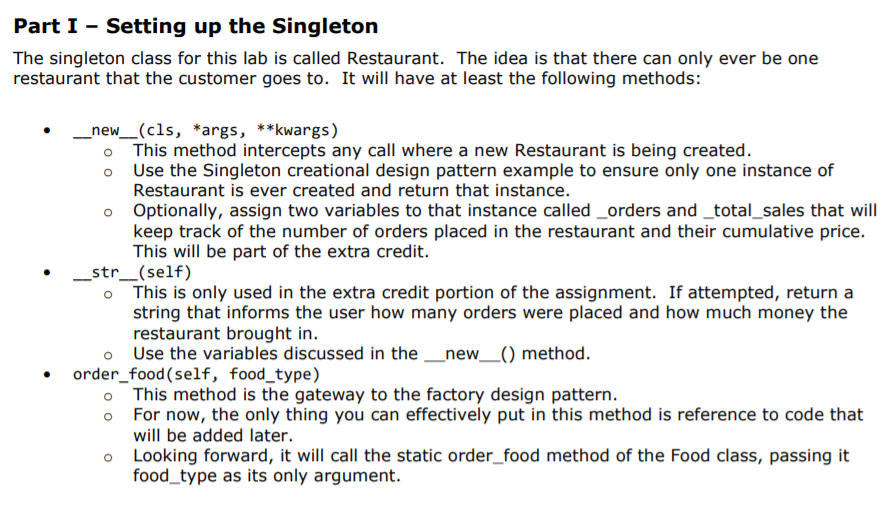

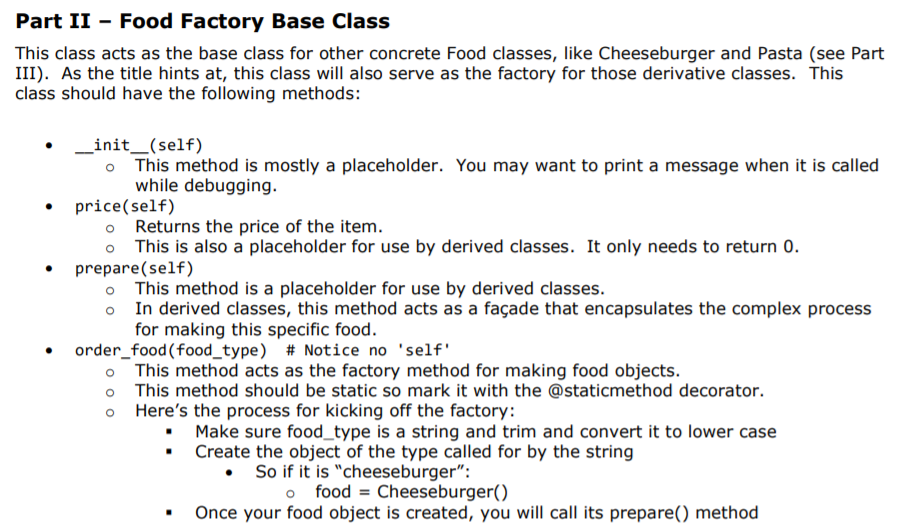
SCRIPT:
#stub function to order food def order_food_stub(food_type): return 50
#restaurant class class Restaurant(object): #instance object for singleton _instance = None #new method for making call singleton and initiliazing class vars def __new__(cls, *args, **kwargs): #making singleton if cls._instance is None: cls._instance = super(Restaurant, cls).__new__(cls) #init class vars cls._orders = 0 cls._total_sales = 0 return cls._instance #to string method def __str__(self): msg = str(self._orders)+" total orders that amount to $"+str(self._total_sales) return msg
#order method def order_food(self, food_type): #increment count and price self._orders += 1 #one way of calling the method #Food().order_food(food_type) self._total_sales += order_food_stub(food_type) #functionality test res = Restaurant() res.order_food("sushi") print(res)
#singleton test res2 = Restaurant() res2.order_food("fries") print(res2)
# class Cheeseburger class Cheeseburger: def __init__(self): pass
def prepare(self): print("preparing CheeseBurger")
class Food: # updated method @staticmethod def order_food(food_type): if type(food_type) == str: food_type = food_type.lower() if food_type == "cheeseburger": food = Cheeseburger() food.prepare() print(food_type)
def __init__(self): print("it is debugging.")
def price(self): # place holder for derived classes return 0
def prepare(self): # place holder for derived classes pass
# tested code rs = Restaurant() rs.order_food('cheeseburger')
OUTPUT:

Part I - Setting up the Singleton The singleton class for this lab is called Restaurant. The idea is that there can only ever be one restaurant that the customer goes to. It will have at least the following methods: _new__(cls, *args, **kwargs) This method intercepts any call where a new Restaurant is being created. Use the Singleton creational design pattern example to ensure only one instance of Restaurant is ever created and return that instance. Optionally, assign two variables to that instance called _orders and _total_sales that will keep track of the number of orders placed in the restaurant and their cumulative price. This will be part of the extra credit. __str__(self) This is only used in the extra credit portion of the assignment. If attempted, return a string that informs the user how many orders were placed and how much money the restaurant brought in. o Use the variables discussed in the __new__() method. order_food(self, food_type) o This method is the gateway to the factory design pattern. o For now, the only thing you can effectively put in this method is reference to code that will be added later. Looking forward, it will call the static order_food method of the Food class, passing it food_type as its only argument. O Additionally, this is where you will have to alter the _orders and _total_sales variables if you are going down that route. Part II - Food Factory Base Class This class acts as the base class for other concrete Food classes, like Cheeseburger and Pasta (see Part III). As the title hints at, this class will also serve as the factory for those derivative classes. This class should have the following methods: . _init__(self) o This method is mostly a placeholder. You may want to print a message when it is called while debugging. price(self) o Returns the price of the item. This is also a placeholder for use by derived classes. It only needs to return 0. prepare(self) This method is a placeholder for use by derived classes. In derived classes, this method acts as a faade that encapsulates the complex process for making this specific food. order_food(food_type) # Notice no 'self' o This method acts as the factory method for making food objects. o This method should be static so mark it with the @staticmethod decorator. o Here's the process for kicking off the factory: . Make sure food_type is a string and trim and convert it to lower case Create the object of the type called for by the string So if it is "cheeseburger": o food = Cheeseburger() . Once your food object is created, you will call its prepare() method 1 total orders that amount to $50 2 total orders that amount to $100