Question
Task 2.3: Finding Maximum Daily Increase in Cases Our third task is to implement find_max_increase_in_cases which takes in one argument n_cases_increase and finds the maximum
Task 2.3: Finding Maximum Daily Increase in Cases
Our third task is to implement find_max_increase_in_cases which takes in one argument n_cases_increase and finds the maximum daily increase in confirmed cases for each country.
In this case, n_cases_increase is the output obtained from calling compute_increase_in_cases(n_cases_cumulative).
The return value should be a 1D np.ndarray that represents the maximum daily increase in cases for each country. In particular, the -th entry of this array should correspond to the increase in confirmed cases in the -th country as represented in n_cases_increase.
Returning to our previous example in task 2.2, suppose the daily increase in cases is given by np.array([[1, 1, 1, 1], [1, 2, 3, 4]]). Clearly, the maximum daily increase in cases is 1 and 4 for country 0 and 1, respectively. Therefore, we should expect the output of this function to be np.array([1, 4]).
In this task, the goal is to learn how to use np.max, or the equivalent np.amax, to find the max value in a column of a matrix. The following is a sample execution of how these functions work:
In [ ]:
a = np.arange(4).reshape((2,2)) # array([[0, 1], [2, 3]])
np.amax(a) # 3 -> Maximum of the flattened array
np.amax(a, axis=0) # array([2, 3]) -> Maxima along the first axis (first column)
np.amax(a, axis=1) # array([1, 3]) -> Maxima along the second axis (second column)
np.amax(a, where=[False, True], initial=-1, axis=0) # array([-1, 3])
b = np.arange(5, dtype=float) # array([0., 1., 2., 3., 4.])
b[2] = np.NaN # array([ 0., 1., nan, 3., 4.])
np.amax(b) # nan
In [ ]:
def find_max_increase_in_cases(n_cases_increase):
'''
Finds the maximum daily increase in confirmed cases for each country.
Parameters
----------
n_cases_increase: np.ndarray
2D `ndarray` with each row representing the data of a country, and the columns
of each row representing the time series data of the daily increase in the
number of confirmed cases in that country, i.e. the ith row of
`n_cases_increase` contains the data of the ith country, and the (i, j) entry of
`n_cases_increase` is the daily increase in the number of confirmed cases on the
(j + 1)th day in the ith country.
Returns
-------
Maximum daily increase in cases for each country as a 1D `ndarray` such that the
ith entry corresponds to the increase in confirmed cases in the ith country as
represented in `n_cases_increase`.
'''
# TODO: add your solution here and remove `raise NotImplementedError`
raise NotImplementedError
In [ ]:
# Test case for Task 2.3
n_cases_increase = np.ones((100, 20))
actual = find_max_increase_in_cases(n_cases_increase)
expected = np.ones(100)
assert(np.all(actual == expected))
sample_increase = np.array([[1,2,3,4,8,8,10,10,10,10],[1,1,3,5,8,10,15,20,25,30]])
expected2 = np.array([10, 30]) # max of [1,2,3,4,8,8,10,10,10,10] => 10, max of [1,1,3,5,8,10,15,20,25,30] => 30
assert(np.all(find_max_increase_in_cases(sample_increase) == expected2))
Step by Step Solution
There are 3 Steps involved in it
Step: 1
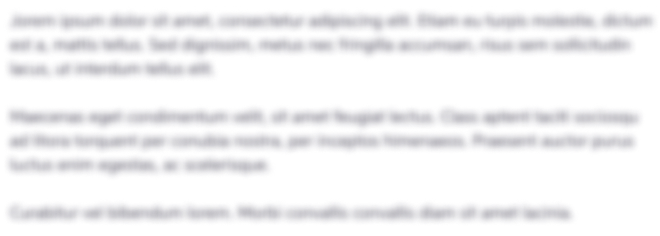
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started