Question
slot_unit.py import lab05 as lab05 import unittest from unittest.mock import patch from io import StringIO class TestLabFunctions(unittest.TestCase): def test_play_again_returns_True(self): user_input = [YeS] with patch('sys.stdout', new_callable=StringIO)
slot_unit.py
import lab05 as lab05 import unittest from unittest.mock import patch from io import StringIO
class TestLabFunctions(unittest.TestCase):
def test_play_again_returns_True(self):
user_input = ["YeS"] with patch('sys.stdout', new_callable=StringIO) as es: with patch('builtins.input', side_effect=user_input): results = lab05.play_again() self.assertTrue(results, "When input is yes the function should return True")
def test_play_again_returns_False_with_N_or_NO(self):
user_input = ["nO"] with patch('sys.stdout', new_callable=StringIO) as es: with patch('builtins.input', side_effect=user_input): results = lab05.play_again() self.assertFalse(results, "When input is No play_again should return False")
def test_play_again_warns_user_with_bad_input(self):
user_input = ["incorrect", "nO"] with patch('sys.stdout', new_callable=StringIO) as es: with patch('builtins.input', side_effect=user_input): results = lab05.play_again() self.assertFalse(results, "When input is no the play_again should return False") l = es self.assertIn("try again", l.getvalue().lower(), "Bad input should be followed with an error with at least Please Try again in it.")
def test_play_again_works_with_multiple_bad_inputs(self):
user_input = ["chiefs", "incorrect", "nO"] with patch('sys.stdout', new_callable=StringIO) as es: with patch('builtins.input', side_effect=user_input): results = lab05.play_again() self.assertFalse(results, "play_again should return False when given 2 incorrect inputs and then no")
def test_get_wager_returns_wager_amount(self):
user_input = ["30"] with patch('sys.stdout', new_callable=StringIO) as es: with patch('builtins.input', side_effect=user_input): results = lab05.get_wager(200) self.assertEqual(30, results, "getWager should return 30 when the user enters 30")
def test_get_wager_returns_wager_amount_after_negative(self):
user_input = ["-5", "45"] with patch('sys.stdout', new_callable=StringIO) as es: with patch('builtins.input', side_effect=user_input): results = lab05.get_wager(200) self.assertEqual(45, results, "getWager should return 45 when the user enters -5, 30")
def test_get_wager_returns_wager_amount_after_negative(self):
user_input = ["-5", "45"] with patch('sys.stdout', new_callable=StringIO) as es: with patch('builtins.input', side_effect=user_input): results = lab05.get_wager(200) self.assertEqual(45, results, "getWager should return 45 when the user enters -5, 30") l = es
def test_get_wager_returns_wager_amount_after_too_high_value(self):
user_input = ["105", "22"] with patch('sys.stdout', new_callable=StringIO) as es: with patch('builtins.input', side_effect=user_input): results = lab05.get_wager(30) self.assertEqual(22, results, "getWager should return 22 when the user enters 105, 22, and the bank is less than 105") l = es
def test_get_slot_results_returns_a_tuple_of_integers(self):
results = lab05.get_slot_results() self.assertIsInstance(results, tuple, "get_slot_results should return a tuple") self.assertEqual(3, len(results), "get_slot_results shoudl return 3 items") self.assertIsInstance(results[0], int, "Item at index 0 was not an integer") self.assertIsInstance(results[1], int, "Item at index 1 was not an integer") self.assertIsInstance(results[2], int, "Item at index 2 was not an integer")
def test_get_matches_returns_correct_number_of_matches(self):
results = lab05.get_matches(3, 3, 3) self.assertEqual(3, results, "get_matches returns 3 when given 3 matching values") results = lab05.get_matches(4, 4, 4) self.assertEqual(3, results, "get_matches returns 3 when given 3 matching values") results = lab05.get_matches(4, 4, 5) self.assertEqual(2, results, "get_matches returns 2 when given 2 matching values") results = lab05.get_matches(8, 4, 4) self.assertEqual(2, results, "get_matches returns 2 when given 2 matching values") results = lab05.get_matches(8, 4, 8) self.assertEqual(2, results, "get_matches returns 2 when given 2 matching values") results = lab05.get_matches(8, 4, 3) self.assertEqual(0, results, "get_matches returns 0 when given 0 matching values")
def test_get_payout_returns_correct_results(self):
results = lab05.get_payout(3, 0) self.assertEqual(-3, results, "get_payout returns -3 when given 3 and no matches.") results = lab05.get_payout(2, 3) self.assertEqual(18, results, "get_payout returns 8 when given 2 and 3 matches.") results = lab05.get_payout(5, 2) self.assertEqual(10, results, "get_payout returns 10 when given 5 and 2 matches.")
if __name__ == "__main__": unittest.main()
########### ## ## CS 101 Lab ## Program # ## Name ## Email TT ## TT ## PROBLEM : Describe the problem TT TT ## ALGORITHM : TT 1. Write out the algorithm TT ## ERROR HANDLING: TT ## Any Special Error handling to be noted. TT ## OTHER COMMENTS: ## Any special comments ## Wager not less than 0. etc ################# | #import modules needed def play_again() -> bool: "Asks the user if they want to play again, returns False if N or NO, and True if Y or YES. Keeps asking until they respond yes return True def get_wager (bank : int) -> int: "11 Asks the user for a wager chip amount. Continues to ask if they result is tuple: " Returns the result of the slot pull !!! return 1, 2, 3 def get_matches (reela, reelb, reelc) -> int: !!! Returns 3 for all 3 match, 2 for 2 alike, and 0 for none alike. " return 0 def get_bank() -> int: !!! Returns how many chips the user wants to play with. Loops until a value greater than 0 and less than 101 11 return 0 def get_payout (wager, matches): " Returns how much the payout is.. 10 times the wager if 3 matched, 5 times the wager if 2 match, and negative wager if O match " return wager * -1 if name main playing = True while playing: bank = get_bank() while True: # Replace with condition for if they still have money. wager = get_wager (bank) reell, reel2, reel3 = get_slot_results() matches = get_matches (reell, reel2, ree13) payout = get_payout (wager, matches) bank = bank + payout print("Your spin", reell, reel2, ree13) print("You matched", matches, "reels") print ("You won/lost", payout) print ("Current bank", bank) print() print ("You lost all", 0, "in", 0, "spins") print ("The most chips you had was", 0) playing = play_again() det best players Lab Week 5 - Functions with patch, Calablargon : Skills Needed to complete this Lab Crunciara . - All the youve marad din Lab05.py You will start out with labo5.py program. Notice that it has been stubbed out with functions and much of the main program has been written. Most of your work will just be in making each function work correctly. This can help you see how you can break a program down into smaller sub-problems which makes design and testing much easier. . Python 2.7.1 3.7.1:200cc, ct 20 30L1, 14:05:16 MSC 7.1915 33 bitince , 23 ( poredite for more information PEETART: CVbank peBox Work 101 Bolutionislot_Unit ut. RAL 10 tests 0.0 Creating Functions begin to Sed by All of these functions have been stubbed out to return a literal value. You can actually run the program and see it running. de functionne parameteri, parter2, ...): statement statement statement return return value ned For testing below, make sure that any functions are before the if name == " main section and anything in the main por- tion of the program is indented under this if statement. play_again() function The play_again () function will ask the user if they want to play again. It will return True if the user enters Y or YES regardless of case. The function will return False if the user enters Nor NO. If the user enters anything else then it will warn the user and ask them to enter another value. Functions make it much easier to debug a program as well. You can call the function from the command line multiple times to make sure it functions correctly. Once you are satisfied you can all the function in your program and be confident that the re- sults will be consistent Slot_UnitTests.py Slot_UnitTests will run your functions and check for valid re- sults. They don't test everything, but if you get all the tests passing then you'll have pretty good indication that you've writ- ten most of the functions effectively, To test this function, run the program like normal and then stop the execution by hitting ctrl-C, or command C on the mac. In the shell you can type the function as shown below to test the results You can edit and run the Slot_UnitTests.py in python just like your program. However, make sure you save your labo5.py program before you run the tests. It can't tell that you've got some unsaved changes in the editor. Slots In this program you are going to simulate a slot machine in Pierson Hall. The slot machine has 3 reels and each reel will have numbers ranging from 1 to 10. The user can choose how many chips they start out with. They are allowed to wager be- tween 1 to the amount of bank they currently have on each spin. If they match 3 numbers, they will win 10 times their wager. If they match 2 numbers they will win 3 times their wager. Examples get_bank() -> int: function Ask the user for how many chips they want to start with. Must be greater than 0 and less than 101. If not valid entry then it will warn the user and keep asking until they enter valid input and return the valid input. You matched o reels You won/lost -5 Current bank 5 Keyboard repl >> play again() Do you want to play wain? - You must unter YYESIN/NO LO Continue. Please Ley again De you want to play win? - 3 Please try again You must enter Y/YESIN/NO to continue Do you want to play againy True >> play again Do you want to play again? - You must enter Y/YES/N/NO to continue. Do you want to play again? How many chips do you want to wager? ==> 1 Your spin 9 6 6 You matched 2 reels You won/lost 2 Current bank 7 Please try again get_payout(wager, matches): function Returns the payout based on the # of matches and the wager, It assumes that the bank did not automatically initially subtract the wager. If matches is 3, then the payout is 10 times the wa- ger and subtract the wager. If matches is 2 then the payout is 3 times the wager and subtract the wager. If matches is 0, then the payout is the negative of the wager. get_wager(bank: int) -> int: function This function should return an integer. It asks the user how many chips they want to wager. It must be greater than 0, and less than or equal to the bank. Otherwise the user gets warned and they are prompted until they enter a valid value. How many chips do you want to wager? ==> ! The wager amount cannot be greater than how much you have. 7 How many chips do you want to wager? --> 7 Your spin 3 3 3 You matched reels You won/lost 63 Current bank 70 Finishing the program. You will have to modify the main program in order to finish, but most of the work is done and you can see how functions make the main program much smaller, more compact, easier to com- prehend and to debug get_slot_results() -> tuple: function Returns 3 random reels at once. It returns them as a tuple. No- you can return 3 integers all In the same return separated by a comma. Notice in the main program we can assign them to 3 variables when the function is called. Each reel is a ran- dom number between 1 and 10 inclusive. How many chips do you want to wager? --> 70 Your spin 613 You matched o reels You won/lost -70 Current bank @ You lost all 10 in 4 spins The most chips you had was 70 Do you want to play again? --> e get_matches(reela, reelb, reelc) -> int: function This function returns 3 if all 3 reels match, 2 if there are 2 matching reels and otherwise. Example How many chips do you want to start with? --> -1 Too low a value, you can only choose 1 - 100 chips How many chips do you want to start with? --> 500 Too high a value, you can only choose 1 - 100 chips How many chips do you want to start with? --> 10 How many chips do you want to wager? --> -1 The wager amount must be greater than e. Please enter again. How many chips do you want to wager? --> 12 The wager amount cannot be greater than how much you have. 10 How many chips do you want to wager? --> 5 Your spin 4 29 You must enter Y/YES/N/ND to continue. Please try again Do you want to play again? ==> n Grading and Turning InStep by Step Solution
There are 3 Steps involved in it
Step: 1
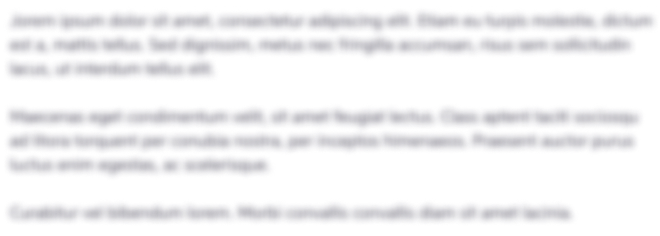
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started