Question
So I being working on a program for my c++ class and am getting a couple errors when i debugg and I have become very
So I being working on a program for my c++ class and am getting a couple errors when i debugg and I have become very frustrated trying to solve it. i get a error R6010 and it calls to abort. the program is trying to translate file from infix to postfix. using stacks.
#pragma once
//'* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
//'* Program name : myStack.h *
//'* *
#ifndef H_mystack
#define H_mystack
#include
#include
using namespace std;
//template
//class stackADT
//{
//public:
// virtual void initializeStack() = 0;
// //Method to initialize the stack to an empty state.
// //Postcondition: Stack is empty
//
// virtual bool isEmptyStack() const = 0;
// //Function to determine whether the stack is empty.
// //Postcondition: Returns true if the stack is empty,
// /* otherwise returns false.*/
//
// virtual bool isFullStack() const = 0;
// //Function to determine whether the stack is full.
// //Postcondition: Returns true if the stack is full,
// /* otherwise returns false.*/
//
// virtual void push(const Type& newItem) = 0;
// //Function to add newItem to the stack.
// //Precondition: The stack exists and is not full.
// //Postcondition: The stack is changed and newItem
// /* is added to the top of the stack.
//*/
// virtual Type top() const = 0;
// /*Function to return the top element of the stack.
// Precondition: The stack exists and is not empty.
// Postcondition: If the stack is empty, the program
// terminates; otherwise, the top element
// of the stack is returned.*/
//
// virtual void pop() = 0;
// /*Function to remove the top element of the stack.
// Precondition: The stack exists and is not empty.
// Postcondition: The stack is changed and the top
// element is removed from the stack.*/
//};
template
class MyStack /*: public stackADT*/
{
public:
void initializeStack();
//Function to initialize the stack to an empty state.
//Postcondition: stackTop = 0
bool isEmptyStack() const;
//Function to determine whether the stack is empty.
//Postcondition: Returns true if the stack is empty,
// otherwise returns false.
bool isFullStack() const;
//Function to determine whether the stack is full.
//Postcondition: Returns true if the stack is full,
// otherwise returns false.
void push(const Type& );
//Function to add newItem to the stack.
//Precondition: The stack exists and is not full.
//Postcondition: The stack is changed and newItem
// is added to the top of the stack.
Type top() const;
//Function to return the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: If the stack is empty, the program
// terminates; otherwise, the top element
// of the stack is returned.
void pop();
//Function to remove the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: The stack is changed and the top
// element is removed from the stack.
MyStack(int = 20);
//constructor
//Create an array of the size stackSize to hold
//the stack elements. The default stack size is 100.
//Postcondition: The variable list contains the base
// address of the array, stackTop = 0, and
// maxStackSize = stackSize.
~MyStack();
//destructor
//Remove all the elements from the stack.
//Postcondition: The array (list) holding the stack
// elements is deleted.
private:
int maxStackSize; //variable to store the maximum stack size
int stackTop; //variable to point to the top of the stack
Type *list; //pointer to the array that holds the
//stack elements
};
template
void MyStack
{
stackTop = 0;
}//end initializeStack
template
bool MyStack
{
return(stackTop == 0);
}//end isEmptyStack
template
bool MyStack
{
return(stackTop == maxStackSize);
} //end isFullStack
template
void MyStack
{
if (!isFullStack())
{
list[stackTop] = newItem; //add newItem to the
//top of the stack
stackTop++; //increment stackTop
}
else
cout << "Cannot add to a full stack." << endl;
}//end push
template
Type MyStack
{
assert(stackTop != 0); //if stack is empty,
//terminate the program
return list[stackTop - 1]; //return the element of the
//stack indicated by
//stackTop - 1
}//end top
template
void MyStack
{
if (!isEmptyStack())
stackTop--; //decrement stackTop
else
cout << "Cannot remove from an empty stack." << endl;
}//end pop
template
MyStack
{
if (stackSize <= 0)
{
cout << "Size of the array to hold the stack must "
<< "be positive." << endl;
cout << "Creating an array of size 100." << endl;
maxStackSize = 100;
}
else
maxStackSize = stackSize; //set the stack size to
//the value specified by
//the parameter stackSize
stackTop = 0; //set stackTop to 0
list = new Type[maxStackSize]; //create the array to
//hold the stack elements
}//end constructor
template
MyStack
{
delete[] list; //deallocate the memory occupied
//by the array
}//end destructor
#endif
#pragma once
//'* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
//'* Program name : myStack.h *
//'* *
#ifndef H_mystack
#define H_mystack
#include
#include
using namespace std;
//template
//class stackADT
//{
//public:
// virtual void initializeStack() = 0;
// //Method to initialize the stack to an empty state.
// //Postcondition: Stack is empty
//
// virtual bool isEmptyStack() const = 0;
// //Function to determine whether the stack is empty.
// //Postcondition: Returns true if the stack is empty,
// /* otherwise returns false.*/
//
// virtual bool isFullStack() const = 0;
// //Function to determine whether the stack is full.
// //Postcondition: Returns true if the stack is full,
// /* otherwise returns false.*/
//
// virtual void push(const Type& newItem) = 0;
// //Function to add newItem to the stack.
// //Precondition: The stack exists and is not full.
// //Postcondition: The stack is changed and newItem
// /* is added to the top of the stack.
//*/
// virtual Type top() const = 0;
// /*Function to return the top element of the stack.
// Precondition: The stack exists and is not empty.
// Postcondition: If the stack is empty, the program
// terminates; otherwise, the top element
// of the stack is returned.*/
//
// virtual void pop() = 0;
// /*Function to remove the top element of the stack.
// Precondition: The stack exists and is not empty.
// Postcondition: The stack is changed and the top
// element is removed from the stack.*/
//};
template
class MyStack /*: public stackADT*/
{
public:
void initializeStack();
//Function to initialize the stack to an empty state.
//Postcondition: stackTop = 0
bool isEmptyStack() const;
//Function to determine whether the stack is empty.
//Postcondition: Returns true if the stack is empty,
// otherwise returns false.
bool isFullStack() const;
//Function to determine whether the stack is full.
//Postcondition: Returns true if the stack is full,
// otherwise returns false.
void push(const Type& );
//Function to add newItem to the stack.
//Precondition: The stack exists and is not full.
//Postcondition: The stack is changed and newItem
// is added to the top of the stack.
Type top() const;
//Function to return the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: If the stack is empty, the program
// terminates; otherwise, the top element
// of the stack is returned.
void pop();
//Function to remove the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: The stack is changed and the top
// element is removed from the stack.
MyStack(int = 20);
//constructor
//Create an array of the size stackSize to hold
//the stack elements. The default stack size is 100.
//Postcondition: The variable list contains the base
// address of the array, stackTop = 0, and
// maxStackSize = stackSize.
~MyStack();
//destructor
//Remove all the elements from the stack.
//Postcondition: The array (list) holding the stack
// elements is deleted.
private:
int maxStackSize; //variable to store the maximum stack size
int stackTop; //variable to point to the top of the stack
Type *list; //pointer to the array that holds the
//stack elements
};
template
void MyStack
{
stackTop = 0;
}//end initializeStack
template
bool MyStack
{
return(stackTop == 0);
}//end isEmptyStack
template
bool MyStack
{
return(stackTop == maxStackSize);
} //end isFullStack
template
void MyStack
{
if (!isFullStack())
{
list[stackTop] = newItem; //add newItem to the
//top of the stack
stackTop++; //increment stackTop
}
else
cout << "Cannot add to a full stack." << endl;
}//end push
template
Type MyStack
{
assert(stackTop != 0); //if stack is empty,
//terminate the program
return list[stackTop - 1]; //return the element of the
//stack indicated by
//stackTop - 1
}//end top
template
void MyStack
{
if (!isEmptyStack())
stackTop--; //decrement stackTop
else
cout << "Cannot remove from an empty stack." << endl;
}//end pop
template
MyStack
{
if (stackSize <= 0)
{
cout << "Size of the array to hold the stack must "
<< "be positive." << endl;
cout << "Creating an array of size 100." << endl;
maxStackSize = 100;
}
else
maxStackSize = stackSize; //set the stack size to
//the value specified by
//the parameter stackSize
stackTop = 0; //set stackTop to 0
list = new Type[maxStackSize]; //create the array to
//hold the stack elements
}//end constructor
template
MyStack
{
delete[] list; //deallocate the memory occupied
//by the array
}//end destructor
#endif
#pragma once
//'* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
//'* Program name : infixToPostfix.h *
//'*
#ifndef H_infixToPostfix
#define H_infixToPostfix
#include
#include "myStack.h"
#include
#include
using namespace std;
class InfixPostfix
{
public:
bool precedence(char oper1, char oper2);
bool isitOperator(const char c);
void getInFix(string infixinputString)
{
infix = infixinputString;
}
void showPostFix();
void showInFix()
{
cout << "The infix Expression is: " << infix < } private: string infix; string postfix; }; bool InfixPostfix::isitOperator(const char c) // test to see if it is a operator { if ((c == '+') || (c == '-') || (c == '*') || (c == '/') || (c == '^') || (c == '%')) { return true; } else { return false; } } bool InfixPostfix::precedence(char oper1, char oper2) // checks precedence { int pres1, pres2; switch(oper1) { case '^': cout<<"exponent can be considered for precedence the program will result in wierd ouput." << endl; break; case '*': pres1 =3; break; case '/': pres1 = 2; break; case '+': pres1 = 1; break; case '-': pres1 = 0; break; } switch (oper2) { case '^': cout<<"exponent can be considered for precedence the program will result in wierd ouput." << endl; break; case '*': pres2 =3; break; case '/': pres2 = 2; break; case '+': pres2 = 1; break; case '-': pres2 = 0; break; } if (pres1 == pres2) //eqaul precedence of oper1 and oper2 { return 0; } else if (pres1 > pres2) //oper1 is higher than oper2 { return 1; } else if (pres1 < pres2) //oper1 is less than oper2 { return 0; } else { return 0; } } void InfixPostfix::showPostFix() { MyStack string postfix = ""; infix.append(")"); PostStack.push('('); int z = 0; do { if (isitOperator(infix[z]))//a { if (isitOperator(PostStack.top()))//b { if (precedence(infix[z], PostStack.top()) == 0)//c finds precedence of the operator { postfix = postfix + PostStack.top(); PostStack.pop(); } else if (precedence(infix[z], PostStack.top()) == 1)//pushs stack when higher prcense occurs { PostStack.push(infix[z]); z++; }// end of c } else // psuhes stack if the top of stack is not operator { postfix = postfix + PostStack.top(); PostStack.pop(); }// end of b } else if (infix[z] == ')') { while (PostStack.top() != '(') { postfix = postfix + PostStack.top(); PostStack.pop(); } PostStack.pop(); z++; } else { if (infix[z] == '(') { PostStack.push(infix[z]); z++; } else { postfix = postfix + infix[z]; z++; } }//end of a } while (z != infix.length() ); // while z is less than the length of the infix the program will continue above cout << "The postfix expresion is: " << postfix; // prints the postfix expression } #endif main// #include #include #include #include "infixToPostfix.h" #include "myStack.h" using namespace std; int main() { InfixPostfix InfixExp; string infix; ifstream infile; infile.open("G:\\New folder\\Project1\\Debug\\infixData.txt"); if (!infile) { cout << "Cannot open input file. Program termination initiated." << endl; system("PAUSE"); return 1; } getline(infile, infix); while (infile) { InfixExp.getInFix(infix); InfixExp.showInFix(); InfixExp.showPostFix(); cout << endl; getline(infile, infix); } infile.close(); system("PAUSE"); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
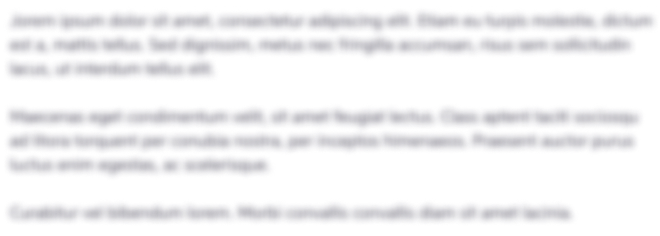
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started