Question
Python programming question. #### I have developed the following code, however, I do not know how I am supposed to decode the program to match
Python programming question.
#### I have developed the following code, however, I do not know how I am supposed to decode the program to match the desired output.
< symbolize what I have
> symbolizes what I need
http://prntscr.com/hkhyba
To decode the function look at the differences in the tests run in the screenshot above, for example: In the bottom test case E=A, P=R, and H=E, and so on.
I was also given very strict instructions to not modify the main function provided.
# HERE ARE THE INSTRUCTIONS I WAS GIVEN
You will write a program to encrypt and decrypt messages based on a "code book." The "code book" will be a very long string read in from the user; you will process this book by calculating how many times each letter (A-Z) appears. Then you will sort the list with the highest frequency letters first, down to the lowest frequency letters. If two letters have the same frequency, they are listed in alphabetical order. Ignore upper/lower case when processing letters. Once you have the sorted list, assign the code as follows: Most common letter in the "code book" is an "A"; the second most common letter in the "code book" is "B", etc. The least common letter in the code will be assigned the letter "Z". Once you have the code, you will encode and decode messages using the code. You will also implement two commands: SKIP and EXIT. SKIP means that you will SKIP an entry, and EXIT means that you will stop asking and end the program. For example:
Please enter the codebook: pooiiiuuuuyyyyyttttttrrrrrrreeeeeeeewwwwwwwwwqqqqqqqqqq Sorted frequency of letters in code text: ('Q', 10) ('W', 9) ('E', 8) ('R', 7) ('T', 6) ('Y', 5) ('U', 4) ('I', 3) ('O', 2) ('P', 1) ('A', 0) ('B', 0) ('C', 0) ('D', 0) ('F', 0) ('G', 0) ('H', 0) ('J', 0) ('K', 0) ('L', 0) ('M', 0) ('N', 0) ('S', 0) ('V', 0) ('X', 0) ('Z', 0) Encode: ['Q', 'W', 'E', 'R', 'T', 'Y', 'U', 'I', 'O', 'P', 'A', 'B', 'C', 'D', 'F', 'G', 'H', 'J', 'K', 'L', 'M', 'N', 'S', 'V', 'X', 'Z'] Decode: ['K', 'L', 'M', 'N', 'C', 'O', 'P', 'Q', 'H', 'R', 'S', 'T', 'U', 'V', 'I', 'J', 'A', 'D', 'W', 'E', 'G', 'X', 'B', 'Y', 'F', 'Z'] Please enter a message to encode: This is a test. LIOK OK Q LTKL. Please enter a message to decode: LIOK OK Q LTKL. THIS IS A TEST. Please enter a message to encode: SKIP Please enter a message to decode: EXIT This program will self destruct in 10 seconds...
### THIS IS THE START OF THE CODE I DEVELOPED
def analyze(codebook):
codebook = codebook.upper()
string = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
codes = {}
for c in codebook:
if c in string:
if c in codes:
codes[c] += 1
else:
codes[c] = 1
freq = []
for c in codes:
freq.append((codes[c], c))
freq.sort(key=lambda x: x[1])
freq.sort(key=lambda x: x[0], reverse=True)
keys = codes.keys()
for c in string:
if not c in keys:
freq.append((0, c))
result = []
for item in freq:
result.append((item[1], item[0]))
return result
def printFreq(freq):
print("Sorted frequency of letters in code text:")
for i in range(len(freq)):
print(freq[i], end = " ")
if (i+1) % 8 == 0:
print("")
print("")
def assign(freq):
encode = []
string = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
for item in freq:
encode.append(item[0])
return (encode, list(string))
def codeme(encode, enc):
result = ""
enc = enc.upper()
string = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
for c in enc:
if c in string:
result += encode[ord(c) - ord('A')]
else:
result += c
return result
def decodeme(decode, dec):
result = ""
dec = dec.upper()
string = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
for c in dec:
if c in string:
result += decode[ord(c) - ord('A')]
else:
result += c
return result
# PLACE YOUR CODE UP HERE
# DO NOT MODIFY ANYTHING BELOW HERE OR YOU WILL GET A ZERO FOR THIS PROJECT
def main():
# read the code from input
codebook = input("Please enter the codebook: ")
print(codebook)
freq = analyze(codebook)
printFreq(freq)
encode, decode = assign(freq)
print("Encode:")
print(encode)
print("Decode:")
print(decode)
while (True):
enc = input("Please enter a message to encode: ")
print(enc)
if enc == "EXIT":
break
if enc != "SKIP":
coded = codeme(encode, enc)
print(coded)
dec = input("Please enter a message to decode: ")
print(dec)
if dec == "EXIT":
break
if dec != "SKIP":
coded = decodeme(decode, dec)
print(coded)
print("This program will self destruct in 10 seconds...")
main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
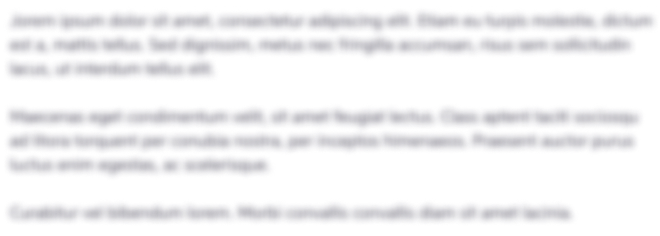
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started