Question
The following tutorial is designed for you to practice some of the basics of debugging. Create a new Console project and replace the generated .cpp
The following tutorial is designed for you to practice some of the basics of debugging. Create a new Console project and replace the generated .cpp file with the following code:
#include "stdafx.h"
#include
#include
using namespace std;
/* PURPOSE: To skip n spaces on a line
RECEIVES: n - the number of spaces to skip
REMARKS: n should be non-negative
*/
void skip(int n)
{
int i; /* a counter */
for (i = 0; i <= n; i++ )
cout << " ";
}
/* PURPOSE: To calculate n factorial
RECEIVES: n - calculate the factorial of n
RETURNS: n factorial
REMARKS: n must be >= 0. Also if n is too large overflow may result
*/
int factorial(int n)
{
int product = 1; /* accumulator for the running product */
for (int i = 1; i <= n; i++)
{
product = product + i ;
}
return(product);
}
/* PURPOSE: to calculate the number of combinations of n things taken
k at a time (n choose k)
RECEIVES: n - the number of items to choose from
k - the number of items choosen
RETURNS: n choose k
REMARKS: n and k must be non-negative and k <= n. This program uses
the formula (n choose k) = n! / ( k! * (n-k)! ).
*/
int combination(int n, int k)
{
int comb = factorial(n) / factorial(k) * factorial(n-k);
return comb;
}
int main(void)
{
int nrows; /* the number of rows to print */
int n; /* a counter for the current row */
int k; /* a counter for the current column */
int comb; /* the number of combinations */
int spaces_to_skip; /* spaces to skip */
cout << "Enter the number of rows (<=13) in Pascal's triangle: ";
cin >> nrows;
cout << " ";
/* print the title * /
skip(16);
cout << "TABLE 1: THE FIRST " << nrows
<< " ROWS OF PASCAL'S TRIANGLE ";
/ * start a loop over the number of rows */
spaces_to_skip = 36;
for (n = 0; n < nrows; n = n + 2)
{
skip(spaces_to_skip); /* space to make a triangle */
spaces_to_skip = spaces_to_skip - 2;
for (k = 0; k <= n; k++)
{
comb = combination(n,k);
cout << setw(4) << comb;
}
cout << " " ;
}
system("pause");
return 0;
}
The program's purpose is to display Pascal's Triangle, a sequence of integers that arises in numerous areas of math and computer science, especially combinatorics and probability. When completed, the program's output should be:
Enter the number of rows (<= 13) in Pascal's Triangle. 5
THE FIRST 5 ROWS OF PASCAL's TRIANGLE:
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
Entries in Pascal's triangle are indexed by integers. n is the number of the row and k is the position from the leftmost member of the row. The indexes in both directions start at zero (0), so in the fifth row listed above, C(4,0) = 1, C(4,1) = 4, and so on.
The values themselves are computed by the formula C(n, k) = n! / ( k! * (n-k)!), which is called a combination. n! denotes a factorial, that is, the product n(n-1)(n-2)...(2)(1). The combinations can be interpreted as the number of ways to choose k elements from a collection containing n elements. When described, it is customary to say "n choose k", for instance '4 choose 3 is 4' ".
If 4 objects are numbered 1 through 4, how many ways can you select 2 of them ? Show all the possible pairings here. Then compute C(4, 2) by using the formula.. Does it match the number of selections?
As you will discover with the help of your debugger, the program to compute Pascal's triangle has some mistakes. Each provides an opportunity to learn a debugging concept in context of its use.
Compile the file
- Select Build Solution from the Build menu.
- Select the Start Debugging command from the Debug menu.
- Enter 5 for input (the number of rows for the program to display)
What output is displayed?
Step over / inspect
- Then use the Step Into command (F11 shortcut) to restart the program from the very beginning.
- Now use the Step Over command (F10 shortcut) several times, until the active line is cin >> nrows;
- Select Step Over again
- The program is now expecting keyboard input because the cin >> nrows line is being executed.
- Type 5 (followed by the Enter key) for the input.
On what line is the cursor positioned now ?
- Place your cursor over the nrows variable to inspect its value
What is the value for nrows shown in the dialog box?
More stepping
- Select Step Over twice
- Look at the output for Pascal's triangle.
Does the output have the title: TABLE 1: THE FIRST 5 ROWS OF PASCAL'S TRIANGLE ?
That is strange. The program is supposed to print three newlines, then the title. Let's find out why the title doesn't appear.
- Select Stop Debugging in the Debug menu to stop the debugger
- Select Step Into
- Then select Step Over until you reach the line prompting for input.
- Enter 5 for nrows
- Select Step Over one more time. This should skip over the line cout << ;
- Select Step Over one more time.
What line are you on now?
- Notice that you skipped over the line cout << "TABLE 1: THE FIRST " << nrows << " ROWS OF PASCAL'S TRIANGLE "); The reason is that two delimiters for comments had extra spaces in them.
- Stop the debugger
- Change the line /* print a title * / into /* print a title */ and the line / * start a loop over the number of rows */ into /* start a loop over the number of rows */
When you changed the positioning of the comment delimiters, did any changes occur to the commented text?
Watch
- Rebuild your solution
- Select Step Into
- Select Step Over until input is requested.
- Enter 5 for the number of rows as before
- Select the Continue command (F5) in Debug menu
Does the title now show up on the screen?
How many rows of Pascal's triangle are displayed?
- To Watch a variable, you must be debugging the project. Select the variable with the cursor and right click with the mouse and select Add watch
- Add n to the watch list.
- Add k to the watch list.
How many lines are now in the watch window?
- Stop the debugger
- Select Step Into
- Select Step Over until you reach the line skip(spaces_to_skip); /* space to make a triangle */
What values of n and k are in the watch window?
- Keep selecting Step Over until you are again at the line skip(spaces_to_skip); /* space to make a triangle */.
What are the values of n and k?
- Keep selecting Step Over until you exit the for loops and arrive at the line system(pause);
Now what are the values of n and k?
- View the program output. Notice that there are only three rows in the triangle. This is because the n values listed above went from 0 to 2 to 4 to 6. That is, the loop index i should have been increased by one each time, not two.
- Change the line of code: for (n = 0; n < nrows - 1; n = n + 2) to be for (n = 0; n < nrows; n++)
- Rebuild your solution
- Select Step Into
- Select Step Over until input is requested.
- Enter 5 for the number of rows as before
- Select the Continue command (F5) in Debug menu. You should now have five rows in the triangle.
Step into
- The entries in the triangle are still incorrect. To see one reason why, Step Into the program, then press Step Over quite a few times, until the watch window first displays:
n: 2
k: 0
You should be at line comb = combination(n, k);
- Press Step Into, to get to the line int combination = factorial(n) / factorial(k) * factorial(n-k); Notice that Step Into goes into each function as it is executed. Using Step Over always steps over the next function to the next line of code in the current procedure.
- Press Step Into again. You should be at the line int product = 1; /* accumulated product */ in the function factorial(n)
- Place your cursor over the product variable to inspect its value
- Now press Step Into until you are at the line return product;
What value does n have in the window? (Note that k is dimmed in the watch window since k is not active in the function factorial).
What is the value of product?
- The reason that product is incorrect is that the line
product = product + i;
should be
product = product * i;
- Make the above change in your editor and Rebuild your solution.
- Select Step Into
- Select Step Over until input is requested.
- Enter 5 for the number of rows as before
- Select the Continue command (F5) in Debug menu. Oh no! The triangle is still wrong!
Setting breakpoints
It is tedious to have to keep stepping to get inside a program. A more efficient way is to set a breakpoint at a line of interest. Then the program runs at full speed until a breakpoint is reached.
- Select the line comb = combination(n,k) ;
- Right click with the mouse button and select Toggle Breakpoint. You should see a red circle appear in the column to the left of the code region. You can toggle a breakpoint by directly clicking in this region next to the line you wish the debugger to break on.
- Select Start Debugging in the Debug menu. The program will stop at the breakpoint.
- Repeatedly select Continue from the Debug menu until the watch window displays
n: 3
k: 1
- Step Into once
- Step Over until you are at the line: return comb;
- Place your cursor over the comb variable to inspect its value
What is the value listed for comb?
- The true value for the combination of 3 things taken 1 at a time is 3! / 1!2! = 6 / 2 = 3. So, the above value of comb is wrong.
- Something must be wrong with our combination calculation. In fact, the programmer has omitted parentheses in the denominator for the line:
comb = factorial(n) / factorial(k) * factorial(n-k) ;
- Change the line to be:
comb = factorial(n) / (factorial(k) * factorial(n-k)) ;
- Rebuild the solution and select Start Debugging
Is your Pascal's Triangle correct (for nrows = 5)?
Finally
- Select the line comb = combination(n,k) ;
- Select Toggle Breakpoint.
- Select Start Debugging
Do the results look OK when you input 13 for the number of rows?
They should now. Exit, saving any files you might wish to keep.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
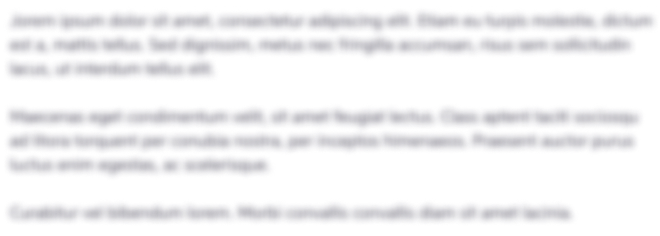
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started