Question
Question: The program sorts user input in ascending order. It accepts a -n argument to indicate that the input will be numeric. The default is
Question:
The program sorts user input in ascending order. It accepts a -n argument to indicate that the input will be numeric. The default is a string of characters. Modify the program to handle a -r flag, which indicates sorting in reverse (decreasing) order. Be sure that -r work with -n.
code:
#include
#define MAXLINES 5000 char *lineptr[MAXLINES];
int readlines(char *lineptr[], int nlines); void writelines(char *linptr[], int nlines);
void qsort_(void *lineptr[], int left, int right, int (*comp)(void *, void *));
int numcmp(char *, char *);
int main(int argc, char* argv[]) { int nlines; int numeric =0; if(argc > 1 && strcmp(argv[1], "-n") ==0) numeric=1; if ((nlines = readlines(lineptr, MAXLINES)) >= 0) { qsort_((void **) lineptr, 0, nlines-1, (int (*)(void*, void*))(numeric ? numcmp : strcmp)); writelines(lineptr, nlines); return 0; } else { printf("input too big to sort "); return 1; }
return 0; }
void qsort_(void *v[], int left, int right, int (*comp)(void *, void *)) { int i, last; void swap(void *v[], int, int); if (left >= right) return; swap(v, left, (left +right)/2); last = left; for (i = left +1; i
int numcmp(char *s1, char *s2){ double v1, v2; v1 = atof(s1); v2 = atof(s2); if (v1
void swap(void *v[], int i, int j){ void *temp; temp = v[i]; v[i] = v[j]; v[j] = temp; }
#define MAXLEN 1000 int getline_(char *, int); char *alloc(int);
int readlines(char *lineptr[], int maxlines){ int len, nlines; char *p, line[MAXLEN]; nlines = 0; while ((len = getline_(line, MAXLEN)) > 0){ //printf("%s\t%d ", line, len); if (nlines >= maxlines || (p = alloc(len)) == NULL){ return -1; } else{ line[len - 1] = '\0'; strcpy(p, line); lineptr[nlines++] = p; } //printf("p=%s\t%p ", p, p); } return nlines; }
void writelines(char *lineptr[], int nlines){ int i; while (nlines-- > 0) printf ("%s ", *lineptr++); }
int getline_(char *s, int lim){ int c, i; for (i=0; i #define ALLOCSIZE 10000 static char allocbuf[ALLOCSIZE]; static char* allocp = allocbuf; char *alloc(int n){ //return point to n characters if (allocbuf + ALLOCSIZE - allocp >= n){ allocp+=n; return allocp - n; } /ot enough room in the buffer return 0; } I am as specific as possible, this was actually the question and these changes are necessary. So I explained what format the code was in and what changes are required.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
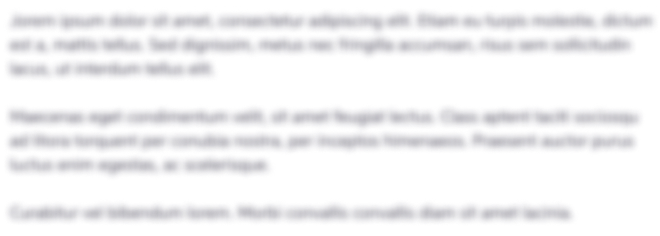
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started