Question
Requriements Submit only the files requested Print all floats to 2 decimal points unless stated otherwise Restrictions No global variables may be used Your program
Requriements
Submit only the files requested
Print all floats to 2 decimal points unless stated otherwise
Restrictions
No global variables may be used
Your program must have at least 1 user defined function
Description
For this program you will be implementing one attack in a turn based battle game.
Each character has the following stats
Stat | Description |
HP | How much life the character has left |
Strength | Attacks deal damage between their strength and half their strength rounded down. For example if a characters strength was 9 they could do between 4 and 9 points of damage |
Accuracry | The odds of landing an attack |
Dodge | The odds of avoiding an attack |
Crit Chance | The odds of landing a critical hit. A critical hit never misses and does a strength + 1 damage |
The game works as follows
The attacker has a critical hit chance. If they score a critical hit their attack cannot miss and they do their strength + 1 damage
If the attacker does not score a critical hit they make a normal attack based on their accuracry
If the attacker would successfully land a hit the defender attempts to dodge the attack based on their dodge stat
If the dodge is successful the defender receives no damage
If the dodge fails the defender takes a random amount of damage between the attacker's strength and half the attacker's strength rounded down
Damage is dealt in integer units
If the attacker fails to hit the defender because they missed or the defender dodged the attack the defender makes a counter attack
The attacker can dodge this attack but not counter attack back
Additionaly the defender could have been in a guarded stance
If the defender was in a guarded stance then they will counter attack regardless of whether they dodge or not
If however the attacker manages to kill the defender, reduce their hp to 0, the defender does not get to counter attack because they are dead
Your program should report crits, hits, misses, dodges, and how much health both the attacker and defender have at the end.
Your program should be able to handle both upper and lower case letters for the guard command
Assumptions
Input will always be valid
Tips
In order to implement the random parts of this problem you will need to import randomrandom.random can be used to generate a random value between 0 and 1
Use this for determing hits and misses
random.randint(start, stop) can be used to generate a random integer between [start, stop]
Use this for figuring out how much damage was done if an attack connects
Don't forget to call random.seed at the beginning of the program but make sure to only call it once
Your calls to the functions must be in the same order as mine and you cannot make more or less than I did
If you do your output won't match mine
Only make a call to the functions in the random module if you absolutely need to
For example if you crit you shouldn't be making any calls to see if you hit or if the defender dodges
The return values from random may be slightly different than mine on your computer but if you have the right logic it will work on Mimir
Especially true for Mac users
Examples
In the examples below user input has been underlined. You don't have to do any underlining in your program. It is just there to help you differentiate between what is input and what is output.
Example 1
Enter the seed to run the fight with: 2 Enter the attacker's hp: 10 Enter the attacker's strength: 10 Enter the attacker's accuracy: 80 Enter the attacker's crit chance: 20 Enter the attacker's dodge rate: 50 Enter the defender's hp: 10 Enter the defender's strength: 10 Enter the defender's accuracy: 80 Enter the defender's crit chance: 20 Enter the defender's dodge rate: 50 Is the defender guarding? Y for yes, n for no: n attacker missed defender defender crit attacker for 11 points of damage After fighting the attacker has 0 hp left and the defender has 10 hp left
Example 2
Enter the seed to run the fight with: 3 Enter the attacker's hp: 10 Enter the attacker's strength: 10 Enter the attacker's accuracy: 80 Enter the attacker's crit chance: 20 Enter the attacker's dodge rate: 50 Enter the defender's hp: 10 Enter the defender's strength: 10 Enter the defender's accuracy: 82 Enter the defender's crit chance: 20 Enter the defender's dodge rate: 50 Is the defender guarding? Y for yes, n for no: n defender dodged attacker's attack attacker dodged defender's attack After fighting the attacker has 10 hp left and the defender has 10 hp left
Example 3
Enter the seed to run the fight with: 5 Enter the attacker's hp: 10 Enter the attacker's strength: 10 Enter the attacker's accuracy: 95 Enter the attacker's crit chance: 20 Enter the attacker's dodge rate: 50 Enter the defender's hp: 3 Enter the defender's strength: 10 Enter the defender's accuracy: 80 Enter the defender's crit chance: 20 Enter the defender's dodge rate: 5 Is the defender guarding? Y for yes, n for no: n attacker hit defender for 10 points of damage After fighting the attacker has 10 hp left and the defender has 0 hp left
Step by Step Solution
There are 3 Steps involved in it
Step: 1
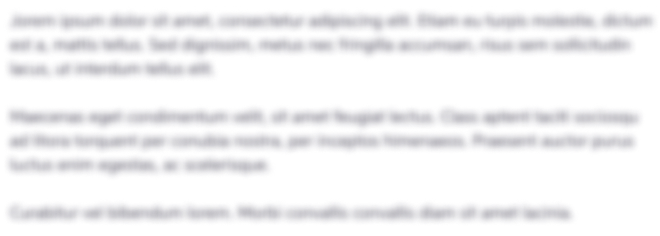
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started