Question
Solution should be in Python Program implementation Implement a Python program that has a method that returns a dictionary of parts that had over-voltage in
Solution should be in Python Program implementation
Implement a Python program that has a method that returns a dictionary of parts that had over-voltage in two or more test runs.
Precondition: The "runData" parameter contains all the data. That's a dictionary of test runs. Each entry contains LotID as a key, and a List of Part objects. The Part class is defined as below: class Part:
def __init__(self, serialNumber:str) -> None:
self.serialNumber:str = serialNumber
self.readings:List[float] = [0] * 3
Postcondition: returns a dictionary of parts who had over-voltages in 2 or more test runs. The key of each entry is partName, and the value is a list of failed test runs. To pass a test, the average voltages must be greater than 5.0V. For example {PartCC: 5.5, 6.8, 4.8} failed Lot123 because its average score is 5.
def getBadParts (runData : Dict[str, List[Part]]) -> Dict[str, List[str]]:
Example, if runData parameter has the following data
Key (lotID) Value (List of Part objects)
Lot123 {PartAA: [3.5, 3.8, 3.9]}, {PartBB: [4.5, 4.2, 4.9]}, {PartCC: [5.5, 6.8, 4.8]}
Lot124 {PartAA: [2.5, 3.8, 3.9]}, {PartBB: [1.8, 3.9, 4.9]}, {PartDD: [7.2, 4.3, 6.4]},{PartEE: [4.4, 8.5, 8.5]}
Lot125 {PartAA: [3.8, 3.8, 3.9]}, {PartCC: [7.4, 4.5, 8.6]}, {PartDD: [5.0, 6.3,4.1]},{PartEE: [7.3, 4.2, 6.5]}
Lot126 {PartAA: [3.7, 3.8, 3.9]}, {PartBB: [3.9, 1.9, 5.9,]}, {PartFF: [3,2,2]},{PartEE: [9.0, 3.4, 9.5]}
Then, the getBadParts() will return the following data.
Key Value
PartCC {Lot123, Lot125}
PartDD {Lot124, Lot125}
PartEE {Lot124, Lot125, Lot126}
2. In competitive diving, each diver makes a dive of varying degrees of difficulty. Nine judges score each dive from 0 through 10 in steps of 0.5. The total score is obtained by discarding the lowest and highest of the judges' scores, adding the remaining scores, and then multiplying the scores by the degree of difficulty. The degree of difficulty ranges from 1.2 to 3.6. Write a Python program which reads the data file named "data.txt", processes the data and writes a summary in the output file named "final.txt".
Define a class named Diver. Each dive has 9 scores and one difficulty. Write a method named "readFileToList" to read the data file and add the data to the ArrayList of diver objects. def readFileToList( filename, diverList: Diver) -> None: Write a method named "writeListToFile" to write the final scores to the output file. def writeListToFile( filename:str, diverList: Diver) -> None: Calculate each diver's final score using the grading guidelines outlined above. Write a main method to test the above two methods.
Sample input file: data.txt (format: Divers name, difficulty, score1, score2, score3, score4, score5, score6, score7, score8, score9)
Peter, 2.5, 10.0, 10.0, 10.0, 10.0, 10.0, 10.0, 10.0, 10.0, 10.0
David, 3.5, 9.0, 8.0, 7.0, 8.0, 8.0, 5.0, 8.0, 9.5, 5.5
Judy, 2.5, 9.5, 8.0, 8.5, 8.0,7.0, 5.5, 8.5, 9.5, 10.0
Output file: finals.txt
Peter 175.0
David 185.5
Judy 147.5
Step by Step Solution
There are 3 Steps involved in it
Step: 1
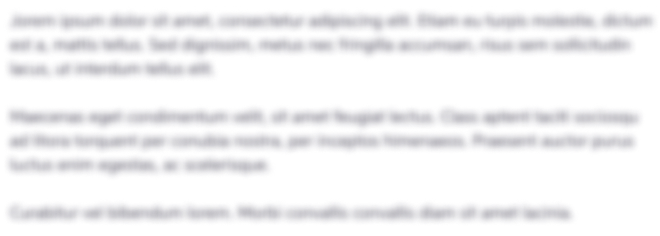
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started