Question
Starting out with JAVA from control structures through objects 6th edition Tony Gaddis Programming Challenge Chapter 10 Challenge 9: QUESTION: Re-write the challenge using your
Starting out with JAVA from control structures through objects 6th edition Tony Gaddis
Programming Challenge Chapter 10 Challenge 9:
QUESTION: Re-write the challenge using your code from last week and mark the monthlyProcess method abstract in the BankAccount class.
Hint: What else will you have to change? <-
Basically last week we made this code not abstract and now we must make it abstract in the places it states above. please help thank you very much may you please put comments where you add stuff so i can understand as well thanks alot.
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
Code i made from last week to be used to answer question above.<<<< ----
First class BankAccount Class vvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvv
public class BankAccount {
private int no_of_deposits, no_of_withdrawls; /* variables set that will be used to hold the number of deposits and withdrawls throughout the program*/
private double balance,annual_interest_rate,service_charges; /* variables set, balance variable will hold total amount in the balance, annual interest rate will hold the percentage interest rate that will be used, and service charges variables will hold the amount charged monthly*/
/** two-arg constructor */
public BankAccount(double newbalance,double newinterest) {
this.balance = newbalance;
this.annual_interest_rate = newinterest;
}
/** accessor for private data member balance */
public double getBalance() {
return balance; //returns balance after accessing private data from other class to get the balance
}
/** accessor for private data member no_of_withdrawls */
public int getNo_of_withdrawls() {
return no_of_withdrawls; //returns number of withdrawls after accessing private data from other class to get the the number
}
/** mutator for private data member service_charges */
public void setService_charges(double service_charges) {
this.service_charges = service_charges;
}
/** Method to change balance via a withdrawal */
public void withdraw(double amount) {
balance -= amount; // Subtract amount from balance
no_of_withdrawls++; // increase count
}
/** Method to change balance via a deposit */
public void deposit(double amount) {
balance += amount; // add amount to balance
no_of_deposits++; // increase count
}
/** Method to change calculate interest and it to balance */
public void calcInterest() {
double monthly_interest_rate = annual_interest_rate/12; // calculates monthly interest rate by dividing annual byAmountOfMonths
double monthly_interest = monthly_interest_rate * balance; //calculating month interest rate to the balance
balance = balance + monthly_interest; //adding the monthly interest rate calculated to the balance
}
/** Method to process monthly charges and interests*/
public void monthlyProcess() {
balance = balance - service_charges; // subtract charges from balance
calcInterest(); // call to interest method calcInterest
no_of_deposits = 0; //setting number of deposits to 0 to start because at the beginning their are none
no_of_withdrawls = 0; //same for withdrawls ^^
service_charges = 0; //same for service charges start at 0 because when you start an account you have no service charges yet
} public static void main(String[] args) { //static main method to demonstrate the way it works
BankAccount savings1 = new BankAccount(100, 15.0);
BankAccount savings2 = new BankAccount(20, 15.0);
savings1.withdraw(10);
savings2.withdraw(10);
System.out.println(savings1.getBalance()); /*sends the numbers through the private accessor of the other class SavingsAccount and brings back the number after going through the process of getBalance there then prints it */
System.out.println(savings2.getBalance()); // same as above but for savings2
savings2.deposit(4);
System.out.println(savings2.getBalance());
System.out.println(savings2.getNo_of_withdrawls()); //calcs number of withdrawls and displays
savings2.deposit(15);
savings2.withdraw(1);
savings2.withdraw(1);
savings2.withdraw(1);
savings2.withdraw(1);
savings2.withdraw(1);
savings2.withdraw(5);
System.out.println(savings2.getBalance());
System.out.println("No Of withdrawls before monthly process "+savings2.getNo_of_withdrawls());
savings2.monthlyProcess(); //displays number of withdrawls before the monthly process
System.out.println("No Of withdrawls after monthly process "+savings2.getNo_of_withdrawls()); //displays the number after
System.out.println("Balance after monthly process "+savings2.getBalance()); //displays the final balance after process
}
}
//////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
Second Class SavingsAccount vvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvv
public class SavingsAccount extends BankAccount {
private boolean IsInactive = false; /* determines this boolean variable is_inactive, it will be used to hold true or false for if the account is active or inactive*/
/** two-arg constructor */
public SavingsAccount(double newbalance, double newinterest) {
super(newbalance, newinterest);
if (newbalance < 25) { // verify the balance and set the status flag of $25
IsInactive = true;
}
}
@Override
public void withdraw(double amount) {
if (!IsInactive) { // check status flag before calling withdraw method
super.withdraw(amount);
if ( getBalance() < 25) { // check balance after withdraw and set the status flag
IsInactive = true;
}
} else {
System.out.println("Account is inActive, please maintain above 25 balance"); //printed if less than $25 dollars in account
}
}
@Override
public void deposit(double amount) {
if (!IsInactive) { // check status flag before calling deposit method
super.deposit(amount);
} else if (getBalance() + amount >= 25) { // check status flag before calling deposit method including both balance and deposited amount
super.deposit(amount);
IsInactive = false;
} else {
System.out.println("Account is Still inactive with deposited amount"); //if still under $25 this displays
}
}
@Override
public void monthlyProcess() {
int no_of_withdrawls = getNo_of_withdrawls(); // get number of withdrawals performed
if (no_of_withdrawls > 4) { // check if number of withdrawals is more than 4
setService_charges(no_of_withdrawls - 4); // if number of withdrawals more than 4 then set service charges 1 dollar per 1 transaction excluding first 4
}
super.monthlyProcess();
if ( getBalance() < 25) { // if the balance < 25 after monthly process, set the status flag to true
IsInactive = true;
}
} public static void main(String[] args) { //static main methood to demonstrate the way it works
SavingsAccount savings1 = new SavingsAccount(100, 15.0);
SavingsAccount savings2 = new SavingsAccount(20, 0.0);
savings1.withdraw(10);
savings2.withdraw(10);
System.out.println(savings1.getBalance());
System.out.println(savings2.getBalance());
savings2.deposit(4);
System.out.println(savings2.getBalance());
System.out.println(savings2.getNo_of_withdrawls());
savings2.deposit(15);
savings2.withdraw(1);
savings2.withdraw(1);
savings2.withdraw(1);
savings2.withdraw(1);
savings2.withdraw(1);
savings2.withdraw(5);
System.out.println(savings2.getBalance());
System.out.println("No Of withdrawls before monthly process "+savings2.getNo_of_withdrawls());
savings2.monthlyProcess(); //displays number of withdrawls before monthly process
System.out.println("No Of withdrawls after monthly process "+savings2.getNo_of_withdrawls()); //displays the after
System.out.println("Balance after monthly process "+savings2.getBalance()); //displays the balance after monthly process
System.out.println(savings2.IsInactive); //displays true or false for if the account is active or inactive
}
}
///////////////////////////////////////////////////////////////////////////////////////////////////////////////
Step by Step Solution
There are 3 Steps involved in it
Step: 1
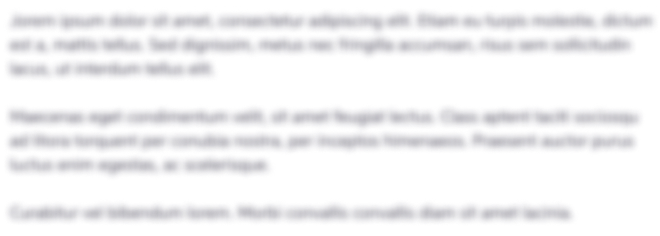
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started