Question
Some information on the Internet may be encrypted with a simple algorithm known as rot13 , which rotates each character by 13 positions in the
Some information on the Internet may be encrypted with a simple algorithm known as rot13, which rotates each character by 13 positions in the alphabet. A rot13 cryptogram is a message or word in which each letter is replaced with another letter that comes from a position in the alphabet moved from the original letter position at a constant displacement value, e.g. 13 letters forward.
- rot13 is an example of symmetric key encryption.
For example, the string
the bird was named squawk
might be scrambled to form
cin vrjs otz ethns zxqtop
Details
-
Write missing method implementations for the class Rot13 that get a functional ROT13 cipher.
-
The input plain or encrypted message string is placed into the Rot13 object during the initialization.
- The input string may only contain Latin lowercase letters and spaces. You must check if the input string is valid before storing the input in the text field. If the input is invalid, the text field must be left empty.
-
Overload the bang operator ! to check if the Rot13 object is correctly initialized, and contains a non-empty message.
- The operator returns true if the message is empty.
-
Overload the stream extraction operator >> for the Rot13 class to perform rot13 encryption.
- The operator has to be implemented as a member function with a (modifiable) string reference parameter which is used to store an encrypted message.
- Encrypt the message stored in the string field text, and place it in the method reference parameter.
- The encryption procedure replaces every occurrence of the character with the character 13 steps forward in the alphabet (in a circular way). For example, a with n , b with o , and x with k, and so on.
- Spaces are not scrambled.
- Use static_cast<> operator to transform characters into numbers which would allow to perform rotation transformation using addition/subtraction operations.
- You can find numerical codes for letters in ASCII Table
-
Overload the insertion operator << for the Rot13 class to perform decryption of the ROT13 cipher into the original message.
- Because ROT13 is a symmetric key encryption, the decryption process is a exact reversal of the encryption. The decryption replaces every occurrence of the character with the character 13 steps backward in the alphabet (in a circular way).
- Spaces are not scrambled.
-
You can add any number of additional methods into the class for better code reuse.
-
All method implementations must be outside the class declaration.
// Implement the class methods
class Rot13 {
const int rotation{13};
std::string text;
public:
Rot13(std::string msg = "");
bool operator!();
void operator>>(std::string&);
friend void operator<<(std::string&, Rot13&);
};
//------------------------------
// DO NOT MODIFY TEST CASES
//------------------------------
TEST_CASE( "Assignment" ) {
SECTION( "v1" ) {
Rot13 cipher;
REQUIRE( !cipher );
}
SECTION( "v2" ) {
Rot13 cipher{"ABCDEFGHIJKLMNOPQRSTUVWXYZ"};
REQUIRE( !cipher );
}
SECTION( "v3" ) {
Rot13 cipher{"slkgjskjg akjf Adkfjd fsdfj"};
REQUIRE( !cipher );
}
SECTION( "v4" ) {
Rot13 cipher{"abcdefghijkl mnopqrst uvwxyz"};
REQUIRE( !!cipher );
}
SECTION( "e1" ) {
Rot13 cipher{"abcdefghijkl mnopqrst uvwxyz"};
REQUIRE( !!cipher );
std::string secret;
cipher >> secret;
REQUIRE( secret == "nopqrstuvwxy zabcdefg hijklm" );
}
SECTION( "e2" ) {
Rot13 cipher{"nopqrstuvwxy zabcdefg hijklm"};
REQUIRE( !!cipher );
std::string msg;
msg << cipher;
REQUIRE( msg == "abcdefghijkl mnopqrst uvwxyz" );
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
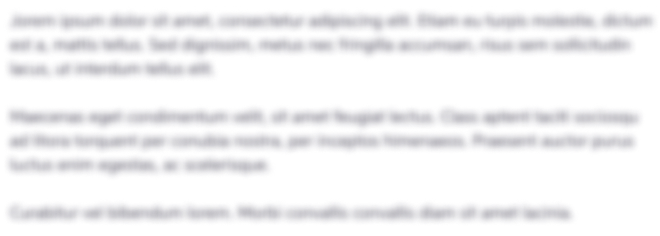
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started