Question
Simple Lisp Interpreter (Bison & Flex Programming) Design and implement the language for the following simple Lisp interpreter.Language design and implementation One statement (Lisp-expression) per
Simple Lisp Interpreter (Bison & Flex Programming)
Design and implement the language for the following simple Lisp interpreter.Language design and implementation One statement (Lisp-expression) per line. The return value of each statement will be output to console.
(stop) to terminate the program
constants: a number (the type of a number is double)
List expression and functions for basic arithmetic (+, -, *, /, 1+, 1-, ^)
where 1+ is to increment 1, 1- is to decrement 1,
^ is exponent (that is, (^ 2 3) is 2 to the power of 3.
(+ 1 2)
(+ 1.0 2.5)
(+ 1 2 3 4)
(+ (* 1 2) (- 3 4) )
variable (atom)
The length of a variable-name can be up to 255 characters long
Variable name begins with an alphabet and followed by alpha-numeric characters.
Support a symbol table to handle many variable entries (with C++ map)
A variable is either undefined or set for a number (type double).
let statement to set a variable with a number (to be converted to double), for example,
(let x 1.5)
g comparison:
EQ, LT, LE, GT, GE, NE
for equal to, less than, less than, greater than, greater than or equal to, or not equal
which is resulting in 0 (which means false) or non-zero (which means true)
(EQ 1 2) => 0 which means false
(EQ 1 1) => 1 which means true
if-list in (if condition then expression else expression), for example,
(if (EQ a 1) 1) ;; if a=1 then return 1
(if (EQ a 1) 1 2) ;; if a=1 then return 1 else return 2.
(if (GT a 1) (+ a 1) (- a 1))
comment ";" (as in lisp to ignore the rest of the line).
(symbol-table) will print the content of the symbol-table, in name-value (sorted by its name).
Support C-like integer-number with the base of either 10 or 8 (octal).
If a number (integer) begins with 0 then its base is octal; otherwise, it is decimal.
Note. You may need to detect a number (whether it is INTEGER or REAL) from your Lexer. If Integer, then check whether it begins with 0 for OCTAL integer or not for DECIMAL integer. Once it is identified as an integer (octal or decimal), you may return its token type and value properly.
[0-9]+ { yylval = atoi(yytext); return INTEGER; } ;; You may like to start this as a base.
Try your program with the sample test cases.
Test Case#
1.1
2.2.5
3.(+ 1 2)
4.(+ 1.0 2.5)
5.(+ 1 2 3 4 5 6 7 8 9 10)
6.(* 1 2 3 4 5 6 7 8 9 10)
7.(+ (* 2 2) 1 (- 5 4))
8.(1+ (* 2 3))
9.(^ 2 3)
10.(let x 1)
11.(let y 2)
12.(+ x y)
13.(let z 1234.56789)
14.(print z)
15.(if (EQ 1 1) 3 4)
16.(if (EQ 1 2) 3 4)
17.(if (EQ 1 2) (+ 1 2) (+ 3 4))
18.(stop)
19.(+ 1 2) ;;; this is comment
20.(+ 010 010) ;;; this is for Octal 10 and Octal 20 to be added. The result is in decimal.
21.(+ 010 20) ;;; this is for Octal 10 and decimal 20 to be added.
Place your results here (your unix or linux session-log, or MobaXterm save terminal text, console buffer log file) to show that you have done this item, and to submit this document and a zip file (to include all the flex and bison files, Makefile, and executables of your work).
Submit (upload to elearning) two items: (1) a word document [this document with your answers, including all the listings] of all of source codes,
Makefile, and a listing of compile and run (session log or screen-print), and (2) zip file containing all the source codes, Makefile, readme, any other files (to compile and run), and the executable
Step by Step Solution
There are 3 Steps involved in it
Step: 1
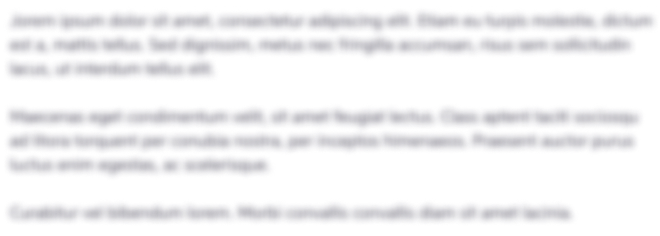
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started