Question
question Make a Fraction class with two int members (numerator and denominator). Create the following member functions: Create an Input function to allow the user
question
Make a Fraction class with two int members (numerator and denominator). Create the following member functions:
- Create an Input function to allow the user to enter the numerator and denominator. Make sure to have exception or if condition to deal with a denominator of 0 (zero).
- Create a Print function which will print out the Fraction in the form numerator/denominator i.e. 2/3
- Empty constructor which initializes the Fraction zero
- A one-parameter constructor for just a numerator (int). This would make the Fraction represent a whole number.
- A one-parameter constructor with a double. This would make a Fraction by multiplying the double by 100000, then creating a numerator and denominator as the result. For example, PI, 3.14159, would be saved in a Fraction object as 314159/100000
- A two-parameter constructor with both numerator (int) and denominator (int). This would make a Fraction with both a numerator and denominator
- Add function taking a Fraction as a parameter and returning a Fraction
- Subtract function taking a Fraction as a parameter and returning a Fraction
- Multiply function taking a Fraction as a parameter and returning a Fraction
- Divide function taking a Fraction as a parameter and returning a Fraction
- Add function taking two Fractions as parameters and updating the current object
- Subtract function taking two Fractions as parameters and updating the current object
- Multiply function taking two Fractions as parameters and updating the current object
- Divide function taking two Fractions as parameters and updating the current object
Make sure to use exception handling in the event there is ever a divide by zero or any other error condition that would cause the program to terminate unexpectedly.
In the main program, create a looping menu driven interface, which allows the user to enter in two Fractions using the Input() function and a mathematical operation. After the operation, print the Fraction result using the Print() function. The loop ends when the user enters Q or q to quit for the math operation.
Please upload the following:
- The main program including .h and .cpp, please don't upload .sln, or .project or .exe
- Any input or output data files that you create
- Screen shots of an entire output with the result.
my code:
#include
//creating class to store information of fractions class Fraction { private: int numerator; int denominator; public: void input(); void print(); //default constructor Fraction(); //constructor for numerator int to make a fraction whole number Fraction(int n); //constructor with double Fraction(double); //constructor with two parameter for both int numerator and denominator Fraction(int&, int&); Fraction Add(Fraction&); Fraction Subract(Fraction&); Fraction multiply(Fraction&); Fraction divide(Fraction&); void Add(Fraction, Fraction); void Subract(Fraction, Fraction); void multiply(Fraction, Fraction); void divide(Fraction, Fraction);
};
//fraction.cpp
//fraction.cpp #include
// defining the input function to allow user to enter the numerator and denominator void Fraction::input() { // Ask user to enter numerator value and store it cout << "Please enter numerator: "; cin >> numerator;
// Ask user to enter numerator valueand store it
if (denominator == 0) { cout << "Sorry denomiantor can't be zero"; } else { cout << "Please enter denominator not equals to zero: "; cin >> denominator; }
}
//defining the Print function which will print out the Fraction in the form numerator / denominator i.e. 2 / 3 void Fraction::print() { cout << numerator << "/" << denominator << endl; }
// defining the default ocnstructor Fraction::Fraction() { numerator = 0; denominator = 0;
}
// defining the parameterized constructor for numerator int to make a fraction whole number Fraction::Fraction(int n) { numerator = n; denominator = 1; }
//defining the parameterized constructor with double. This would make a Fraction by multiplying the double by 100000, then creating a numerator and denominator as the result. For example, PI, 3.14159, would be saved in a Fraction object as 314159/100000 Fraction::Fraction(double x) { numerator = x * 100000; denominator = 100000; }
//defining a constructor with two-parameter with both numerator (int) and denominator (int). This would make a Fraction with both a numerator and denominator Fraction::Fraction(int& n, int& d) {
// if denomintaor is "0" if (d == 0) { //then set the numerator as 1 adn denominator as 0 n = 1; d = 0; //cout >> "denominator cannot be zero">>endl; } //else if denominator is less than 0 else if (d < 0) { //change the sign of numerator
numerator = 0 - d; denominator = 0 - n; } else { numerator = n; denominator = d; } }
//defining the Add function taking a Fraction as a parameter and returning a Fraction //Add function taking two Fractions as parameters and updating the current object f Fraction Fraction::Add(Fraction& obj_f) { obj_f.numerator= obj_f.numerator * denominator; numerator = numerator * obj_f.denominator; obj_f.numerator = obj_f.numerator + numerator; obj_f.denominator = obj_f.denominator * denominator; return obj_f;
}
// defining a Subtract function taking a Fraction as a parameter and returning a Fraction //Subtract function taking two Fractions as parameters and updating the current object Fraction Fraction::Subract(Fraction& obj_f) { obj_f.numerator = obj_f.numerator * denominator; numerator = numerator * obj_f.denominator; obj_f.numerator = obj_f.numerator - numerator; obj_f.denominator = obj_f.denominator * denominator; return obj_f; }
//Multiply function taking a Fraction as a parameterand returning a Fraction //Multiply function taking two Fractions as parametersand updating the current object Fraction Fraction::multiply(Fraction& obj_f) { obj_f.numerator = obj_f.numerator * numerator; obj_f.denominator = obj_f.denominator * denominator; return obj_f; }
//Divide function taking a Fraction as a parameterand returning a Fraction // Divide function taking two Fractions as parametersand updating the current object Fraction Fraction::divide(Fraction& obj_f) { if (obj_f.denominator == 0 || denominator == 0) { cout << "Sorry the result cannot divide by zero. please enter new number:" << endl; obj_f = { 86,86 };
} else obj_f.numerator = numerator * obj_f.denominator; obj_f.denominator = denominator * obj_f.numerator; return obj_f; } void Fraction:: Add(Fraction obj1, Fraction obj2) { numerator = obj2.numerator * obj1.denominator + obj1.numerator * obj2.denominator; denominator = obj2.denominator * obj1.denominator; }
void Fraction::Subract(Fraction obj1, Fraction obj2) { numerator = obj1.numerator * obj2.denominator - obj2.numerator* obj1.denominator; denominator = obj1.denominator * obj2.denominator; }
void Fraction::multiply(Fraction obj1, Fraction obj2) { numerator = numerator = obj1.numerator * obj2.numerator; denominator = numerator = obj1.denominator * obj2.denominator;
}
void Fraction::divide(Fraction obj1, Fraction obj2) { numerator = obj1.numerator * obj2.denominator; denominator = obj1.denominator * obj2.numerator; }
//main.cpp(please help with execution try catch, throw)
error i am dealing with uptill now:
warning C4244: '=': conversion from 'double' to 'int', possible loss of data : error C2679: binary '=': no operator found which takes a right-hand operand of type 'initializer list' (or there is no acceptable conversion) message : could be 'Fraction &Fraction::operator =(Fraction &&)' message : or 'Fraction &Fraction::operator =(const Fraction &)' : while trying to match the argument list '(Fraction, initializer list)' 1>Done building project "mp2_classes.vcxproj" -- FAILED. ========== Build: 0 succeeded, 1 failed, 0 up-to-date, 0 skipped ==========
my question:
it needs to be done in c++
i need help in main program with and without execution and exception handling
please use two screenshots, one with execution and one with no exceution
kindly please help me in removing errors
please don't copied the code from other chegg sources i want my code to be modified
Step by Step Solution
There are 3 Steps involved in it
Step: 1
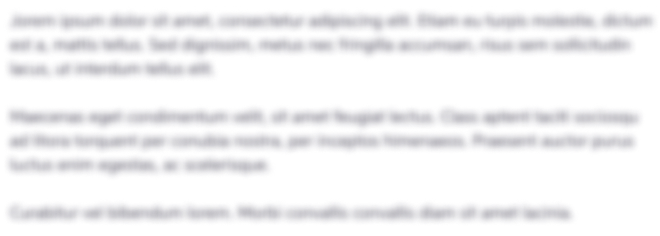
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started