Question
Question 3) Write a method for Tester that would return true if the ClockDisplay code works correctly when the time is set to 10:50 initially
Question 3) Write a method for Tester that would return true if the ClockDisplay code works correctly when the time is set to 10:50 initially and then the display is changed to a 12 hour display and then timeTick is called. The method should check the output from getTime() to see whether the result is correct.
public boolean testClock(){
...
This is the class, ClockDisplay that Question 3 refers to.
public class ClockDisplay { private NumberDisplay hours; private NumberDisplay minutes; private String displayString; // simulates the actual display private boolean twelveHourDisplay; /** * Constructor for ClockDisplay objects. This constructor * creates a new clock set at 00:00. */ public ClockDisplay() { hours = new NumberDisplay(24); minutes = new NumberDisplay(60); updateDisplay(); twelveHourDisplay=false; }
/** * Constructor for ClockDisplay objects. This constructor * creates a new clock set at the time specified by the * parameters. */ public ClockDisplay(int hour, int minute) { hours = new NumberDisplay(24); minutes = new NumberDisplay(60); setTime(hour, minute); twelveHourDisplay=false; } /** * This method should get called once every minute - it makes * the clock display go one minute forward. */ public void timeTick() { minutes.increment(); if(minutes.getValue() == 0) { // it just rolled over! hours.increment(); } updateDisplay(); }
/** * Set the time of the display to the specified hour and * minute. */ public void setTime(int hour, int minute) { hours.setValue(hour); minutes.setValue(minute); updateDisplay(); } /** * Return the current time of this display in the format HH:MM. */ public String getTime() { return displayString; } public void toggleDisplay() { if(twelveHourDisplay) { twelveHourDisplay=false; } else { twelveHourDisplay=true; } updateDisplay(); } /** * Update the internal string that represents the display. */ private void updateDisplay() { int h=Integer.parseInt(hours.getDisplayValue()); if(twelveHourDisplay) { if(h>12) { h=h-12; displayString = h + ":" + minutes.getDisplayValue()+":"+"PM"; } else displayString = h + ":" + minutes.getDisplayValue()+":"+"AM"; } else displayString = hours.getDisplayValue() + ":" + minutes.getDisplayValue(); } }
NumberDisplay.java
public class NumberDisplay { private int limit; private int value; /** * Constructor for objects of class NumberDisplay */ public NumberDisplay(int rollOverLimit) { limit = rollOverLimit; value = 0; }
public int getValue() { return value; } /** * Set the value of the display to the new specified * value. If the new value is less than zero or over the * limit, do nothing. */ public void setValue(int replacementValue) { if((replacementValue >= 0) && (replacementValue < limit)) { value = replacementValue; } } /** * Return the display value (that is, the current value * as a two-digit String. If the value is less than ten, * it will be padded with a leading zero). */ public String getDisplayValue() { if(value < 10) { return "0" + value; } else { return "" + value; } } /** * Increment the display value by one, rolling over to zero if * the limit is reached. */ public void increment() { value = (value + 1) % limit; } }
Test.java that contains main method
public class Test { public static void main(String[] args) { ClockDisplay clock = new ClockDisplay(); clock.setTime(21,01); System.out.print(" In 24 hour format:"); System.out.println(clock.getTime()); clock.toggleDisplay(); System.out.print(" In 12 hour format:"); System.out.println(clock.getTime()); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
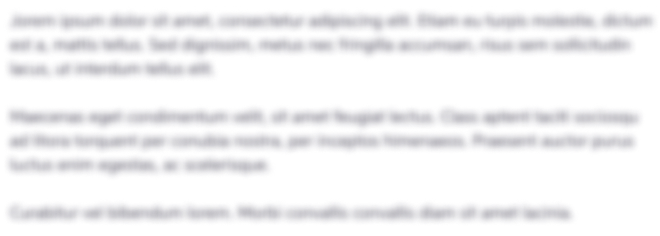
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started