Question
Question 1: Write a function mul_bin(b1, b2) that takes as inputs two strings b1 and b2 that represent binary numbers . The function should multiply
Question 1:
Write a function mul_bin(b1, b2) that takes as inputs two strings b1 and b2 that represent binary numbers. The function should multiply the numbers and return the result in the form of a string that represents a binary number. For example:
>>> mul_bin('11', '10') # 3 * 2 = 6 '110' >>> mul_bin('1001', '101') # 9 * 5 = 45 '101101'
In this function, you should not use recursion or perform any binary arithmetic. Rather, you should make use of the conversion functions that you wrote for problem 1. If you included the line mentioned above to import your code from pr4pr1.py, you should be able to call your dec_to_bin and bin_to_decfunctions from within this function. Convert both b1 and b2 to decimal, multiply the resulting decimal numbers, and then convert the resulting product back to binary! Here is the pseudocode:
n1 = the decimal value of the input string b1 (use one of your conversion functions!) n2 = the decimal value of the input string b2 (use that function again!) b = the binary value of (n1 * n2) (use your other function!) return b
Quesion 2:
Write a function add_bytes(b1, b2) that takes as inputs two strings b1 and b2 that represent bytes (i.e., 8-bit binary numbers). The function should compute the sum of the two bytes and return that sum in the form of a string that represents an 8-bit binary number. For example:
>>> add_bytes('00000011', '00000001') '00000100' >>> add_bytes('00011100', '00011110') '00111010'
Note that you will need to ensure that the result is exactly 8 bits long. If the result is too short, you should add 0s on the left-hand side to get a string that is 8 characters long. If the result is too big to be represented in 8 bits, you should return the rightmost 8 bits of the result. For example:
>>> add_bytes('10000011', '11000001') # the result (101000100) has 9 bits, so return the rightmost 8 bits '01000100'
Here again, you should not use recursion or perform any binary arithmetic. Rather, you should make use of the conversion functions that you wrote for problem 1.
Hint: To ensure that your result has the correct length, we encourage you to do the following:
Use your conversion functions to determine the binary representation of the sum, and store that result in a variable.
Determine the length of the result using the len() function, and store that length in a variable. Then you can use conditional execution (if-else or if-elif-else) to decide what you should return.
If the result is less than 8 bits long, precede the result with the correct number of leading '0's. You can use the multiplication operator to produce the necessary string of '0's.
Make sure that you also handle the case in which the result is more than 8 bits long.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
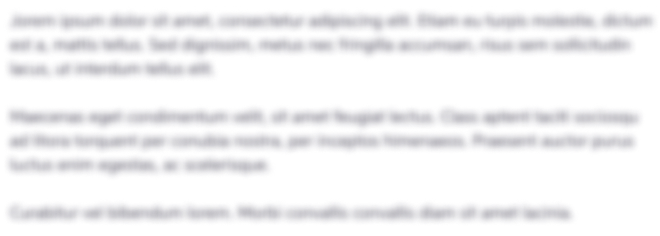
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started