Question
Question: Class and Constructor Creation Book Class Create a script called library.js. In this file create a constructor function for a Book object. The Book
Question:
Class and Constructor Creation Book Class Create a script called library.js. In this file create a constructor function for a Book object. The Book object should have the following properties: Title Available: Boolean representing whether the book is checked out or not. The initial value should be false. Publication Date: Use a date object Checkout Date: Use a date object Call Number: Make one up Authors: Should be an array of Author objects Author Class Create a constructor function for an object called Author. It should have a property for the first name and last name of the author. Patron Class Create a constructor function for an object called Patron. This represents a person who is allowed to check out books from the library. Give it the following properties: Firstname Lastname Library Card Number (Make one up) Books Out: Make it an array fine: Starts a 0.00 B. Methods to add Book Class Add a function to the Book prototype called "checkOut". The function will change the available property of the book from true to false and set the checkout date. The checkout date should be set to the current date minus some random number of days. Add a function to the Book prototype called "checkIn". The function will change the available property of the book from false to true. Add a function called isOverdue that checks the current date and the checked out date and if it's greater than 14 days it returns true Patron Class Add a function to the Patron prototype called "read" that adds a book to it's books out property. Add a function to the Patron prototype called "return" that removes a book from it's books out property. C. Test Program Create 5 different books from the Book Class and store them in an array called catalog. Create 5 different patrons from the Patron Class and store them in an array called patrons. Write a loop that simulates checkouts and checkins for a 3 month period. Every day iterate over the catalog, and every person in the patrons array. If the patron currently has the book checked out then check it in. If it is not checked out then add it to the patrons list of books via the patrons read method. If the book is overdue then add a fine of $5.00 to the patron returning it. At the end of the 3 month period, display each patron, the books they have currently checked out and any fine they may have.
I submit this:
//Author class var Author = function(firstName, lastName) { this.firstName = firstName; this.lastName = lastName; };
var Book = function(title, Available, publicationDate, checkoutDate, callNumber, Authors) { this.title = title; this.Available = Available; this.publicationDate = publicationDate; this.checkoutDate = checkoutDate; this.callNumber = callNumber; this.Authors = Authors; };
Book.prototype.checkOut = function(){ this.Available = false; var temp = new Date(1000000000); var d = new Date()-temp; var res = new Date(d); this.checkoutDate = res; };
Book.prototype.isOverdue = function(){ //Get 1 day in milliseconds var singleDay=1000*60*60*24;
var todayDate = new Date().getTime(); var difference = todayDate - this.checkoutDate.getTime(); if(Math.round(difference/singleDay) >= 14){ return true; } return false; };
var Patron = function(firstName, lastName, libraryCardNumber, booksOut, fine) { this.firstName = firstName; this.lastName = lastName; this.libraryCardNumber = libraryCardNumber; this.booksOut = booksOut; this.fine = fine; };
Patron.prototype.read = function(book){ this.booksOut.add(book); }
Patron.prototype.read = function(book){ this.booksOut.remove(this.booksOut.length); }
//creating author objects var authors = [] authors[0] = new Author("Neil","Armstrong"); authors[1] = new Author("Hard","Popeye");
Var mybooks = [] mybooks[0] = new Book('gravity',true,new Date(2000,5,20), new Date(), 10,authors); mybooks[1] = new Book('munro',true,new Date(2000,5,20), new Date(), 11,authors); mybooks[2] = new Book('kohli',true,new Date(2000,5,20), new Date(), 12,authors); mybooks[3] = new Book('sachin',true,new Date(2000,5,20), new Date(), 13,authors); mybooks[4] = new Book('sehwag',true,new Date(2000,5,20), new Date(), 14,authors);
var patrons = [] patrons[0] = new Patron('master','jumbo',1,mybooks,0.00); patrons[1] = new Patron('kyle','munro',1,mybooks,0.00); patrons[2] = new Patron('master','jumbo2',1,mybooks,0.00); patrons[3] = new Patron('master','jumbo3',1,mybooks,0.00); patrons[4] = new Patron('master','jumbo4',1,mybooks,0.00);
var j=0; while(j < patrons.length){ var books = patrons[j].booksOut; var fine = patrons[j].fine; for(var i=0;i if(books[i].isOverdue()){ fine = fine + 5.00; } } patrons[j].fine = fine; }
and the professor said:
-10 Syntax error- var should be lowercase
C:\Users\C0rbin\Documents\SPC Classes\2017_02(spring_b)\COP2801\Assignment4\Venuto_Assig4_Attempt1\Venuto_Assig4_Attempt1\library.js:64
Var mybooks = []
^^^^^^^
Once thats fixed there is no output.
-25 missing simulation
Here is a structure you should maybe use
//while loop or for loop for 90 days
//For loop over catalog
//forloop over patrons
//Check if available , if so check book out(Here would be a good place to made checkout date random 2-25 days in the past to force some late books when checking back in)
//If not available check book back in
//check checking back in check to see if book is overdue and if so add a fine
//When down loop over patrons to see their fees
Step by Step Solution
There are 3 Steps involved in it
Step: 1
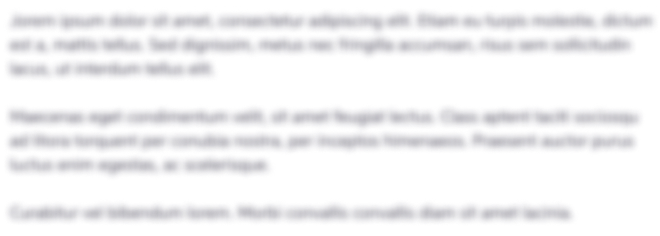
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started