Answered step by step
Verified Expert Solution
Question
1 Approved Answer
python programming design and implement a python script that operates a text file,reads all the lines in the file and display it to the console
python programming design and implement a python script that operates a text file,reads all the lines in the file and display it to the console as follows: -the lines should displays in reverse order,the first lines in the file is the last line displayed. -the word order in the line is reversed ,the first word in the line from the file is the last word displayed on a line in the console. - the characters in each word are not reversed the first letter of each word in the file appears as the first letter in the word displayed. for this purpose a 'word' is any text separated by one or more spaces for example, suppose i have text file name months.txt with three followings lines January February March April May June July August . September October November December --Running your script on the console should produce the following output: December November October September August . July June May April March February January --use Stack operations . TStack.py has the same Stack ADT interface, and has been thoroughly tested. If the script does not use the Stack ADT correctly, this implementation will cause a runtime error. script should not the reverse() method for lists, or anything similar for strings. script should not uses the extended slice syntax for lists or strings to reverse the data. script should use the Stack ADT in a way that demonstrates mastery of the stack concept # A stack (also called a pushdown or LIFO stack) is a compound # data structure in which the data values are ordered according # to the LIFO (last-in first-out) protocol. # # Implementation: # This implementation was designed to point out when ADT operations are # used incorrectly. # This implementation is not to be considered a good example of anything. # It simply prevents casual and mistaken violations of the ADT Principle. __stack_emsg = "If you're seeing this message, you gave the wrong 1st argument to Stack.{}" def create(): """ Purpose creates an empty stack Return an empty stack """ return '__Stack__',list() def is_empty(stack): """ Purpose checks if the given stack has no data in it Pre-conditions: stack is a stack created by create() Return: True if the stack has no data, or false otherwise """ if type(stack) is tuple: t,s = stack assert t == '__Stack__', __stack_emsg.format('is_empty()') return len(s) == 0 else: assert False, __stack_emsg.format('is_empty()') def size(stack): """ Purpose returns the number of data values in the given stack Pre-conditions: stack: a stack created by create() Return: The number of data values in the queue """ if type(stack) is tuple: t,s = stack assert t == '__Stack__', __stack_emsg.format('size()') return len(s) else: assert False, __stack_emsg.format('size()') def push(stack, value): """ Purpose adds the given data value to the given stack Pre-conditions: queue: a stack created by create() value: data to be added Post-condition: the value is added to the stack Return: (none) """ if type(stack) is tuple: t,s = stack assert t == '__Stack__', __stack_emsg.format('push()') s.append(value) else: assert False, __stack_emsg.format('push') def pop(stack): """ Purpose removes and returns a data value from the given stack Pre-conditions: stack: a stack created by create() Post-condition: the top value is removed from the stack Return: returns the value at the top of the stack """ if type(stack) is tuple: t,s = stack assert t == '__Stack__', __stack_emsg.format('pop()') return s.pop() else: assert False, __stack_emsg.format('pop()') def peek(stack): """ Purpose returns the value from the front of given stack without removing it Pre-conditions: stack: a stack created by create() Post-condition: None Return: the value at the front of the stack """ if type(stack) is tuple: t,s = stack assert t == '__Stack__', __stack_emsg.format('peek()') return s[-1] else: assert False, __stack_emsg.format('peek()')
# CMPT 145: Linear ADTs # Defines the Stack ADT # # A stack (also called a pushdown or LIFO stack) is a compound # data structure in which the data values are ordered according # to the LIFO (last-in first-out) protocol. # # Implementation: # This implementation was designed to point out when ADT operations are # used incorrectly. # This implementation is not to be considered a good example of anything. # It simply prevents casual and mistaken violations of the ADT Principle. __stack_emsg = "If you're seeing this message, you gave the wrong 1st argument to Stack.{}" def create(): """ Purpose creates an empty stack Return an empty stack """ return '__Stack__',list() def is_empty(stack): """ Purpose checks if the given stack has no data in it Pre-conditions: stack is a stack created by create() Return: True if the stack has no data, or false otherwise """ if type(stack) is tuple: t,s = stack assert t == '__Stack__', __stack_emsg.format('is_empty()') return len(s) == 0 else: assert False, __stack_emsg.format('is_empty()') def size(stack): """ Purpose returns the number of data values in the given stack Pre-conditions: stack: a stack created by create() Return: The number of data values in the queue """ if type(stack) is tuple: t,s = stack assert t == '__Stack__', __stack_emsg.format('size()') return len(s) else: assert False, __stack_emsg.format('size()') def push(stack, value): """ Purpose adds the given data value to the given stack Pre-conditions: queue: a stack created by create() value: data to be added Post-condition: the value is added to the stack Return: (none) """ if type(stack) is tuple: t,s = stack assert t == '__Stack__', __stack_emsg.format('push()') s.append(value) else: assert False, __stack_emsg.format('push') def pop(stack): """ Purpose removes and returns a data value from the given stack Pre-conditions: stack: a stack created by create() Post-condition: the top value is removed from the stack Return: returns the value at the top of the stack """ if type(stack) is tuple: t,s = stack assert t == '__Stack__', __stack_emsg.format('pop()') return s.pop() else: assert False, __stack_emsg.format('pop()') def peek(stack): """ Purpose returns the value from the front of given stack without removing it Pre-conditions: stack: a stack created by create() Post-condition: None Return: the value at the front of the stack """ if type(stack) is tuple: t,s = stack assert t == '__Stack__', __stack_emsg.format('peek()') return s[-1] else: assert False, __stack_emsg.format('peek()')
Step by Step Solution
There are 3 Steps involved in it
Step: 1
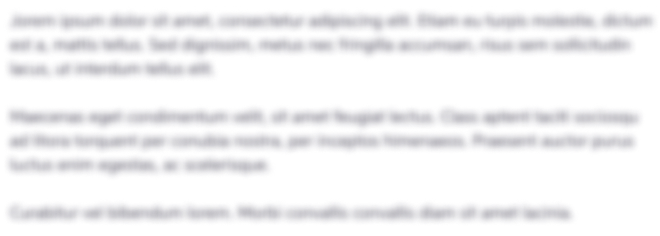
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started