Question
************* th.ap******************* Note : you have to use one the files below to complete the program. Note : please test you peogram and show some
************* th.ap*******************
Note : you have to use one the files below to complete the program.
Note : please test you peogram and show some output.
Write code that forks into two processes: a parent process, and a child process.
NOTE : you are only have to do one ( REGULAR VERSION or PREMIUM VERSION ) .
--------------------------------------------------------------------------------
REGULAR VERSION
Your code will ask the user for an arbitrary sized set of positive numbers. Do not worry about bad input such as "xyz". Your code will not be tested in this way. Also, for the regular version you can ignore any divide by zero issues (these will deal with in the premium version). There will be at least 2 numbers sent. The parent process will take each number one at a time, you can usescanf for this:
scanf("%7s", buffer); // use 7 as the maximum string size Use a char array of 8 in size (this will hold 7 digits as there is a null character on the end). The first number will be about 5 digits or smaller in size, the other numbers will be smaller (1 or 2 digits) in size so 7 possible digits gives some room. If the user types a 'q', this is the end of the numbers. The child process will read the numbers sent by the parent process one at a time, and divide them accumulatively. for example, the numbers 200 2 10 5 should calculate 200 / 2 = 100 /10 = 10 / 5 = 2. Since the numbers are integers, the result will have any fractional part truncated by default; this is okay.
NOTE: Keep in mind that the child is getting a string of characters. Use atoi to convert the strings to actual ints.
Start by reading the first number and initialize the quotient to this number. Then get the other numbers in a loop. The child process will communicate the quotient of the numbers to the parent as the return value from main(). The parent process will need to reap the child process to find out the quotient. Remember to close the pipe ends when your program is done.
--------------------------------------------------
EXAMPLE RUN
CS - Assignment 3 Regular - david enter number (or 'q' to quit)300 enter number (or 'q' to quit)5 enter number (or 'q' to quit)3 enter number (or 'q' to quit)2 enter number (or 'q' to quit)q
quotient = 10
--------------------------------------------------------
Important: printing should be done only by the parent process. The child process should not print anything.
Your output should be formatted as shown (small differences in wording or whitespace is OK. Make sure all asked for info is there). Remember to replace "I. Forgot" with your own name. Start with the provided code file a03.c.
--------------------------------------------------------------------------------
PREMIUM VERSION
Same as the Regular version, except that your code should catch any possible SIGFPE signal that might be generated if one of the non-first numbers is a zero (yes, it's probably better to check for the zero first but the point of this assignment is to practice using signal handlers). If caught, just print out the error message:
"caught signal
NOTE: Replace
Call the exit function with -1. Don't return or you will get into an infinite loop and will need to hit ctrl-C to get out of the loop.
NOTE: the signal handler should be setup in the child process. The parent process can't use WIFSIGNALED(status) since the signal is being caught. However if the exit value is -1, the return value is 255 so the parent can pick this up and print out a "child aborted" message. NOTE: I will avoid any series of numbers where the final quotient will be 255. Note also that you will need to type a 'q' in the parent program to end the parent process if the child has aborted.
----------------------------------------------------------
EXAMPLE RUN
CS - Assignment 3 Premium - David enter number (or 'q' to quit)400 enter number (or 'q' to quit)20 enter number (or 'q' to quit)0 enter number (or 'q' to quit)
caught signal 8 from 31691. Aborting program!
q
child aborted
Start with the provided code file a03p.c.
--------------------------------------------------------------------------------
REQUIRED PLATFORM
I grade your code on a Linux system running gcc version 5.4.0 Your code should be portable enough to compile and run correctly on any Linux machine.
GRADING YOUR CODE
Your code needs to compile without errors or warnings and run correctly (use the -Wall flag while developing your code). Code that does not compile and crashes on normal input will receive much fewer points. Please don't use filenames with spaces or '-' in it. These names don't work with my grading scripts.
---------------------------------------------------------
******** a03.c ********
// Numbers from the user are sent to child process
// from parent process one at a time through pipe.
//
// Child process divides numbers sent through pipe.
//
// Child process returns quotient of numbers to parent process.
//
// Parent process prints quotient of numbers.
// NOTE: Make sure child does NOT print anything to the terminal
// or you will get much fewer points.
#include
int main(int argc, char **argv)
{
printf("CS - Assignment 3 Regular - I. Forgot ");
// start code here. remember to set up pipe before
// doing a fork. Always check for errors with all
// system calls.
}
----------------------------------------------------------------
**** a03p.c ****
// Numbers from the user are sent to child process
// from parent process one at a time through pipe.
//
// Child process divides numbers sent through pipe.
//
// Child process returns quotient of numbers to parent process.
//
// Parent process prints quotient of numbers.
// NOTE: Child process should not print anything
// EXCEPT the error message when the SIGFPE signal is given.
//
// PREMIUM: Child catches divide by zero (SIGFPE) and
// prints error message (see instructions). Note that
// parent will need to handle a situation for the
// child might end abnormally.
#include
// need signal handler - put it here
int main(int argc, char **argv)
{
printf("CS - Assignment 3 Premium - I. Forgot ");
// start code here. remember to set up pipe before
// doing a fork. Always check for errors with all
// system calls. set up signal handling inside the child
// and when the quotient returned is 255 in the parent, this will
// be considered an abort.
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
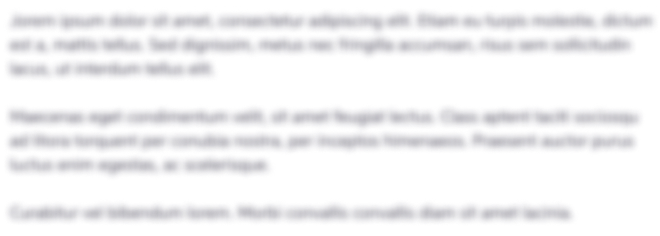
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started