Question
Simple Math Accumulator (Programming to an Interface) Summary Create a class ( SimpleMath ) that implements the AccumulatorInterface and allows the program LineCalculator.java to do
Simple Math Accumulator (Programming to an Interface)
Summary
Create a class (SimpleMath) that implements the AccumulatorInterface and allows the program LineCalculator.java to do simple math.
Details
I have provided you with a program (LineCalculator) that is supposed to let the user enter a simple math expression and report back the value of that expression. Unfortunately, it's missing a class: SimpleMath. This class allows us to enter numbers and operations in the usual (i.e. infix) order, but doesn't respect the normal "PEDMAS" order of operations. It implements the AccumulatorInterface, which has four methods:
int enterNumber(int n) which enters a number into the accumulator.
int enterOperator(char op) which enters an operator into the accumulator.
int getValue() which gets the current value of the accumulator.
void reset() which returns the accumulator to its initial state in preparation for a new calculation.
Our program gives the SimpleMath object numbers and operators in alternation, starting and ending with a number: a number, an operator, a number, an operator, ..., an operator, a number. For example, 10 * 5 + 3. Our simple math accumulator thus can't complete an operation until the following number has been entered. So operations actually occur when a number is entered. Thus for the expression above:
The 10 gets entered. The accumulator remembers and returns the 10 (but our program ignores this return value).
The * gets entered. The accumulator remembers the * and returns 10 (which, again, our program ignores).
The 5 gets entered. The accumulator multiplies the 10 by the 5 to get and return 50.
The + is entered. the accumulator remembers the + and returns the 50.
The 3 is entered. The accumulator adds the 3 to the 50 to get and return 53.
Note that the first number is handled differently than the others. If there is no operator to apply, then the number is just remembered. If there is an operator, it can be applied. The SimpleMath accumulator needs to know when it's in the middle of a calculation and when it's not. It should start NOT in the middle of a calculation, and then continue in the middle of a calculation until the reset method gets called.
A SimpleMath accumulator only recognizes the four operations +, -, * and / (plus, minus, times and divide). Other operations are ignored (as stated in the AccumulatorInterface specification.
Notes
Our SimpleMath accumulator does not respect the order of operations ("PEDMAS"). If we give it
10 + 5 * 3
it gives us 45 as the answer -- 10 plus 5 is 15, multiplied by 3 is 45. Later we may make a version that does.
Note that our SimpleMath accumulator could be used in way different than our progam does. For example, you might try the following code:
AccumulatorInterface sm = new SimpleMath(); sm.enterOperation('+'); for (int i = 1; i <= 10; ++i) { System.out.println(sm.enterNumber(i)); }
It should print the sums of the numbers from 1 to i (that is: 1, 3, 6, 10, ..., 55). Our sample program doesn't use it that way, but other programs could.
Grading Outline
60% -- Program performs as required
20% -- Program shows good design
20% -- Submitted material meets the standard requirements as described in the rules for submissions and the style rules.
_______________________________________________________________________________________________________________________________________________
import java.util.Scanner; import java.io.FileNotFoundException; import java.io.File; import java.util.NoSuchElementException; /** * A program that reads and evaluates simple math expressions using an * Accumulator. * * @author Mark Young (A00000000) */ public class LineCalculator { public static void main(String[] args) { // create required objects Scanner kbd = new Scanner(System.in); AccumulatorInterface sm = new SimpleMath(); // create necessary variables String line; Scanner input; int number; char op; // introduce yourself printIntroduction(); // loop until a blank line System.out.print(" Enter formula > "); line = kbd.nextLine(); while (!line.equals("")) { input = new Scanner(line); // read number/operator until line ends try { number = input.nextInt(); while (input.hasNext()) { // save the number sm.enterNumber(number); // get the operator op = input.next().charAt(0); sm.enterOperator(op); // get following number number = input.nextInt(); } // enter the last number and get the result sm.enterNumber(number); System.out.println(line + " = " + sm.getValue()); } catch (NoSuchElementException nse) { // report that that expression didn't work System.out.println("'" + line + "' is not a valid expression."); } // reset the accumulator to get rid of old values or garbage sm.reset(); // get next math expression System.out.print(" Enter formula > "); line = kbd.nextLine(); } System.out.println(" " + "Goodbye! "); } /** * Print the introduction to this program. */ private static void printIntroduction() { System.out.println(" " + "Line-Oriented Calculator " + "------------------------ " + " " + "This calculator does simple math. " + "Enter a simple math expression on each line, " + "and the calculator will tell you its value. " + "For example, enter the line " + " 10 + 5 * 3 " + "and the program will tell you it's 45. " + "(Note that the program does not do " + "order of operations! That's (10 + 5) * 3.) " + " " + "You can use only integer numbers, " + "and the +, -, * and / operators. " + " " + "Enter your math expression at the prompt. " + "Just leave the input line blank when " + "you're done. " + ""); } }
_______________________________________________________________________________________________________________________________________________
/** * An interface for an object that accepts operators and numbers (integer only) * and returns a value based on what operators and values it has accepted. * * @author Mark Young (A00000000) */ public interface AccumulatorInterface { /** * Enter an integer operand into the accumulator. * Return value is implementation-dependent, * but is typically the latest value of the accumulator. * * @param n the number to enter. * @return implementation dependent. */ public int enterNumber(int n); /** * Enter an operator into the accumulator. * Return value is implementation-dependent, * but is typically the latest value of the accumulator. * * @param op the operator to enter. * @return implementation dependent. */ public int enterOperator(char op); /** * Get the value from the accumulator. * * @return the value currently saved in the accumulator. */ public int getValue(); /** * Return the accumulator to its original state. */ public void reset(); }
_______________________________________________________________________________________________________________________________________________
BOLD FONT = User Inputs
OUTPUT:
Line-Oriented Calculator
------------------------
This calculator does simple math. Enter a simple math expression on each line, and the calculator will tell you its value. For example, enter the line 10 + 5 * 3 and the program will tell you it's 45. (Note that the program does not do order of operations! That's (10 + 5) * 3.)
You can use only integer numbers, and the +, -, * and / operators.
Enter your math expression at the prompt. Just leave the input line blank when you're done.
Enter formula > 1 + 1
1 + 1 = 2
Enter formula > 2 * 3
2 * 3 = 6
Enter formula > 40 - 5
40 - 5 = 35
Enter formula > 100 / 5
100 / 5 = 20
Enter formula > 1 + 2 + 3 + 4 + 5
1 + 2 + 3 + 4 + 5 = 15
Enter formula > 1 * 2 * 3 * 4 * 5
1 * 2 * 3 * 4 * 5 = 120
Enter formula > 10 + 5 * 3
10 + 5 * 3 = 45
Enter formula > 100 * 100 - 20 * 20
100 * 100 - 20 * 20 = 199600
Enter formula > 100 * 100
100 * 100 = 10000
Enter formula > 10000 - 20
10000 - 20 = 9980
Enter formula > 9980 * 20
9980 * 20 = 199600
Enter formula >
Goodbye!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
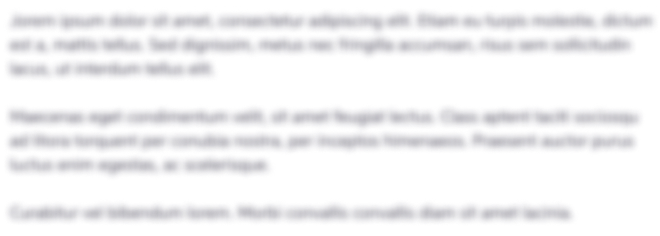
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started