Question
Q5 Analyze step by step the time complexity(BigOh) of your implementations on Q1 on the two methods containsAll( ), addAll(): You can work on this
Q5 Analyze step by step the time complexity(BigOh) of your implementations on Q1 on the two methods containsAll( ), addAll():
You can work on this question on paper and take a clear picture of your solution, and submit your work as a file via the insert tab on menu bar.
Implementation
import java.util.Collection;
public interface MyList
/**
* Add a new element at the specified index in this list
*/
public void add(int index, E e);
/**
* Return the element from this list at the specified index
*/
public E get(int index);
/**
* Return the index of the first matching element in this list.
*
* Return -1 if no match.
*/
public int indexOf(Object e);
/**
* Return the index of the last matching element in this list
*
* Return -1 if no match.
*/
public int lastIndexOf(E e);
/**
* Remove the element at the specified position in this list
*
* Shift any subsequent elements to the left.
*
* Return the element that was removed from the list.
*/
public E remove(int index);
/**
* Replace the element at the specified position in this list
*
* with the specified element and returns the new set.
*/
public E set(int index, E e);
@Override
/**
* Add a new element at the end of this list
*/
public default boolean add(E e) {
add(size(), e);
return true;
}
@Override
/**
* Return true if this list contains no elements
*/
public default boolean isEmpty() {
return size() == 0;
}
@Override
/**
* Remove the first occurrence of the element e
*
* from this list. Shift any subsequent elements to the left.
*
* Return true if the element is removed.
*/
public default boolean remove(Object e) {
if (indexOf(e) >= 0) {
remove(indexOf(e));
return true;
} else {
return false;
}
}
@Override
public default boolean containsAll(Collection> c) {
//looping through each item in c
for (Object e : c) {
//if this list does not contain element e, returning false
if (!this.contains(e)) {
return false;
}
}
//all elements on c is present in this list
return true;
}
/**
* Adds the elements in otherList to this list.
*
* Returns true if this list changed as a result of the call
*/
@Override
public default boolean addAll(Collection extends E> otherList) {
//initially list isnt changed
boolean changed = false;
//looping through each element in other list
for (E element : otherList) {
//adding element to this list, if addition returns true, then list
//is changed
if (this.add(element)) {
changed = true;
}
}
return changed;
}
@Override
public default boolean removeAll(Collection> c) {
boolean changed = false;
for (Object ob : c) {
//removing current element in c from this set
if (this.remove(ob)) {
//removal success, list is modified.
changed = true;
}
}
return changed;
}
/**
* Retains the elements in this list that are also in otherList
*
* Returns true if this list changed as a result of the call
*/
@Override
public default boolean retainAll(Collection> c) {
boolean changed = false;
int index = size() - 1;
//looping from last index to first
while (index >= 0) {
//getting element
Object ob = get(index);
//if c contains this element, removing it
if (c.contains(ob)) {
remove(index);
//list changed.
changed = true;
}
//moving to previous index
index--;
}
return changed;
}
@Override
public default Object[] toArray() {
//creating an Object array
Object array[] = new Object[size()];
//copying elements
for (int i = 0; i < size(); i++) {
array[i] = get(i);
}
//returning it
return array;
}
@Override
public default
//if array is not big enough to hold all elements, reinitializing array
if (array.length < size()) {
array = (T[]) new Object[size()];
}
//copying all elements to array
for (int i = 0; i < size(); i++) {
array[i] = (T) get(i);
}
//returning
return array;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
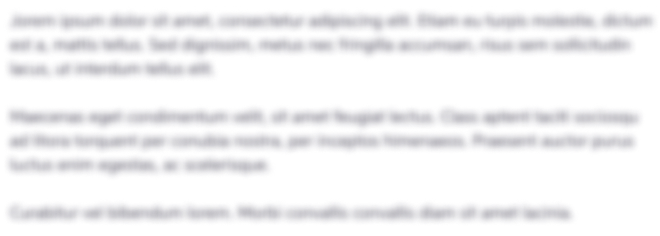
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started