Question
Python:( Need help in Exercise 2,3,4) Exercise 1: Prompt for Integer Function Create a function called PromptForInteger The function should return one value - a
Python:( Need help in Exercise 2,3,4)
Exercise 1: Prompt for Integer Function
- Create a function called PromptForInteger
- The function should return one value - a valid integer (between 1 and 100) that the user entered
- Inside the function, ask the user to enter an integer between 1 and 100 by displaying:
- "Please enter an integer (1 to 100): "
- Use the input() function here and provide the string as its parameter
- Read in the input and store it in a variable
- While the user enters invalid values (less than 1 or greater than 100)
- Print the error "Your response must be from 1 to 100, try again: "
- Use the input() function here and provide the error message string as its parameter
- Once the user enters a valid value, return that value
- Call the function in the area for Exercise 1 and print the value it returns
Exercise 2: Custom Range
- Modify your PromptForInteger to have four parameters:
- a minimum value for the integer
- a maximum value for the integer
- a string that represents the initial message printed
- a string that represents the error message printed if an invalid response is received
- Modify your loop in the function to use the minimum and maximum values
- Modify your prompts in the function to use the parameters
- Modify exercise 1 to use this new version of the function
- Call the function four times in the area for Exercise 2 and print the value it returns. Use the following min and max values and messages:
- -50, 50, "Please enter an integer (-50 to 50): ", "Your response must be from -50 to 50, try again: "
- 98, 106, "Please enter your temperature (98 to 106): ", "You must be dead, try again (98 to 106): "
- -25, -1, "Please enter the deduction from your account (-25 to -1): ", "Your response must be from -25 to -1, try again: "
- 1, 10, "Please enter a menu selection (1 to 10): ", "You must choose 1 to 10, try again: "
Exercise 3: Modifying the Exercise Loop
- Modify the while loop that loops through the exercises to use your PromptForInteger method
- Use the initial prompt and the appropriate error from the 'else' statement as arguments
- There is no need to modify the Exercise 3 area
- This will change the functionality of this system just a little, but the previous exercises should still work!
Exercise 4: Display List Elements on Demand and Copying Lists
- Create a list of names: "Stan", "Cartman", "Butters", "Kyle", "Kenny"
- Use enumerate() in a loop to display the index of each name followed by a space, followed by the name, on a line by itself, like so:
- "# name"
- replace # with the index and name with the actual name from the list
- ex:
- 0 Stan
- 1 Cartman
- 2 Butters
- Using PromptForInteger, ask the user for the index of an item in the list (hint: base the maximum on the size of the list. what would be the minimum?)
- message: "Choose a name from the list by number: "
- error: "You must choose one of the above numbers, try again: "
- Print the name the user selects
- "You chose name X" where X is replaced by the name
- Make a copy of the list of names
- Print the copy
- Remove Cartman from the original list
- Print the original list
- Print the copy
- Remove Kenny from the copy
- Print the original list
- Print the copy
My Code:
# Functions
def PromptForInteger(min,max): myInteger=int(input("Please enter an integer (1 to 100): ")) while not(myInteger>=min and myInteger<= max): myInteger=int(input("Your response must be from 1 to 100, try again: "))
return myInteger
def promptForInteger(min,max,init_msg,error_msg): number=int(input(init_msg)) while not (myInteger>=min and myInteger): number=int(input(error_msg)) return number
# Main # Exercise 1: Prompt for Integer # Now you can call function print(PromptForInteger(1,100))
# Exercise 2: Custom Range
# Function definition is here # Now you can call function print(promptForInteger(-50,50,"Please enter an integer (-50 to 50): ","Your response must be from -50 to 50, try again: "))
print(promptForInteger(98, 106, "Please enter your temperature (98 to 106): ", "You must be dead, try again (98 to 106): "))
print(promptForInteger(-25, -1, "Please enter the deduction from your account (-25 to -1): ", "Your response must be from -25 to -1, try again: "))
print(promptForInteger(1, 10, "Please enter a menu selection (1 to 10): ", "You must choose 1 to 10, try again: ")) # Exercise 3: Modifying the Exercise Loop # Exercise 4: Simple Lists
Step by Step Solution
There are 3 Steps involved in it
Step: 1
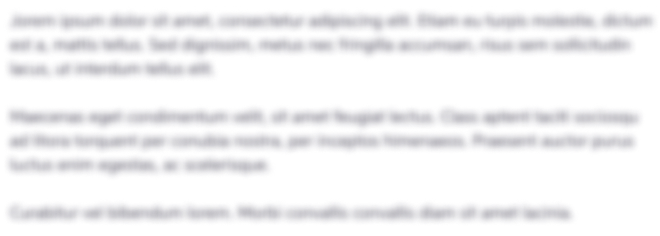
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started