Answered step by step
Verified Expert Solution
Question
1 Approved Answer
solve problems of LinkedList.java, problems are in comments of LinkedList.java Please showing the output There are some tested method in LinkedListTest.java (Please don't modify Account.java
solve problems of "LinkedList.java", problems are in comments of "LinkedList.java" Please showing the output
There are some tested method in "LinkedListTest.java"
(Please don't modify "Account.java" and "Main.java", after solve problems of "LinkedList.java", please open three java documents altogether and run with "Main.java" Please don't write other 4th extra java document Thank you )
"LinkedList.java"
public class LinkedList { // Definition of the Node class that LinkedList internally uses class Node { T item; Node next; public Node() { } public Node(T item) { this.item = item; } } private Node head; private Node tail; // maintain track of array size private int size = 0; public void push(T item) { // Problem: complete "push" method // Problem: need consider 2 cases. When list is empty, when list is not empty // Problem: increment size } public T get(int i) throws ListIndexOutOfBoundException { rangeCheck(i); // throws exception but won't catch it here // Problem: complete rest of method // should return the item that stored in the node, instead of null return null; } public int find(T item) { // Problem: complete "find" method return -1; } public void insert(int i, T item) throws ListIndexOutOfBoundException { // Problem: Complete "insert" method /* * There 3 cases: * ---1. list is empty (head is null) * ---2. inserting at the beginning of the list (i = 0) * ---3. inserting anywhere else * * new node would inserted on index and push the current node to right. e.g. * [ac1][ac2][ac3] * insert(1, ac1.5) * [ac1][ac1.5][ac2][ac3] * insert(3, ac2.5) * [ac1][ac1.5][ac2][ac2.5][ac3] */ } public int size() { return size; } public T delete(int i) throws ListIndexOutOfBoundException, EmptyListException { // Problem: complete "delete" method // Problem: apply this method to store item, then return it in the end T deletedItem; /* * similar with insert, 3 cases must consider * empty list, insert at the beginning, and anywhere else * decrement size * * * Remind: use cursor.next = cursor.next.next */ return deletedItem; } // The Utility methods public void rangeCheck(int i) throws ListIndexOutOfBoundException { if (i < 0 || i >= size) throw new ListIndexOutOfBoundException(); } @Override public String toString() { StringBuilder sb = new StringBuilder(); Node cur = head; sb.append("["); while (cur != null) { if (cur.next == null) { sb.append(cur.item.toString()); } else { sb.append(cur.item.toString()); sb.append(", "); } cur = cur.next; } sb.append("]"); return sb.toString(); } } class ListIndexOutOfBoundException extends Exception { } class EmptyListException extends Exception { }
"Main.java"
public class Main { public static void main(String[] args) throws ListIndexOutOfBoundException, EmptyListException { LinkedList accounts = new LinkedList<>(); accounts.push(new Account("user1", "1234")); accounts.push(new Account("user2", "abcd")); accounts.push(new Account("user3", "eeee")); accounts.push(new Account("user4", "aaaa")); System.out.println(accounts); System.out.println(accounts.get(1)); Account user1 = new Account("user1.5", "abcdefg"); accounts.insert(3, user1); System.out.println(accounts); System.out.println(accounts.find(user1)); accounts.delete(1); System.out.println(accounts); System.out.println(accounts.size()); } }
"Account.java"
public class Account { private String userName; private String password; public Account(String userName, String password) { this.userName = userName; this.setPassword(password); } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } // @Override is a Java annotation that hints the compiler that we are overriding // an inherited method @Override public boolean equals(Object user) { Account otherUser = (Account) user; return this.getUserName().equals(otherUser.getUserName()) && this.getPassword().equals(otherUser.getPassword()); } @Override public String toString() { return String.format("{username: %s, password: %s}", this.getUserName(), this.getPassword()); } }
'LinkedListTest.java"
import static org.junit.jupiter.api.Assertions.assertEquals; import static org.junit.jupiter.api.Assertions.assertThrows; import org.junit.jupiter.api.BeforeEach; import org.junit.jupiter.api.Test; public class LinkedListTest { private LinkedList accounts; @BeforeEach public void init() { accounts = new LinkedList<>(); } @Test public void testGet() throws ListIndexOutOfBoundException { Account u1 = new Account("user1", "1234"); Account u2 = new Account("user2", "abcd"); Account u3 = new Account("user3", "eeee"); assertThrows(ListIndexOutOfBoundException.class, () -> accounts.get(0)); accounts.push(u1); accounts.push(u2); accounts.push(u3); assertEquals(u2, accounts.get(1)); } @Test public void testPush() throws ListIndexOutOfBoundException { accounts.push(new Account("user1", "1234")); accounts.push(new Account("user2", "abcd")); accounts.push(new Account("user3", "eeee")); assertEquals(3, accounts.size()); assertEquals("user3", accounts.get(2).getUserName()); } @Test public void testInsert() throws ListIndexOutOfBoundException { accounts.insert(0, new Account("user1", "1234")); assertEquals(1, accounts.size()); assertEquals("user1", accounts.get(0).getUserName()); assertThrows(ListIndexOutOfBoundException.class, () -> accounts.insert(10, null)); Account u3 = new Account("user3", "abcdefg"); accounts.push(u3); Account u2 = new Account("user2", "abcd"); accounts.insert(1, u2); assertEquals(u2, accounts.get(1)); assertEquals(3, accounts.size()); Account u4 = new Account("user4", "fffff"); accounts.insert(accounts.size() - 1, u4); assertEquals(u4, accounts.get(2)); assertEquals(u3, accounts.get(3)); } @Test public void testFind() { accounts.push(new Account("user1", "1234")); accounts.push(new Account("user2", "abcd")); accounts.push(new Account("user3", "eeee")); assertEquals(1, accounts.find(new Account("user2", "abcd"))); assertEquals(-1, accounts.find(new Account("user6", "abcd"))); } @Test public void testDelete() throws ListIndexOutOfBoundException, EmptyListException { Account u1 = new Account("user1", "1234"); Account u2 = new Account("user2", "abcd"); Account u3 = new Account("user3", "eeee"); accounts.push(u1); accounts.push(u2); accounts.push(u3); accounts.delete(1); assertEquals(2, accounts.size()); assertEquals(0, accounts.find(u1)); assertEquals(1, accounts.find(u3)); assertEquals(-1, accounts.find(u2)); accounts.delete(0); accounts.delete(0); assertEquals(0, accounts.size()); accounts.push(u1); accounts.push(u2); assertEquals(2, accounts.size()); assertEquals(1, accounts.find(u2)); assertEquals(0, accounts.find(u1)); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Lets address the problems in the LinkedListjava file java public class LinkedList Add generic type t...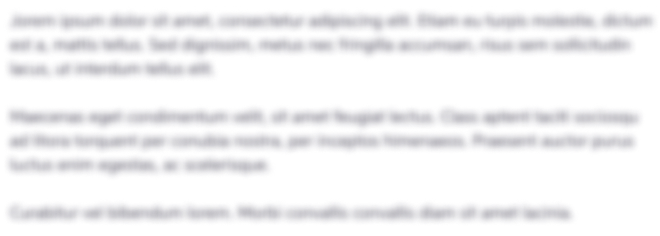
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started