Question
Question 1). What does the following method do on a chain of nodes? Assume that curr is a reference to the first node containing data
Question 1). What does the following method do on a chain of nodes? Assume that curr is a reference to the first node containing data and Listnode is a singly-linked Listnode as described in lecture and readings.
public static boolean methodA(Listnode
if (curr == null) return true;
if (curr.getNext() == null) return true;
return curr.getData() > curr.getNext().getData() && methodA(curr.getNext());
}
What the code return?
A). Returns true iff if the integers are in ascending order. Given the code, an empty chain is considered in ascending order by definition.
B). Returns true iff the Integers in the chain of Listnodes is strictly (no duplicates allowed) in descending order. Given the code, an empty chain is considered in descending order by definition.
C). Returns true iff if the integers are NOT in ascending order. Given the code, an empty chain is considered NOT in ascending order by definition.
D). Returns true iff if the integers are NOT in descending order. Given the code, an empty chain is considered NOT in descending order by definition.
Question 2). What does the following method do on a chain of nodes? Assume that curr is a reference to the first node containing data and Listnode is a singly-linked Listnode as described in lecture and readings.
public static
if (curr == null) return 0;
if (x.equals(curr.getData())) return 1 + methodB(curr.getNext(), x);
return methodB(curr.getNext(), x);
}
A). Returns the number of Listnodes containing items that are NOT equal to item x.
B). Returns the number of Listnodes containing items that equal item x.
C). Returns the one more than the number of Listnodes containing items that equal item x.
D). Returns the number of Listnodes containing items that equal item x+1.
Question 3). What does the call to methodC do on a chain of nodes? Assume that head is a reference to the first node containing data and Listnode is a singly-linked Listnode as described in lecture and readings.
head = methodC(head, x); //initial methodC call
public static Listnode
if (curr == null) return null;
if (curr.getData() == x)
return methodC(curr.getNext(), x);
curr.setNext(methodC(curr.getNext(), x));
return curr;
}
A). Modifies the chain of Listnodes so that all Listnodes containing the exact same Integer object as x (at same address) are removed.
B). Modifies the chain of Listnodes so that all Listnodes AFTER a Listnode containing the exact same Integer object as x (at same address) are removed.
C). Modifies the chain of Listnodes so that all Listnodes that do NOT contain the exact same Integer object as x (at same address) are removed.
D). Modifies the chain of Listnodes so that all Listnodes BEFORE a Listnode containing the exact same Integer object as x (at same address) are removed.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
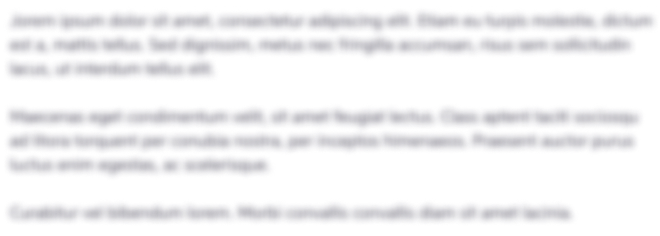
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started