Question
Revise both the servlet file named RegistrationWithHttpSession.java in Listing 37.11 and RegistrationWithHttpSession.html file, to register staff information in Staff table as shown in the following
Revise both the servlet file named RegistrationWithHttpSession.java in Listing 37.11 and RegistrationWithHttpSession.html file, to register staff information in Staff table as shown in the following figures. Attached both files along with the assignment document.
/*
* To change this license header, choose License Headers in Project
Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
import java.sql.*;
/**
*
* @author moaabdel
*/
public class RegistrationWithHttpSession extends HttpServlet {
// Use a prepared statement to store a student into the database
private PreparedStatement pstmt;
/** Initialize variables */
public void init() throws ServletException {
initializeJdbc();
}
/** Process the HTTP Get request */
public void doGet(HttpServletRequest request, HttpServletResponse
response) throws ServletException, IOException {
// Set response type and output stream to the browser
response.setContentType("text/html");
PrintWriter out = response.getWriter();
// Obtain data from the form
String lastName = request.getParameter("lastName");
String firstName = request.getParameter("firstName");
String mi = request.getParameter("mi");
String telephone = request.getParameter("telephone");
String email = request.getParameter("email");
String street = request.getParameter("street");
String city = request.getParameter("city");
String state = request.getParameter("state");
String zip = request.getParameter("zip");
if (lastName.length() == 0 || firstName.length() == 0) {
out.println("Last Name and First Name are required");
}
/*
* To change this license header, choose License Headers in Project
Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
import java.sql.*;
/**
*
* @author moaabdel
*/
public class RegistrationWithHttpSession extends HttpServlet {
// Use a prepared statement to store a student into the database
private PreparedStatement pstmt;
/** Initialize variables */
public void init() throws ServletException {
initializeJdbc();
}
/** Process the HTTP Get request */
public void doGet(HttpServletRequest request, HttpServletResponse
response) throws ServletException, IOException {
// Set response type and output stream to the browser
response.setContentType("text/html");
PrintWriter out = response.getWriter();
// Obtain data from the form
String lastName = request.getParameter("lastName");
String firstName = request.getParameter("firstName");
String mi = request.getParameter("mi");
String telephone = request.getParameter("telephone");
String email = request.getParameter("email");
String street = request.getParameter("street");
String city = request.getParameter("city");
String state = request.getParameter("state");
String zip = request.getParameter("zip");
if (lastName.length() == 0 || firstName.length() == 0) {
out.println("Last Name and First Name are required");
}
else {
// Create an Address object
Address address = new Address();
address.setLastName(lastName);
address.setFirstName(firstName);
address.setMi(mi);
address.setTelephone(telephone);
address.setEmail(email);
address.setStreet(street);
address.setCity(city);
address.setState(state);
address.setZip(zip);
// Get an HttpSession or create one if it does not exist
HttpSession httpSession = request.getSession();
// Store student object to the session
httpSession.setAttribute("address", address);
// Ask for confirmation
out.println("You entered the following data");
out.println("
Last name: " + lastName);
out.println("
First name: " + firstName);
out.println("
MI: " + mi);
out.println("
Telephone: " + telephone);
out.println("
Email: " + email);
out.println("
Address: " + street);
out.println("
City: " + city);
out.println("
State: " + state);
out.println("
Zip: " + zip);
// Set the action for processing the answers
out.println("
");}
out.close(); // Close stream
}
/** Process the HTTP Post request */
public void doPost(HttpServletRequest request, HttpServletResponse
response) throws ServletException, IOException {
// Set response type and output stream to the browser
response.setContentType("text/html");
PrintWriter out = response.getWriter();
// Obtain the HttpSession
HttpSession httpSession = request.getSession();
// Get the Address object in the HttpSession
Address address = (Address)(httpSession.getAttribute("address"));
else {
// Create an Address object
Address address = new Address();
address.setLastName(lastName);
address.setFirstName(firstName);
address.setMi(mi);
address.setTelephone(telephone);
address.setEmail(email);
address.setStreet(street);
address.setCity(city);
address.setState(state);
address.setZip(zip);
// Get an HttpSession or create one if it does not exist
HttpSession httpSession = request.getSession();
// Store student object to the session
httpSession.setAttribute("address", address);
// Ask for confirmation
out.println("You entered the following data");
out.println("
Last name: " + lastName);
out.println("
First name: " + firstName);
out.println("
MI: " + mi);
out.println("
Telephone: " + telephone);
out.println("
Email: " + email);
out.println("
Address: " + street);
out.println("
City: " + city);
out.println("
State: " + state);
out.println("
Zip: " + zip);
// Set the action for processing the answers
out.println("
");}
out.close(); // Close stream
}
/** Process the HTTP Post request */
public void doPost(HttpServletRequest request, HttpServletResponse
response) throws ServletException, IOException {
// Set response type and output stream to the browser
response.setContentType("text/html");
PrintWriter out = response.getWriter();
// Obtain the HttpSession
HttpSession httpSession = request.getSession();
// Get the Address object in the HttpSession
Address address = (Address)(httpSession.getAttribute("address"));
try {
storeStudent(address);
out.println(address.getFirstName() + " " + address.getLastName()
+ " is now registered in the database");
out.close(); // Close stream
}
catch(Exception ex) {
out.println("Error: " + ex.getMessage());
}
}
/** Initialize database connection */
private void initializeJdbc() {
try {
// Load the JDBC driver
Class.forName("com.mysql.jdbc.Driver");
System.out.println("Driver loaded");
// Establish a connection
Connection conn = DriverManager.getConnection
("jdbc:mysql://localhost/test" , "INFO211", "java2");
System.out.println("Database connected");
// Create a Statement
pstmt = conn.prepareStatement("insert into Address " +
"(lastName, firstName, mi, telephone, email, street, city, "
+ "state, zip) values (?, ?, ?, ?, ?, ?, ?, ?, ?)");
}
catch (Exception ex) {
System.out.println(ex);
}
}
/** Store an address to the database */
private void storeStudent(Address address) throws SQLException {
pstmt.setString(1, address.getLastName());
pstmt.setString(2, address.getFirstName());
pstmt.setString(3, address.getMi());
pstmt.setString(4, address.getTelephone());
pstmt.setString(5, address.getEmail());
pstmt.setString(6, address.getStreet());
pstmt.setString(7, address.getCity());
pstmt.setString(8, address.getState());
pstmt.setString(9, address.getZip());
pstmt.executeUpdate();
}
}
class Address {
private String firstName;
private String mi;
private String lastName;
private String telephone;
private String street;
private String city;
private String state;
private String email;
private String zip;
public String getFirstName() {
return this.firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getMi() {
return this.mi;
}
public void setMi(String mi) {
this.mi = mi;
}
public String getLastName() {
return this.lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getTelephone() {
return this.telephone;
}
public void setTelephone(String telephone) {
this.telephone = telephone;
}
public String getEmail() {
return this.email;
}
public void setEmail(String email) {
this.email = email;
}
public String getStreet() {
return this.street;
}
public void setStreet(String street) {
this.street = street;
}
public String getCity() {
return this.city;
}
public void setCity(String city) {
this.city = city;
}
public String getState() {
return this.state;
}
public void setState(String state) {
this.state = state;
}
public String getZip() {
return this.zip;
}
public void setZip(String zip) {
this.zip = zip;
}
}
Please register to your instructor's student address book Last Name* Telephone Street City First Name * MI Email State Georgia-GA Zip SubmitReset *required fields Please register to your instructor's student address book Last Name* Telephone Street City First Name * MI Email State Georgia-GA Zip SubmitReset *required fieldsStep by Step Solution
There are 3 Steps involved in it
Step: 1
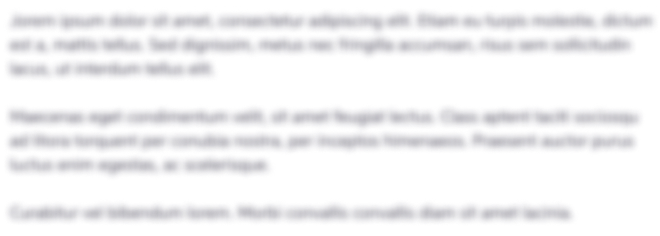
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started