Answered step by step
Verified Expert Solution
Question
1 Approved Answer
// ShowCircles.java // program using javafx to draw random circles // circles are colored based on the quadrant of the // pane they are in.
// ShowCircles.java // program using javafx to draw random circles // circles are colored based on the quadrant of the // pane they are in. import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.Pane; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.scene.shape.Line; import javafx.stage.Stage; public class ShowCircles extends Application { private final int MAX_RADIUS = 20; private final int MIN_RADIUS = 5; private final int PANE_SIZE = 500; @Override // Override the start method in the Application class public void start(Stage primaryStage) { // Create a pane to hold the circle Pane pane = new Pane(); // draw lines to see quadrants Line xLine = new Line(); xLine.setStartX(0.0f); xLine.setStartY(PANE_SIZE / 2); xLine.setEndX(PANE_SIZE); xLine.setEndY(PANE_SIZE / 2); pane.getChildren().add(xLine); Line yLine = new Line(); yLine.setStartY(0.0f); yLine.setStartX(PANE_SIZE / 2); yLine.setEndY(PANE_SIZE); yLine.setEndX(PANE_SIZE / 2); pane.getChildren().add(yLine); // Create a circle and add to pane // add a loop to draw 100 circles Circle circle = getCircle(); pane.getChildren().add(circle); // Create a scene and place it in the stage Scene scene = new Scene(pane, PANE_SIZE, PANE_SIZE); primaryStage.setTitle("ShowCircles"); // Set the stage title primaryStage.setScene(scene); // Place the scene in the stage primaryStage.show(); // Display the stage } // // method to create a circle // private Circle getCircle(){ Circle aCircle = new Circle(); // set radius to a random size between MIN_RADIUS and MAX_RADIUS int radius = (int)(Math.random()*(MAX_RADIUS-MIN_RADIUS))+MIN_RADIUS; // set circle in a random place on the pane aCircle.setCenterX((int)(Math.random()*PANE_SIZE)); aCircle.setCenterY((int)(Math.random()*PANE_SIZE)); aCircle.setRadius(radius); // Set color of circle based on quadrant // 1|2 blue| green // 3|4 red | yellow // can find the center of the circle // with the getCenterX() and getCenterY() methods // // remove the next line when setting the color // based on quadrant. aCircle.setFill(Color.BLACK); return aCircle; } }More fun with Circles The file ShowCircles.java contains an JavaFX Application 1. Compile and run the program. 2. Examine this program. It is similar to the previous ShowCircle.java but has a method to create a circle, getCirele0, that will randomly place set the circle's center in the pane. Also two lines are drawn on the pane that divide the pane in four quadrants. 3. Add code to the start) method to create 100 circles by calling getCircle) 100 times. Add each circle to the pane 4. Add code the the getCircle0 method to set the color of the circle by what quadrant its center is in. Blue for quadrant 1, green for quadrant 2, red for quadrant 3, and ycllow for quadrant 4 5. Compile and run, verifying your program works
Step by Step Solution
There are 3 Steps involved in it
Step: 1
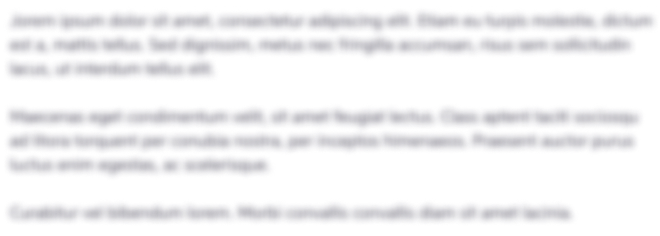
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started