Question
Questions 1-3 are based upon the following code: public class Test { public static void main(String[] args) { System.out.println( multi(5) ); } public static int
Questions 1-3 are based upon the following code:
public class Test
{
public static void main(String[] args)
{
System.out.println( multi(5) );
}
public static int multi(int n)
{
System.out.println(n);
return n * n;
}
}
1. What does the following program display?
a. The program displays 25.
b. The program displays 25 followed by 5.
c. The program displays 5 followed by 25.
d. The program displays 5 followed by 25 followed by 25.
2. True or false?
The Test class has two method definitions.
3. multi is a(n) ______________.
a. void method
b. class
c. static method
d. object
Questions 4-6 are based on the following code:
public static void nPrint(String message, int n)
{
while (n > 0)
{
System.out.print(message);
n--;
}
}
4. The above code is an example of:
a. A variable declaration
b. A while loop
c. A class definition
d. A method definition
5. What is the printout of the call: nPrint("a", 4);
a. aaaaa
b. aaaa
c. aaa
d. aa
6.
What is the value of the identifier k after the following code fragment is evaluated?
int k = 2;
nPrint("A message", k+1);
a. 0
b. 1
c. 2
d. 3
7.
Analyze the following code.
public class Test
{
public static void main(String[] args)
{
int n = 2;
xMethod(n);
System.out.println("n is " + n);
}
public static void xMethod(int n)
{
n++;
}
}
a. The code prints n is 0.
b. The code prints n is 1.
c. The code prints n is 2.
d.The code prints n is 3.
8. Which of the following is false about using methods.
a. Using methods makes a program run faster.
b. Using methods makes reusing code easier
c. Using methods makes programs easier to read.
d. Using methods hides detailed implementation from the client who calls the method.
9. Which of the following is false about the constructor method of a class
a. It can be overloaded.
b. It is always called after the keyword create.
c. The name of the constructor is the same as the class.
d. It may be sent any number of actuals, depending upon the definition.
10.
Which of the following is NOT an example of instantiating an object.
a. Keyboard k; k = new Keyboard( );
b. InputFile infile = new InputFile( fn );
c. String s = abcd;
d. int x = 100;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
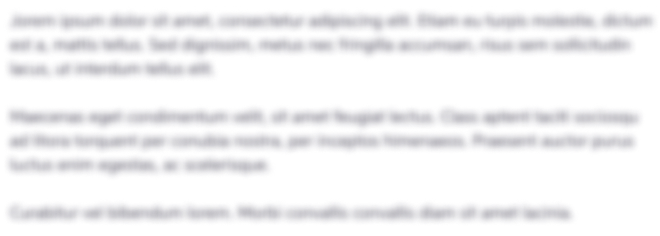
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started