Question
take the input from a file (Part 1) and send the output to a file (Part 2), merge the two programs so that the program
take the input from a file (Part 1) and send the output to a file (Part 2), merge the two programs so that the program operates in "batch mode". The program should run with no interaction from the user. When executed it should open a file for input and use that file to create an output file with the results.
PART 1
#include
using namespace std;
int main() { // Input variables float loanAmt; // Amount of loan float yearlyInt; // Yearly interest int numYears; // Number of years
// Local variables float monthlyInterest; // Monthly interest rate int numberOfPayments; // Total number of payments float payment; // Monthly payment ofstream outData; // Link to output file string outFileName; // Output file name
// Prompt user for output file name cout << "Enter name for output file: "; cin >> outFileName;
// Open output file outData.open(outFileName.c_str());
// Prompt user for loanAmt, yearlyIn, numYears (Enter 0's to quit) cout << "Enter three values: " << " Loan amount, Yearly interest, and Number of years " << " Separate by spaces (Enter all 0's to quit): "; cin >> loanAmt >> yearlyInt >> numYears; cout << endl << endl;
while (loanAmt != 0){
// Calculate values monthlyInterest = yearlyInt / 12; numberOfPayments = numYears * 12; payment = (loanAmt * pow(monthlyInterest + 1, numberOfPayments) * monthlyInterest) / (pow(monthlyInterest + 1, numberOfPayments) - 1);
// Send output to file outData << fixed << setprecision(2) << "Loan amount: $" << loanAmt << endl; outData << fixed << setprecision(2) << "Interest rate: " << yearlyInt << endl; outData << "Years: " << numYears << endl << endl;
outData << "Your monthly payments are $" << payment << endl << endl << endl;
// Send output to screen cout << fixed << setprecision(2) << "Loan amount: $" << loanAmt << endl; cout << fixed << setprecision(2) << "Interest rate: " << yearlyInt << endl; cout << "Years: " << numYears << endl << endl;
cout << "Your monthly payments are $" << payment << endl << endl << endl;
// Prompt user for loanAmt, yearlyIn, numYears cout << "Enter the loan amount, yearly interest, and number of years separated by spaces (0's to quit): "; cin >> loanAmt >> yearlyInt >> numYears; cout << endl << endl; }
ifstream inData; // Link to input file string inFileName; // Input file name
// Prompt user for input file name cout << "Enter name for input file: "; cin >> inFileName;
// Open input file inData.open(inFileName.c_str());
// Get first line of data from file inData >> loanAmt >> yearlyInt >> numYears;
// Loop until 0's read from file while (loanAmt != 0){
// Calculate values monthlyInterest = yearlyInt / 12; numberOfPayments = numYears * 12; payment = (loanAmt * pow(monthlyInterest + 1, numberOfPayments) * monthlyInterest)/(pow(monthlyInterest + 1, numberOfPayments) - 1);
// Send output to screen cout << fixed << setprecision(2) << "Loan amount: $" << loanAmt << endl; cout << fixed << setprecision(2) << "Interest rate: " << yearlyInt << endl; cout << "Years: " << numYears << endl << endl;
cout << "Your monthly payments are $" << payment << endl << endl << endl;
// Get next line of data from file inData >> loanAmt >> yearlyInt >> numYears;
} // Close file outData.close();
cout << "Good bye!" << endl;
return 0; }
PART 2
#include
using namespace std;
int main() { // Input variables float loanAmt; // Amount of loan float yearlyInt; // Yearly interest int numYears; // Number of years
// Local variables float monthlyInterest; // Monthly interest rate int numberOfPayments; // Total number of payments float payment; // Monthly payment ifstream inData; // Link to input file string inFileName; // Input file name
// Prompt user for input file name cout << "Enter name for input file: "; cin >> inFileName;
// Open input file inData.open(inFileName.c_str());
// Get first line of data from file inData >> loanAmt >> yearlyInt >> numYears;
// Loop until 0's read from file while (loanAmt != 0){
// Calculate values monthlyInterest = yearlyInt / 12; numberOfPayments = numYears * 12; payment = (loanAmt * pow(monthlyInterest + 1, numberOfPayments) * monthlyInterest)/(pow(monthlyInterest + 1, numberOfPayments) - 1);
// Send output to screen cout << fixed << setprecision(2) << "Loan amount: $" << loanAmt << endl; cout << fixed << setprecision(2) << "Interest rate: " << yearlyInt << endl; cout << "Years: " << numYears << endl << endl;
cout << "Your monthly payments are $" << payment << endl << endl << endl;
// Get next line of data from file inData >> loanAmt >> yearlyInt >> numYears;
}
// Close file (necessary?) inData.close();
cout << "Good bye!" << endl;
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
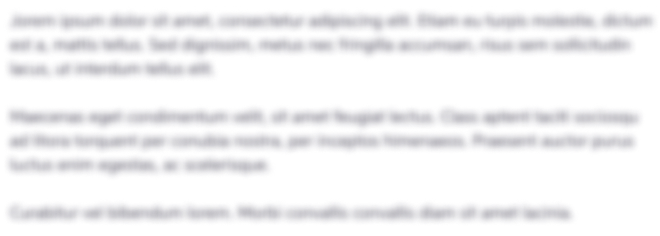
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started