Swift - iOS Xcode 9.4 Write a function that: - takes in an array of integers and separates each element of the array into odd
Swift - iOS Xcode 9.4
Write a function that:
- takes in an array of integers and separates each element of the array into odd and even numbers
- then splits the array into lists that are sorted in DESCENDING order (largest first, smallest last)
- finally, your function should return the sorted evens and odds as a tuple
- Each element in the array COULD be nil. So you will have to figure out how to represent that in the parameter's type.
- If an element in the array is nil, do nothing with it.
- Don't forget edge cases like an empty data or all nil values.
Notes:
- Research how to return multiple values (as a named tuple) from Swift functions
- DO NOT manually sort the integers; find a better way using Swift's libraries!
- Your function MUST work with the commented out function calls below!
//let sortedNumbers = sortedEvenOdd(numbers: [0, 9, 3, nil, 8, 15, 11, 2, 20, nil])
//print(sortedNumbers.evens) // [20, 8, 2, 0]
//print(sortedNumbers.odds) // [15, 11, 9, 3]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
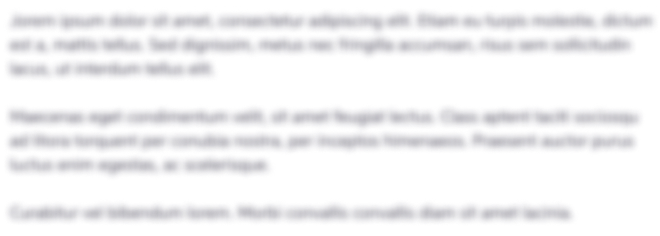
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started