Question
SavingsAccount is a class with two double* data members pointing to the balance and interest rate of the savings account, respectively. Two doubles are read
SavingsAccount is a class with two double* data members pointing to the balance and interest rate of the savings account, respectively. Two doubles are read from input to initialize userAccount. Use the copy constructor to create a SavingsAccount object named copyAccount that is a deep copy of userAccount.
Ex: If the input is 87.00 0.01, then the output is:
Original constructor called Produced a new SavingsAccount object userAccount: $87.00 with 1.00% interest rate copyAccount: $174.00 with 2.00% interest rate
#include
class SavingsAccount { public: SavingsAccount(double startingBalance = 0.0, double startingInterestRate = 0.0); SavingsAccount(const SavingsAccount& acc); void SetBalance(double newBalance); void SetInterestRate(double newInterestRate); double GetBalance() const; double GetInterestRate() const; void Print() const; private: double* balance; double* interestRate; };
SavingsAccount::SavingsAccount(double startingBalance, double startingInterestRate) { balance = new double(startingBalance); interestRate = new double(startingInterestRate); cout << "Original constructor called" << endl; }
SavingsAccount::SavingsAccount(const SavingsAccount& acc) { balance = new double; *balance = *(acc.balance); interestRate = new double; *interestRate = *(acc.interestRate); cout << "Produced a new SavingsAccount object" << endl; }
void SavingsAccount::SetBalance(double newBalance) { *balance = newBalance; }
void SavingsAccount::SetInterestRate(double newInterestRate) { *interestRate = newInterestRate; }
double SavingsAccount::GetBalance() const { return *balance; }
double SavingsAccount::GetInterestRate() const { return *interestRate; }
void SavingsAccount::Print() const { cout << fixed << setprecision(2) << "$" << *balance << " with " << *interestRate * 100 << "\% interest rate" << endl; }
int main() { double balance; double interestRate;
cin >> balance; cin >> interestRate;
SavingsAccount userAccount(balance, interestRate); /* Your code goes here */ copyAccount.SetBalance(copyAccount.GetBalance() * 2); copyAccount.SetInterestRate(copyAccount.GetInterestRate() * 2);
cout << "userAccount: "; userAccount.Print(); cout << "copyAccount: "; copyAccount.Print();
return 0; }
c++ and please please make it correct
Step by Step Solution
There are 3 Steps involved in it
Step: 1
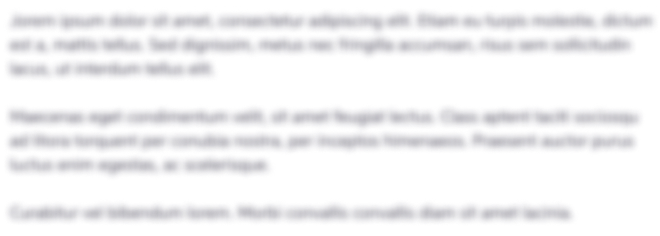
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started