Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The DictionaryTester code: import org.junit.jupiter.api.*; import java.util.ArrayList; import static org.junit.jupiter.api.Assertions.assertEquals; class DictionaryTester { private static int totalScore, extraCredit; private final String ERROR_ONE = ;
The DictionaryTester code:
import org.junit.jupiter.api.*; import java.util.ArrayList; import static org.junit.jupiter.api.Assertions.assertEquals; class DictionaryTester { private static int totalScore, extraCredit; private final String ERROR_ONE = "PART B - Java Programming, Data Structures, and Data Design, 45 points We are hired to implement an interactive dictionary. Our dictionary takes input from users and uses the input as search key to look up values associated with the key. Requirements: - Coding: No hard coding, https://en.wikipedia.org/wiki/Hard_coding. Please think Dynamic and Scalable. - Data Source: Store the original data in a set of enum objects. Each keyword, each part of speech, and each definition must be stored in a separate data field. Do not combine them such as storing three parts in one String. - Data Structure: Use existing data structure(s) or creating new data structure(s) to store our dictionary's data. - Data Loading: When our program starts, it loads all the original data from the Data Source into our dictionary's data structure. Data Loading must finish before our program starts interacting with users. - User Interface: A program interface allows users to input search keys. This interface then displays returned results. Our program searches the dictionary's data (not the Data Source) for values associated with the search keys. - Identical Output: Our program's output must be identical to the complete sample run's output. The complete output is ! Loading data... ! Loading completed... -----DICTIONARY 340 JAVA----- Search: ar ROH Arrow (noun) : Here is one arrow: CIMG> -->> Search: di STINCT Distinct (adjective) Familiar. Worked in Java Distinct (adjective) : Unique. No duplicates. Clearly different or of a different kind Distinct (adverb) Uniquely. Written "distinctly". Distinct (noun) : A keyword in this assignment Distinct (noun) : A keyword in this assignment. Distinct (noun) : A keyword in this assignment. Distinct (noun) : An advanced search option Distinct noun Distinct is a parameter in this assignment. Search: distinct distinct Distinct [adjective) : Familiar. Worked in Java. Distinct fadjective] : Unique. No duplicates. clearly different or of a different kind. Distinct [adverb) Uniquely. Written distinctly". Distinet [noun] A keyword in this assignment. Distinct noun 1 An advanced search option. Distinct noun] Distinct is a parameter in this assignment. Search: diaTINCT noun distinct Distinct (noun) : A keyword in this assignment. Distinct (noun) : An advanced search option Distinct (noun) : Distinct is a parameter in this assignment. Search: PLACEHOLDER Placeholder (adjective) : To be updated... Placeholder (adjective) : To be updated... Placeholder (adverb) : To be updated... Placeholder (conjunction) : To be updated... Placeholder (interjection) : To be updated... Placeholder (noun) : To be updated... Placeholder (noun) : To be updated... Placeholder (noun) To be updated... Placeholder (preposition) To be updated. Placeholder (pronoun) To be updated... Placeholder (verb) To be updated... Search: placaHOLDER adjective Placeholder adjective) To be updated... Placeholder [adjective] : To be updated... Search: PLACEholder adjective distinct Placeholder (adjective) : To be updated... Search: placeHOLDER distinct Placeholder [adjective) : To be updated... Placeholder (adverb) To be updated... Placeholder (conjunction) : To be updated... Placeholder (interjection) : To be updated... Placeholder (noun) : To be updated... Placeholder (preposition) : To be updated... Placeholder (pronoun) ! To be updated... Placeholder (verb) : To be updated... Search: cse340 distinct CSC340 [adjective) = C+version of CSC210 + CSC220 + more. CSC340 [noun] ACS upper division course. CSC340 (noun) : Many hours outside of class. CSC340 [noun] : Programming Methodology Search: cse340 noun CSC340 (noun) ! ACS upper division Course. CSC340 (noun) Many hours outside of class CSC340 (noun) Programming Methodology Search: cs6220 adjective distinct CSC220 (adjective) Ready to create complex data structures Search: $c220 verb CSC220 (verb) to create data structures. Search: book distinct Book (noun) Book [noun] Book (verb) Book [verb] A set of pages. A written work published in printed or electronie for. to arrange for someone to have a seat on a plane. To arrange something on a particular date. Search: adVERB noun distinct Adverb (noun) Adverb is a word that adds more information about place, time, manner, cause Or degree to a verb, an adjective, a phrase or another adverb. Search: ADJECTIVE distinct red and Adjective (noun) : Adjective is a word that describes a person or thing, for example big elever in a big house, red wine and a clever idea. Search: CONJUNCTION verb"; private final String ERROR_TWO = " "; private static Dictionary d; @BeforeAll static void setUp() { totalScore = 0; extraCredit = 0; d = new Dictionary(); d.init(); } @Test void testEmpty() { ArrayList testArray = new ArrayList (); testArray.add(ERROR_ONE); //testArray.toArray(); assertEquals(testArray, d.querryDictionary(null, null, false), "Make sure to return the properly formatted Error Message"); totalScore += 10; } @Test void testPlaceholder() { ArrayList testArray = new ArrayList (); testArray.add("Placeholder [adjective] : To be updated..."); testArray.add("Placeholder [adjective] : To be updated..."); testArray.add("Placeholder [adverb] : To be updated..."); testArray.add("Placeholder [conjunction] : To be updated..."); testArray.add("Placeholder [interjection] : To be updated..."); testArray.add("Placeholder [noun] : To be updated..."); testArray.add("Placeholder [noun] : To be updated..."); testArray.add("Placeholder [noun] : To be updated..."); testArray.add("Placeholder [preposition] : To be updated..."); testArray.add("Placeholder [pronoun] : To be updated..."); testArray.add("Placeholder [verb] : To be updated..."); assertEquals(testArray, d.querryDictionary("Placeholder", null, false), "Make sure the Order is correct"); totalScore += 3; } @Test void testMatchCase() { ArrayList testArray = new ArrayList (); testArray.add("Placeholder [adjective] : To be updated..."); testArray.add("Placeholder [adjective] : To be updated..."); testArray.add("Placeholder [adverb] : To be updated..."); testArray.add("Placeholder [conjunction] : To be updated..."); testArray.add("Placeholder [interjection] : To be updated..."); testArray.add("Placeholder [noun] : To be updated..."); testArray.add("Placeholder [noun] : To be updated..."); testArray.add("Placeholder [noun] : To be updated..."); testArray.add("Placeholder [preposition] : To be updated..."); testArray.add("Placeholder [pronoun] : To be updated..."); testArray.add("Placeholder [verb] : To be updated..."); assertEquals(testArray, d.querryDictionary("PLAceHoldeR", null, false), "Make sure the Order is correct"); testArray.clear(); testArray.add("Bookable [adjective] : Can be ordered in advance."); assertEquals(testArray, d.querryDictionary("boOkAble", null, false), "Make sure the Order is correct"); testArray.clear(); testArray.add("Book [noun] : To arrange for someone to have a seat on a plane."); testArray.add("Book [noun] : To arrange something on a particular date."); assertEquals(testArray, d.querryDictionary("book", null, false), "Make sure the Order is correct"); testArray.clear(); testArray.add("CSC340 [adjective] : = C++ version of CSC210 + CSC220 + more."); testArray.add("CSC340 [noun] : A CS upper division course"); testArray.add("CSC340 [noun] : Many hours outside of class."); testArray.add("CSC340 [noun] : Programming Methodology"); assertEquals(testArray, d.querryDictionary("csC340", null, false), "Make sure the Order is correct"); totalScore += 10; } @Test void testTypeOfSpeech() { ArrayList testArray = new ArrayList (); testArray.add("CSC340 [noun] : A CS upper division course"); testArray.add("CSC340 [noun] : Many hours outside of class."); testArray.add("CSC340 [noun] : Programming Methodology"); assertEquals(testArray, d.querryDictionary("CSC340", "noun", false), "Make sure you have the correct types of speech"); testArray.clear(); testArray.add("CSC220 [adjective] : Ready to create complex data structures."); assertEquals(testArray, d.querryDictionary("CSC220", "adjective", false), "Make sure you have the correct types of speech"); testArray.clear(); testArray.add("CSC220 [noun] : Data Structures."); assertEquals(testArray, d.querryDictionary("CSC220", "noun", false), "Make sure you have the correct types of speech"); testArray.clear(); testArray.add("CSC220 [verb] : To create data structures."); assertEquals(testArray, d.querryDictionary("CSC220", "verb", false), "Make sure you have the correct types of speech"); testArray.clear(); testArray.add(ERROR_ONE); assertEquals(testArray, d.querryDictionary("CSC220", "pronoun", false), "Make sure the error is correctly formatted"); testArray.clear(); testArray.add(ERROR_TWO); assertEquals(testArray, d.querryDictionary("CSC220", "reverb", false), "Make sure the error is correctly formatted"); totalScore += 5; } @Test void testDistinct() { ArrayList testArray = new ArrayList (); testArray.add("Placeholder [noun] : To be updated..."); assertEquals(testArray, d.querryDictionary("Placeholder", "noun", true), "Should only be one item"); testArray.clear(); testArray.add("CSC340 [noun] : A CS upper division course"); testArray.add("CSC340 [noun] : Many hours outside of class."); testArray.add("CSC340 [noun] : Programming Methodology"); assertEquals(testArray, d.querryDictionary("CSC340", "noun", true), "Should have three items"); testArray.clear(); testArray.add("Placeholder [adjective] : To be updated..."); assertEquals(testArray, d.querryDictionary("Placeholder", "adjective", true), "Should have one item"); totalScore += 5; } @Test void testErrors() { ArrayList testArray = new ArrayList (); testArray.add(ERROR_TWO); assertEquals(testArray, d.querryDictionary("Placeholder", "placeholder", false), "Check your error format"); assertEquals(testArray, d.querryDictionary("Bookable", "book", true), "Check your error format"); assertEquals(testArray, d.querryDictionary("Pronoun", "reverb", false), "Check your error format"); testArray.clear(); testArray.add(ERROR_ONE); assertEquals(testArray, d.querryDictionary("Book", "adjective", true), "Check your error format"); assertEquals(testArray, d.querryDictionary("BookableBook", "noun", false), "Check your error format"); assertEquals(testArray, d.querryDictionary("P", "noun", false), "Check your error format"); assertEquals(testArray, d.querryDictionary("Place", "verb", true), "Check your error format"); totalScore += 2; } @Test void testExtraCredit() { ArrayList testArray = new ArrayList (); testArray.add("Placeholder [adjective] : To be updated..."); testArray.add("Placeholder [adverb] : To be updated..."); testArray.add("Placeholder [conjunction] : To be updated..."); testArray.add("Placeholder [interjection] : To be updated..."); testArray.add("Placeholder [noun] : To be updated..."); testArray.add("Placeholder [preposition] : To be updated..."); testArray.add("Placeholder [pronoun] : To be updated..."); testArray.add("Placeholder [verb] : To be updated..."); assertEquals(testArray, d.querryDictionary("Placeholder", null, true), "This is Extra, don't worry if it doesn't pass"); extraCredit += 3; } @AfterAll static void printResults() { System.out.println("Assuming the implementations were not hard-coded specifically for the test cases"); System.out.println("Total Score: " + totalScore); System.out.println("Total Extra Credit: " + extraCredit); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
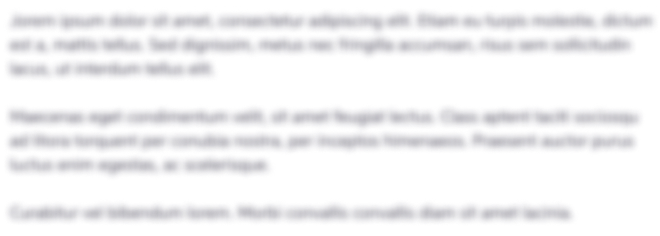
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started