Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The first stage of the project is to recognize the commands the user enters and split them up into their relevant words. Although understanding English
The first stage of the project is to recognize the commands the user enters and split them up into their relevant words. Although understanding English might appear to be quite complex, in reality, most early IF games took a simplified approach: every command was assumed to be of the form VERB NOUN.
Your task for this stage of the project is three-fold:
Using string and getline, accept input (repeatedly) from the user and split it into the noun and verb parts. At this level, you can assume that the user will only type the noun and the verb, with one or more spaces between them.
Support the following commands:
Command Description
LOOK Describe the location
LOOK noun Describe noun
GET noun Pick up noun
DROP noun Drop the noun
INVENTORY List everything the player is carrying right now
GO direction Move in the given direction
QUIT Exit the game
(If you want to add extra commands beyond these, feel free.)
Note that since your game only has one location, GO with any direction should just respond You cant go that way.. Similarly, you can only GET an object that is in the current location (and not already being carried), and you can only DROP an object that is being carried.
Commands that dont make sense (or that are not in the above list) should print a suitable error message. (Since you only have one location, the GO command should always print You cant go that way.)
Create an environment with one room (location) and one object. At a minimum, the player should be able to pick up and drop the object. You can make the setting of your game anything you like. Both the location and object should have full descriptions so that if you LOOK at them they are described in detail
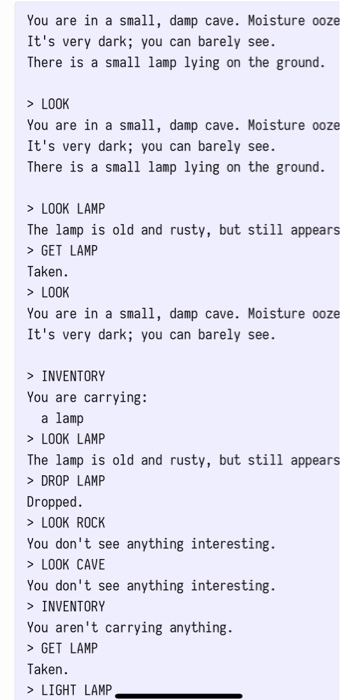
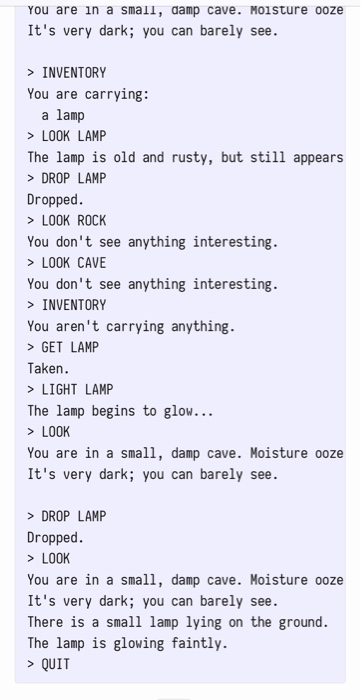
Notice that when you LOOK, the description of the room changes depending on whether or not were carrying the lamp. Similarly, you can try to LOOK at things like the rock walls or the cave itself, and you get an error message. (But one that tries not to break the immersion! Rather than saying thats an error, the game pretends that you can look at anything, its just that most things arent interesting.)
The sample adds a command to light the lamp; the code has to keep track of whether or not the lamp is lit, and adjust the messages printed accordingly.
Implementation hints
If youre having trouble getting started, try these hints. (You dont have to follow these hints if you want to structure your program differently.)
Almost all of main will be a while(true) loop.
Any variables which should keep their values from one game turn to the next should be declared before the loop. (Remember that variables declared inside a loop are reset every time the loop runs.)
Use getline to get the players input, and then use two string variables, together with substr and find/rfind like we did in class to split the input into noun and verb. Note that the LOOK verb comes in two forms: one with a noun (meaning look at this, and one without (meaning look at my general surroundings).
Use a big if-else if-else chain to compare these variables to all the commands and noun(s) your game understands, and respond to them in the appropriate way.
Use a bool variable to keep track of whether or not the player is carrying the object. When the player GETs the object, set it to true; when the player drops it, set it to false. Use this variable in the above if-else if-else conditions when you need to make something different happen depending on whether or not the player is carrying the object.
The QUIT command can just break from the while(true) loop.
Thus, your main will end up looking something like this:
------------------------------------------------------
int main() {
bool carrying_potato = false; // Is the player carrying the potato?
while(true) {
string line;
getline(cin, line);
string verb = ... ; // Split line into verb, noun
string noun = ... ;
if(verb == "look") {
}
else if(verb == "get") {
}
else if(verb == "quit") {
break;
}
else {
cout
}
} // end of while
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
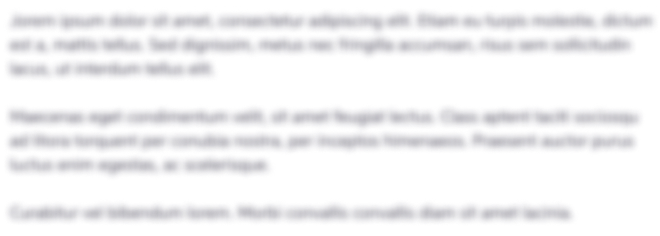
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started