Question
Python Programming. In this project you will read in poverty data collected by the 2010 U.S. census for each county in Michigan. We will do
Python Programming.
In this project you will read in poverty data collected by the 2010 U.S. census for each county in Michigan. We will do some statistics using this data.
The file named michigan_hard.txt contains the original data set from the census. (For the .txt file go to, http://www.census.gov//did/www/saipe/data/statecounty/data/2011.html . Go to the 2011 Michigan State and County estimates. Will have a part of the .txt below)
The web site describes the format of this text file, indicating the columns the data occurs in.
For the most part, we can ignore the column numbers and just work with the file as space-separated data.
However, the county name may have spaces in it so it is best to use a string slice.
The data we care about for each county are:
count of people age 0-17 (i.e., children) in poverty (index 8)
percentage of people age 0-17 (i.e., children) in poverty (index 11)
median household income (index 20)
county name (columns 193-238)
The program will input the data set and construct a dictionary of the needed data. If the dictionary of the needed data cannot be constructed, the program will display an appropriate error message and halt.
After constructing the dictionary of needed data, the program will display a menu of options and allow the user to search for counties which meet certain criteria. The menu options are:
1. Display the county with the highest percentage of children in poverty: The program will print out a summary of the county that includes the following:
County name
Percentage
Count of children in poverty
Median household income
2. Display the county with the lowest percentage of children in poverty: The program will print out a summary of the county that includes the following:
County name
Percentage
Count of children in poverty
Median household income
3. Search for a county by name: Prompt the user for a string, then display a summary of all counties whose name contains that string (regardless of case), which includes the following:
County name
Percentage
Count of children in poverty
Median household income
4. Quit: If the user types either Q or q your program must stop executing
Program specifications
Required functions:
1. Each print specified above will print the same data so create a print_data function.
A. This function will be used by the next functions.
2. Create a function for the highest percentage data: find_highest_data
3. Create a function for the lowest percentage data: find_lowest_data
4. Create a function to search for the county data: find_county_data
Assignment Deliverables
The deliverable for this assignment is the following file:
Lastname_Firstname_10.py the source code for your Python program
Be sure to use the specified file name
Assignment Notes
1. Your program will consist of at least four functions: a separate function to process each of the three menu options listed above and a print function to print the summary of county data.
2. You must use dictionaries somewhere in you program. I dont care what other types you use, but dictionaries are a must.
3. Be sure to display the county information as displayed below
4. Your program will continue to execute until the user selects Q (or q) as the menu option.
5. Be sure to prompt the user for the inputs in the specified order. Also, your program cannot prompt the user for any other inputs.
6. Your program will handle erroneous user inputs. If there are any problems with a particular user input, your program will display the menu and allow the user to select another option.
*Please help. Thank you for your time and effort.*
.txt file (The data comes from the U.S. census of 2010 and has been updated for 2011. It is located at http://www.census.gov//did/www/saipe/data/statecounty/data/2011.html and is available in a number of formats)
26 1 1914 1547 2281 17.9 14.5 21.3 493 383 603 32.6 25.3 39.9 331 251 411 28.2 21.4 35.0 35407 32448 38366 Alcona County MI est11_MI.txt 28NOV2012
26 3 1388 1092 1684 16.3 12.8 19.8 355 275 435 22.7 17.6 27.8 233 175 291 19.2 14.4 24.0 39486 36463 42509 Alger County MI est11_MI.txt 28NOV2012
26 5 13945 11772 16118 12.7 10.7 14.7 5150 4202 6098 18.3 14.9 21.7 3353 2628 4078 16.0 12.5 19.5 50559 48296 52822 Allegan County MI est11_MI.txt 28NOV2012
26 7 5389 4615 6163 18.6 15.9 21.3 1597 1276 1918 27.4 21.9 32.9 1075 834 1316 24.4 18.9 29.9 37270 34747 39793 Alpena County MI est11_MI.txt 28NOV2012
26 9 3830 3242 4418 16.6 14.1 19.1 1376 1144 1608 30.0 24.9 35.1 917 748 1086 26.4 21.5 31.3 41679 38551 44807 Antrim County MI est11_MI.txt 28NOV2012
26 11 2887 2390 3384 18.7 15.5 21.9 952 765 1139 31.9 25.6 38.2 683 547 819 29.8 23.9 35.7 38008 35442 40574 Arenac County MI est11_MI.txt 28NOV2012
26 13 1339 1053 1625 17.2 13.5 20.9 421 329 513 24.9 19.4 30.4 280 211 349 21.5 16.2 26.8 38119 34419 41819 Baraga County MI est11_MI.txt 28NOV2012
26 15 6579 5352 7806 11.3 9.2 13.4 2251 1770 2732 16.3 12.8 19.8 1504 1153 1855 14.5 11.1 17.9 50051 46813 53289 Barry County MI est11_MI.txt 28NOV2012
Sample output
What would you like to do?
1: Display County with the Highest Poverty rate
2: Display County with the Lowest Poverty rate
3: Search for County by name
Q: Quit
>1
County Name: xxxxxx
Percentage of children in poverty: xx.xx%
Count of children in poverty: xxxx
Median Household income: xxx,xxx
What would you like to do?
1: Display County with the Highest Poverty rate
2: Display County with the Lowest Poverty rate
3: Search for County by name
Q: Quit
>2
County Name: xxxxxx
Percentage of children in poverty: xx.xx%
Count of children in poverty: xxxx
Median Household income: xxx,xxx
What would you like to do?
1: Display County with the Highest Poverty rate
2: Display County with the Lowest Poverty rate
3: Search for County by name
Q: Quit
>3
Enter a County name (or part of a name)
>zi
County Name: Benzie County
Percentage of children in poverty: 20.6%
Count of children in poverty: 710
Median Household income: 45,998
Step by Step Solution
There are 3 Steps involved in it
Step: 1
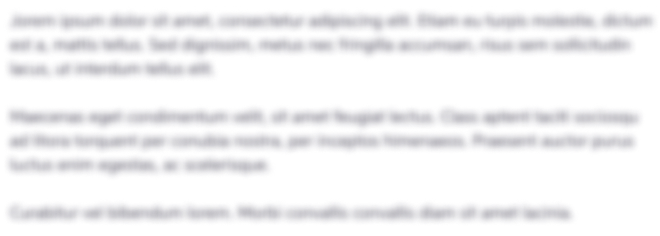
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started