Question
Question (PYTHON) Mycode.py and testprogram.py are two different files for object oriented and I keep receiving an error, any help for fixing error? I've also
Question
(PYTHON) Mycode.py and testprogram.py are two different files for object oriented and I keep receiving an error, any help for fixing error? I've also included the question to the assignment just in case its needed.
Assignment Question:
File #1 (below assignment question):
You creating a system that can be used by a real estate agency that will help them manage their property listing inventory. You will need to be able to create new property objects, add property listings to inventory when a customer wants list their property with the agency, change the listing status (for example, from "for sale" to "sold"), remove property listings from your inventory if the customer pulls their listing from the agency.
Design a class named Property_Inventory that contains:
■ A private int data field named id for the property. You will need to create a class level variable to keep track of the current ID available. Once you assign that ID value to the new property, you will need to increment the class level variable called auto_id.
■ A private string data field named address that stores the property address.
■ A private string data field named city that stores the city property resides in.
■ A private string data field named state that stores the state the property resides in. You should validate that the user only enters two characters to represent the state. You can also validate that a valid state was entered. To perform this validation, you should call an internal class method that is passed the value provided by the user (when instantiating the object) to a method which returns a boolean value indicating if the state is valid or not. If the state is not valid, display a message to the user and set the state to "??".
■ A private string data field named zip that stores the property zip code. You should verify that this zip code has a length of 5 and contains only digits, but needs to be stored in a string so that if the zip has a leading zero, the zero will be displayed.
■ A private string data field named status that stores the property status which be only be one of three values: for sale, for rent, or sold.
■ A private float data field named price that stores the property listing asking price.
■ A private int data field named beds that stores the number of bedrooms at the property (this should be zero for land and commercial type properties and you should validate that).
■ A private int data field named baths that stores the number of bathrooms at the property (this should be zero for land and you should validate that).
■ A private string data field named type that stores one of three values: residential, commercial, or land.
■ A private float data field named agency_fee that stores the fee the customer will pay to the agency. For example, it is common for the customer to pay a 3% fee so, in this case, the value stored in this field will be 3.00.
■ A __init__ that creates an inventory record with the specified defaults for: status (default "for sale"), price (default 0), beds (default 0) , baths (default 0), type (default "residential"), and agency_fee (default 3).
■ The accessor and mutator methods for all fields.
■ A method named display_all_properties that takes a propety type and status and displays all properties that match the type and status. For example, this method would display all residential properties that are for sale if the user requests those conditions. If the user provides an empty string for the property type and "sold" for the status, then this method would display all properties that have been sold. If the user provides an empty string for the status, but provides a property type of "land", then this method would display all "land" listings.
■ A method named discount_agency_fee that decreases the agency_fee by the discount value and property type passed to the method for all active (not sold) properties.
■ A method named mark_sold that will change the property status to "sold" and set the price to the sales price passed to the method.
■ A __str__ method that can be used to display the current state of a property listing.
File #2 (Under file#1 below):
Write a test program that:
1) Creates a "residential" Property_Inventory listing that provides values for every field.
2) Displays the property listing that was just added.
3) Creates two "land" property listings that do not provide values for some of the fields that have default values so that you can demonstrate that the default values are being used.
4) Create one more property listing that provides an invalid state value so that your validation message is triggered and "??" will be provided for the state value.
5) Display all of the "for sale" properties.
6) Display all of the "land" properties that are for sale.
7) Sell a "land" property.
7) Discount the agency_fees for all "land" properties by 1%.
8) Display all "land" property listing so that we can verify that only properties that had not sold had their agency_fees adjusted.
Your class and test program should be stored in different .py files.
File #1
mycode.py:
class Property_Inventory:
auto_id = 1
def __init__(self, address, city, state, zip_code, status="for sale", price=0, beds=0, baths=0, type="residential", agency_fee=3.00):
self.__id = Property_Inventory.auto_id
Property_Inventory.auto_id += 1
self.__address = address
self.__city = city
self.__state = state
self.__zip_code = zip_code
self._status = status
self._price = float(price)
self.__beds = int(beds)
self.__baths = int(baths)
self._type = type
self._agency_fee = agency_fee
def set_state(self, state):
if len(state) == 2 and state.isalpha(): #validates that the user only enters two characters and that its only alphabetic letters
self.__state = state.upper() #sets the state and converts it to upper, if typed lowercase it uppers
else: #else the state is invalid it sets to ??
self.__state = "??"
def set_zip(self, zip_code):
if len(zip_code) == 5 and zip_code.isdigit(): #verifies the zip code has length of 5 and contains only digits and stores it in a string
self.__zip_code = zip_code #if the zip has a leading zero the zeros will display
else:
self.__zip_code = "00000"
def set_beds(self, beds):
if self._type == "land" or self._type == "commercial": #sets the number of bedrooms the property has if in land or commercial it should be set to zero
self.__beds = 0
else:
self.__beds = beds
def set_baths(self, baths):
if self._type == "land": #stores the number of bathrooms at the property, for land it is set to 0
self.__baths = 0
else:
self.__baths = baths
def display_all_properties(self, property_type="", property_status=""): #takes a propety type and status and displays all properties that match the type and status
for prop in property_inventory:
if (not property_type or prop._type == property_type) and (not property_status or prop._status == property_status): #checks if property matches the specified 'type' and 'status'
print(prop)
def discount_agency_fee(self, discount, property_type): #decreases the agency fee by discount value and type passed for not sold properties
for prop in property_inventory:
if prop._type == property_type and prop._status != "sold": #checks if property is not sold and matches the matching property type
prop._agency_fee -= discount
def mark_sold(self, sales_price): ##change the property status to "sold" and set the price to the sales price passed to the method.
if self._status != "sold":
self._status = "sold"
self._price = sales_price #sets property price to sales_price
def __str__(self): #returns string reprensentation of listings
return f"Property ID: {self.__id}\nAddress: {self.__address}, {self.__city}, {self.__state} {self.__zip_code}\nStatus: {self._status}\nPrice: ${self._price:.2f}\nBeds: {self.__beds}\nBaths: {self.__baths}\nType: {self._type}\nAgency Fee: {self._agency_fee}%"
File #2:
testprogram.py
from mycode import Property_Inventory
property_inventory = []
# Create a residential property
residential_property = Property_Inventory("123 Embe St", "Cityville", "TX", "12345", "for sale", 250000, 3, 2, "residential", 3.00)
property_inventory.append(residential_property)
# Display the residential property
print("Residential Property:")
print(residential_property)
# Create two land properties with defaults
land_property1 = Property_Inventory("456 Strawberry Ave", "Townsville", "TX", "54321", "for sale", 200000, 3, 2, type="land")
property_inventory.append(land_property1)
land_property2 = Property_Inventory("789 Berry Dr", "Beach City", "TX", "98765", "for sale", 230000, 3, 3, type="land")
property_inventory.append(land_property2)
# Create a property with an invalid state
invalid_state_property = Property_Inventory("10 Invalid St", "Errorville", "Texas123", "00000", "for sale")
property_inventory.append(invalid_state_property)
# Display all "for sale" properties
print("\nAll 'for sale' properties:")
for sale_properties in property_inventory:
if sale_properties._status == "for sale":
print(sale_properties)
# Display all "land" properties that are for sale
print("\nAll 'land' properties for sale:")
for land_properties in property_inventory:
if land_properties._type == "land" and land_properties._status == "for sale":
print(land_properties)
# Sell a "land" property
land_property1.mark_sold(10000)
print("\nMarked land_property1 as sold.")
# Discount the agency fees for all "land" properties by 1%
print("\nDiscounted agency fees for 'land' properties by 1%.")
land_property1.discount_agency_fee(1.0, "land")
# Display all "land" property listings to verify agency fee adjustment
print("\nAll 'land' properties after agency fee adjustment:")
for land_properties in property_inventory:
if land_properties._type == "land":
print(land_properties)
Step by Step Solution
3.48 Rating (155 Votes )
There are 3 Steps involved in it
Step: 1
It seems like you are working on an objectoriented programming assignment in Python and encountering ...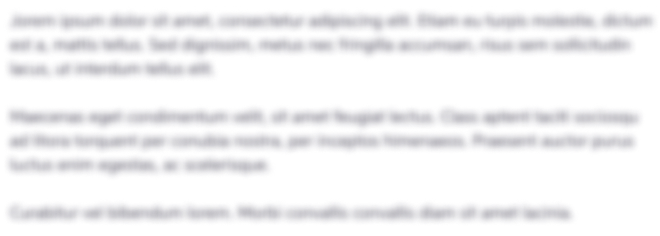
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started