Question
Python - I need help with building my customer object inside my main section. I am stuck with this function. You can see my attempts
Python - I need help with building my customer object inside my main section. I am stuck with this function. You can see my attempts below. I don't understand how to use the max_customers through loops or a comprehension in my function when I am already calling it in the beginning of script. The same goes with the customer's __int__(), I am already calling it in the beginning. I have no idea to create the sleep for 5 seconds so that the teller can go back to work.
Script:
from threading import Semaphore, Thread, Lock from queue import Queue, Empty from random import randint from time import sleep
max_customers_in_bank = 10 # maximum number of customers that can be in the bank at one time max_customers = 30 # number of customers that will go to the bank today max_tellers = 3 # number of tellers working today teller_timeout = 10 # longest time that a teller will wait for new customers
class Customer(): def __init__(self, name): self.name = name
def __str__(self): return f"'{self.name}'"
class Teller(): def __init__(self, name): self.name = name
def __str__(self): return f"'{self.name}'"
def bank_print(lock, msg): with lock: print(msg)
def wait_outside_bank(customer, guard, teller_line, printlock): bank_print(printlock, f"(C) {customer} waiting outside bank") guard.acquire() bank_print(printlock, f"
def teller_job(teller, guard, teller_line, printlock): bank_print(printlock, f"[T] {teller} starting work") while True: try: c = teller_line.get(timeout=teller_timeout) bank_print(printlock, f"[T] {teller} is now helping {c}") sleep(randint(1,4)) bank_print(printlock, f"[T] {teller} done helping {c}") bank_print(printlock, f"
if __name__ == "__main__": printlock = Lock() teller_line = Queue(maxsize=max_customers_in_bank) guard = Semaphore(max_customers_in_bank) bank_print(printlock, "
customer_jobs = [] for i in range(1, max_customers+1): customer_name = 'Customer ' + str(i) customer = Customer(customer_name) c = Thread(target=wait_outside_bank, args=(customer, guard, teller_line, printlock)) c.start() customer_jobs.append(c) sleep(5)
bank_print(printlock, f"*B* Tellers starting work now") teller_jobs = [] for i in range(1, max_tellers+1): teller_name = 'Teller ' + str(i) teller = Teller(teller_name) t = Thread(target=teller_job, args=(teller, guard, teller_line, printlock)) t.start() teller_jobs.append(t) for cj in customer_jobs: cj.join() for tj in teller_jobs: tj.join() bank_print(printlock, f"*B* Bank closed") def wait_outside_bank(customer, guard, teller_line, printlock): target = wait_outside_bank args = (customer, guard, teller_line, printlock)
Part II Get Customers into the Bank Now, in the __main__section, you are going to create the Customer objects that want to go into the bank. This can be done several different ways, such as using a for loop or a comprehension. The key requirements are: You must create max_customers Customer objects Each Customer object must have a unique name (remember, that is the only argument to Customer's _init__() function) Now, each customer must be passed to a thread function, wait_outside_bank(), which we have to define now. We will get back to __main__ in a moment... def wait_outside_bank(customer, guard, teller_line, printlock): Where: customer is a Customer object guard is the security guard semaphore teller_line is the Queue printlock is the Lock The thread function must do the following: Print a customer message indicating that the customer is waiting outside the bank Attempt to acquire a semaphore from the guard object (do NOT use a context manager here since the semaphore is to be released in another method) Print a security guard message indicating they have let that customer into the bank Print a customer message indicating they are trying to get into line Put the customer into the teller_line queue (queue's put() method) Back in the __main__section, after the customer objects are created, spawn a Thread object for each one using the following criteria: target = wait_outside_bank args = (customer, guard, teller_line, printlock) Now, start each of those threads (you can do it in the same loop as the Thread creation). Finally, sleep for 5 seconds to give time for the tellers to get to work. 7 from threading import Semaphore, Thread, Lock from queue import Queue, Empty from random import randint from time import sleep max_customers_in_bank = 10 # maximum number of customers that can be in the bank at one time max_customers = 30 # number of customers that will go to the bank today max_tellers = 3 # number of tellers working today teller_timeout = 10 # longest time that a teller will wait for new customers class Customer(): def _init__(self, name): self.name = name def _str_(self): return f"'{self.name}'" class Teller(): def _init__(self, name): self.r .name = name 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 def _str_(self): return f"'{self.name} '" def bank_print(lock, msg): with lock: print(msg) def wait_outside_bank(customer, guard, teller_line, printlock): bank_print (printlock, f"(C) {customer} waiting outside bank") guard.acquire() bank_print (printlock, f"Step by Step Solution
There are 3 Steps involved in it
Step: 1
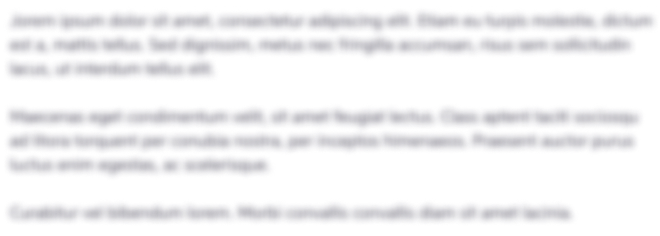
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started