Question
Q) Using Queue algorithm as shown in the code below, create a main class that to create a ticketing system for Customer Service counters. Assuming
Q) Using Queue algorithm as shown in the code below, create a main class that to create a ticketing system for Customer Service counters. Assuming there are 3 counters that need to serve customer of 3 different types of concerns. These are Technical Issue, Billing and New Customer.
Create a ticketing system that will help the Customer Service department in assisting their customer.
Note: The program should have a menu as shown below and include all necessary error checking for this program.
Menu
[1] Choose Services
[a] New Customer [b] Billing
[c] Technical Issue
[2] Task Accomplished
[a] New Customer
[b] Billing
[c] Technical Issue
[3] Display Number of Customers
[a] New Customer [b] Billing
[c] Technical Issue
[4] Exit
Enter your choice:
--------------------------------------------------------------Very important: Please use eclipse and do not add any method to the code below ( do not create display method use just while on the main class that you will create)
class Queue {
private int maxSize;
private long[] queArray;
private int front;
private int rear;
private int nItems;
public Queue(int s)
{
maxSize = s;
queArray = new long[maxSize]; front = 0;
rear = -1;
nItems = 0;
}
public void insert(long j)
{
if(rear == maxSize-1)
rear = -1;
queArray[++rear] = j;
nItems++;
}
public long remove()
{
long temp = queArray[front++];
if(front == maxSize)
front = 0;
nItems--;
return temp;
}
public long peekFront()
{
return queArray[front]; }
public boolean isEmpty()
{
return (nItems==0); }
public boolean isFull()
{
return (nItems==maxSize); }
public int size()
{
return nItems; }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
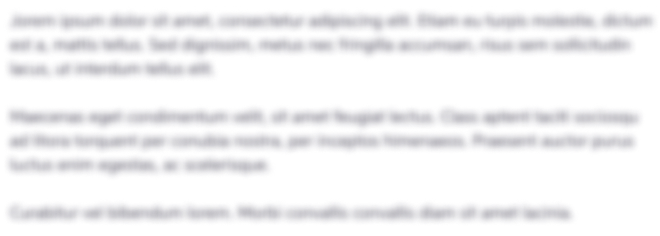
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started