Question
PYTHON PROGRAM: Part C For this program, you will be writing a series of functions that can be used as part of a secret message
PYTHON PROGRAM:
Part C
For this program, you will be writing a series of functions that can be used as part of a secret message encoder. Here are the functions you will be writing as well as some sample code to use to test your work.
Function to Add Letters
# function: add_letters # input: a word to scramble (String) and a number of letters (integer) # processing: adds a number of random letters (A-Z; a-z) after each letter # in the supplied word. for example, if word="cat" and num=1 # we could generate any of the following: # cZaQtR # cwaRts # cEaett # # if word="cat" and num=2 we could generate any of the following: # cRtaHFtui # cnnaNYtjn # czAaAitym # # output: returns the newly generated word def add_letters(word, num): # function code goes here!
Sample Program
# define original word original = "Hello!" # loop to demonstrate the function for num in range(1, 5): # scramble the word using 'num' extra characters scrambled = add_letters(original, num) # output print("Adding", num, "random characters to", original, ". . .", scrambled)
Sample Output
Adding 1 random characters to Hello! . . . HdeulHlHom!t Adding 2 random characters to Hello! . . . HTLedklFNljioMH!bi Adding 3 random characters to Hello! . . . HHHZeZrflqSflzOiosNU!jBk Adding 4 random characters to Hello! . . . HFtRKeivFllRNlUlGTaooYwoH!JpXL
Hint: You will need to use a loop to generate the required number of random characters and you will (obviously) need to use some random number functions as well. Think about the algorithm before you start coding! Draw out the steps you think you need to take on a piece of paper. For example: Start with the first character in the source word. Then generate num new random characters and concatenate these characters. Then move onto the next character in the source word and repeat the process.
Function to Remove Letters
Once you have written the add_letters function you should begin to work on the next function (remove_letters) which will perform the reverse operation.
# function: remove_letters # input: a word to unscramble (String) and the number of characters to remove (integer) # processing: the function starts at the first position in the supplied word and keeps it. # it then removes "num" characters from the word. the process is repeated again # if the word contains additional characters - the next character is kept # and "num" more characters are removed. For example, if word="cZaYtU" and # num=1 the function will generate the following: # # cat (keeping character 0, removing character 1, keeping character 2, removing # character 3, keeping character 4, removing character 5) # # output: returns the newly unscrambed word def remove_letters(word, num): # function code goes here!
Sample Program
word1 = "HdeulHlHom!t" word2 = "HTLedklFNljioMH!bi" word3 = "HHHZeZrflqSflzOiosNU!jBk" word4 = "HFtRKeivFllRNlUlGTaooYwoH!JpXL" unscrambled1 = remove_letters(word1, 1) print("Removing 1 characer from", word1, ". . .", unscrambled1) unscrambled2 = remove_letters(word2, 2) print("Removing 2 characers from", word2, ". . .", unscrambled2) unscrambled3 = remove_letters(word3, 3) print("Removing 3 characers from", word3, ". . .", unscrambled3) unscrambled4 = remove_letters(word4, 4) print("Removing 4 characers from", word4, ". . .", unscrambled4)
Sample Output
Removing 1 characer from HdeulHlHom!t . . . Hello! Removing 2 characers from HTLedklFNljioMH!bi . . . Hello! Removing 3 characers from HHHZeZrflqSflzOiosNU!jBk . . . Hello! Removing 4 characers from HFtRKeivFllRNlUlGTaooYwoH!JpXL . . . Hello!
Hint: String slicing may make your life a lot easier when writing this function!
Function to Shift Characters
Next, write a function called shift_characters that shifts the characters in a word up or down based on their ASCII table positionheres the IPO (input, processing, output) notation and a sample program:
# function: shift_characters # input: a word (String) and a number of characters to shift (integer) # processing: shifts each character in the supplied word to another position in the ASCII # table. the new position is dictated by the supplied integer. for example, # if word = "apple" and num=1 the newly generated word would be: # # bqqmf # # because we added +1 to each character. if we were to call the function with # word = "bqqmf" and num=-1 the newly generated word would be: # # apple # # because we added -1 to each character, which shifted each character down by # one position on the ASCII table. # # output: returns the newly generated word def shift_characters(word, num): # function code goes here!
Sample Program
word1 = "apple" newword1 = shift_characters(word1, 1) print(word1, "shifted by +1 is", newword1) unscrambled1 = shift_characters(newword1, -1) print(newword1, "shifted by -1 is", unscrambled1) word2 = "Pears are yummy!" newword2 = shift_characters(word2, 2) print(word2, "shifted by +2 is", newword2) unscrambled2 = shift_characters(newword2, -2) print(newword2, "shifted by -2 is", unscrambled2)
Sample Output
apple shifted by +1 is bqqmf bqqmf shifted by -1 is apple Pears are yummy! shifted by +2 is Rgctu"ctg"{woo{# Rgctu"ctg"{woo{# shifted by -2 is Pears are yummy!
Hint: use the ord() and chr() functions!
Encode/Decode
Finally, you are going to write an encoder/decoder program that makes use of your three cryptographic functions. Begin by writing a program that continually asks the user to enter in an optionthe user can either (e)ncode a word, (d)ecode a word or (q)uit the program.
If the user chooses to encode a word you should do the following:
Ask the user for a positive number between 1 and 5. Reprompt them if necessary.
Next, ask them to enter in a phrase that they want to encode.
Finally, apply the following algorithm to their word:
Add num random characters in between each letter of their word (using your add_letters) function
Shift the word num characters (using your shift_characters function)
If the user chooses to decode a word you should do the following:
Ask the user for a positive number between 1 and 5. Re-prompt them if necessary.
Next, ask them to enter in a phrase that they want to encode.
Finally, apply the following algorithm to their word:
Subtract num random characters in between each letter of their word (using your remove_letters) function
Shift the word DOWN by num characters (using your shift_characters function)
Heres a sample running of the program:
(e)ncode, (d)ecode or (q)uit: e Enter a number between 1 and 5: 1 Enter a phrase to encode: apple Your encoded word is: boqDqfmsfz (e)ncode, (d)ecode or (q)uit: d Enter a number between 1 and 5: 1 Enter a phrase to decode: boqDqfmsfz Your decoded word is: apple (e)ncode, (d)ecode or (q)uit: e Enter a number between 1 and 5: 2 Enter a phrase to encode: Hello, World!! :) Your encoded word is: JHmgn{nNYnukqFR.Fq"vEY\OqYFt\[n{lf|Y#mJ#kr"UT
Step by Step Solution
There are 3 Steps involved in it
Step: 1
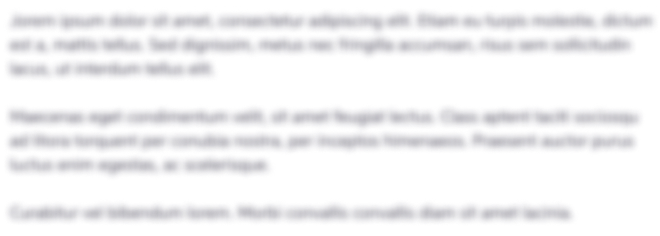
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started