Question
The following is a java code for stack. I need help with adding a new function into the code, I'm not sure what other function
The following is a java code for stack. I need help with adding a new function into the code, I'm not sure what other function would be useful to add in a stack implementation code besides the functions that are already in the code. It could be the search function or any other, except for the range function. Do not add a range function. Just something that could be useful when dealing with the stack data structure.
import java.util.*; /* Class arrayStack */ class arrayStack { protected int arr[]; protected int top, size, len; /* Constructor for arrayStack */ public arrayStack(int n) { size = n; len = 0; arr = new int[size]; top = -1; } /* Function to check if stack is empty */ public boolean isEmpty() { return top == -1; } /* Function to check if stack is full */ public boolean isFull() { return top == size -1 ; } /* Function to get the size of the stack */ public int getSize() { return len ; } /* Function to check the top element of the stack */ public int peek() { if( isEmpty() ) throw new NoSuchElementException("Underflow Exception"); return arr[top]; } /* Function to add an element to the stack */ public void push(int i) { if(top + 1 >= size) throw new IndexOutOfBoundsException("Overflow Exception"); if(top + 1 < size ) arr[++top] = i; len++ ; } /* Function to delete an element from the stack */ public int pop() { if( isEmpty() ) throw new NoSuchElementException("Underflow Exception"); len-- ; return arr[top--]; } /* Function to display the status of the stack */ public void display() { System.out.print(" Stack = "); if (len == 0) { System.out.print("Empty "); return ; } for (int i = top; i >= 0; i--) System.out.print(arr[i]+" "); System.out.println(); } } /* Class StackImplement */ public class StackImplement { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Stack Test "); System.out.println("Enter Size of Integer Stack "); int n = scan.nextInt(); /* Creating object of class arrayStack */ arrayStack stk = new arrayStack(n); /* Perform Stack Operations */ char ch; do{ System.out.println(" Stack Operations"); System.out.println("1. push"); System.out.println("2. pop"); System.out.println("3. peek"); System.out.println("4. check empty"); System.out.println("5. check full"); System.out.println("6. size");
int choice = scan.nextInt(); switch (choice) { case 1 : System.out.println("Enter integer element to push"); try { stk.push( scan.nextInt() ); } catch (Exception e) { System.out.println("Error : " + e.getMessage()); } break; case 2 : try { System.out.println("Popped Element = " + stk.pop()); } catch (Exception e) { System.out.println("Error : " + e.getMessage()); } break; case 3 : try { System.out.println("Peek Element = " + stk.peek()); } catch (Exception e) { System.out.println("Error : " + e.getMessage()); } break; case 4 : System.out.println("Empty status = " + stk.isEmpty()); break; case 5 : System.out.println("Full status = " + stk.isFull()); break; case 6 : System.out.println("Size = " + stk.getSize()); break; default : System.out.println("Wrong Entry "); break; } /* display stack */ stk.display(); System.out.println(" Do you want to continue (Type y or n) "); ch = scan.next().charAt(0); } while (ch == 'Y'|| ch == 'y'); } }
Please add another function, except range and make sure the code runs. Thank you.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
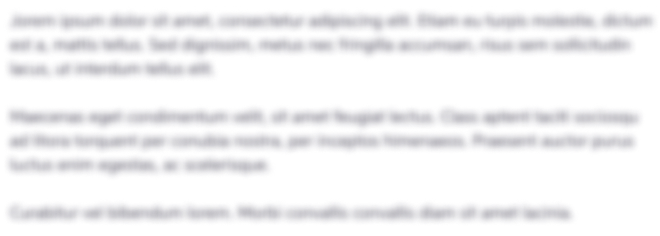
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started