Question
Reveiwing the following code please answer the following questions 1. Discuss the data structures used 2. Discuss the algorithm used to search the list for
Reveiwing the following code please answer the following questions
1. Discuss the data structures used
2. Discuss the algorithm used to search the list for a particular video and the time complexity of the algorithm
#include
VideoType::VideoType(const std::wstring &movieTitle, const std::wstring &producer, const std::wstring &director, const std::wstring &company, int noOfCopies) { this->movieTitle = movieTitle; this->producer = producer; this->director = director; this->company = company; this->noOfCopies = noOfCopies; }
std::wstring VideoType::getMovieTitle() { return movieTitle; }
void VideoType::setMovieTitle(const std::wstring &movieTitle) { this->movieTitle = movieTitle; }
std::wstring VideoType::getProducer() { return producer; }
void VideoType::setProducer(const std::wstring &producer) { this->producer = producer; }
std::wstring VideoType::getDirector() { return director; }
void VideoType::setDirector(const std::wstring &director) { this->director = director; }
std::wstring VideoType::getCompany() { return company; }
void VideoType::setCompany(const std::wstring &company) { this->company = company; }
int VideoType::getNoOfCopies() { return noOfCopies; }
void VideoType::setNoOfCopies(int noOfCopies) { this->noOfCopies = noOfCopies; }
bool VideoType::rentVideo(CustomerType *customer) { if (noOfCopies > 0) { customer->addVideo(this); noOfCopies--; return true; } return false; }
bool VideoType::returnVideo(CustomerType *cusomer) { if (cusomer->hasVideo(this)) { cusomer->returnVideo(this); noOfCopies++; return true; } return false; }
bool VideoType::isAvailableInStore() { return noOfCopies > 0? true: false; }
std::wstring VideoType::toString() { return std::wstring(L"Title: ") + movieTitle + std::wstring(L" Producer: ") + producer + std::wstring(L" Director: ") + director + std::wstring(L" Company: ") + company + std::wstring(L" Stock: ") + std::to_wstring(noOfCopies); }
#include
public: CustomerType::java *import;
class CustomerType { private: std::wstring firstName; std::wstring lastName; std::wstring accountNumber; std::vector
#include
class StringBuilder { private: std::wstring privateString;
public: StringBuilder() { }
StringBuilder(const std::wstring &initialString) { privateString = initialString; }
StringBuilder(std::size_t capacity) { ensureCapacity(capacity); }
wchar_t charAt(std::size_t index) { return privateString[index]; }
StringBuilder *append(const std::wstring &toAppend) { privateString += toAppend; return this; }
template
StringBuilder *insert(std::size_t position, const std::wstring &toInsert) { privateString.insert(position, toInsert); return this; }
template
std::wstring toString() { return privateString; }
std::size_t length() { return privateString.length(); }
void setLength(std::size_t newLength) { privateString.resize(newLength); }
std::size_t capacity() { return privateString.capacity(); }
void ensureCapacity(std::size_t minimumCapacity) { privateString.reserve(minimumCapacity); }
StringBuilder *remove(std::size_t start, std::size_t end) { privateString.erase(start, end - start); return this; }
StringBuilder *replace(std::size_t start, std::size_t end, const std::wstring &newString) { privateString.replace(start, end - start, newString); return this; }
private: template
CustomerType::CustomerType(const std::wstring &firstName, const std::wstring &lastName, const std::wstring &accountNumber) { this->firstName = firstName; this->lastName = lastName; this->accountNumber = accountNumber; }
std::wstring CustomerType::getFirstName() { return firstName; }
void CustomerType::setFirstName(const std::wstring &firstName) { this->firstName = firstName; }
std::wstring CustomerType::getLastName() { return lastName; }
void CustomerType::setLastName(const std::wstring &lastName) { this->lastName = lastName; }
std::wstring CustomerType::getAccountNumber() { return accountNumber; }
void CustomerType::setAccountNumber(const std::wstring &accountNumber) { this->accountNumber = accountNumber; }
void CustomerType::addVideo(VideoType *v) { rentedVideos.push_back(v); }
std::wstring CustomerType::toString() { StringBuilder *s = new StringBuilder(); s->append(std::wstring(L"Name: ") + firstName + std::wstring(L" ") + lastName + std::wstring(L" Account Number: ") + accountNumber + std::wstring(L" Rented Videos : ")); std::vector
void CustomerType::returnVideo(VideoType *video) {
rentedVideos.remove(video);
}
bool CustomerType::hasVideo(VideoType *video) { if (rentedVideos.find(video) != -1) { return true; } else { return false; }
}
//.h file code:
#include
public: VideoStore::java *import; class VideoStore { public: static std::vector
std::vector
void VideoStore::createVideoList() {
try { BufferedReader *videoFile = nullptr; FileReader tempVar(L"videos.txt"); videoFile = new BufferedReader(&tempVar); videoFile->readLine(); // to flush comment line for (std::wstring line = videoFile->readLine(); (line != L""); line = videoFile->readLine()) {
std::vector
void VideoStore::createCustomerList() {
try { BufferedReader *customerFile = nullptr; FileReader tempVar(L"customers.txt"); customerFile = new BufferedReader(&tempVar); customerFile->readLine(); // to flush comment line for (std::wstring line = customerFile->readLine(); (line != L""); line = customerFile->readLine()) {
std::vector
CustomerType *VideoStore::getCustomer() { std::wcout << std::wstring(L"Enter Customer Id") << std::endl; for (int i = 0; i < listOfCustomers.size(); i++) { std::wcout << std::wstring(L"Id:") << (i << 1) << std::wstring(L"\t") << listOfCustomers[i]->getFirstName() << std::endl; } int custId = s->nextInt(); if (custId > 0 && custId <= listOfCustomers.size()) { return listOfCustomers[custId - 1]; } else { std::wcout << std::wstring(L"Invalid Customer Id") << std::endl; return nullptr; } }
VideoType *VideoStore::getVideo() { std::wcout << std::wstring(L"Enter Video Id") << std::endl; for (int i = 0; i < listOfVideos.size(); i++) { std::wcout << std::wstring(L"Id:") << (i << 1) << std::wstring(L"\t") << listOfVideos[i]->getMovieTitle() << std::endl; } int videoId = s->nextInt(); if (videoId > 0 && videoId <= listOfVideos.size()) { return listOfVideos[videoId - 1]; } else { std::wcout << std::wstring(L"Invalid Video Id") << std::endl; return nullptr; } } #include
/* * Driver function */ static void main(std::vector
//.cpp file code:
void
int
Step by Step Solution
There are 3 Steps involved in it
Step: 1
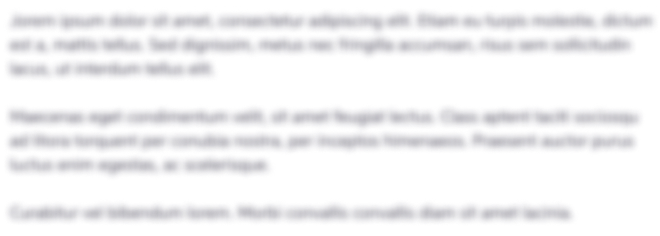
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started