Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The goal of this asigment is to implement a single linked list as follows: IntSLList.h Singly linked node This class implements the singly linked list
The goal of this asigment is to implement a single linked list as follows:
IntSLList.h
Singly linked node This class implements the singly linked list using templates Each list has two attributes: -head: first node in the list -tail: last node in the list Considerations: 1. head and tail point to null in an empty list 2. tail->next=null ******************/ #include "IntSLLNode.h" templateclass IntSLList{ public: //Default constructor: creates an empty list IntSLList(); //Destructor: deallocate memory ~IntSLList(); //addToHead(T val): creates a new node with val as info, //and this new node is the new head void addToHead(T val); //addToTail(T val): creates a new node with val as info, //and this new node is the new tail void addToTail(T val); //deleteFromHead: remove head from the list, //the new head is the previous head->next //if the list had only one node, head and tail point null void deleteFromHead(); //deleteFromTail: remove tail from the list, //the new tail is the previous node to tail //if the list had only one node, head and tail point null void deleteFromTail(); //In the list is empty, returns true //otherwise, returns false bool isEmpty(); //sortInsert(T val): creates a new node, and it is inserted sortly void sortInsert(T val); //insert(int pos, T val): creates a new node, and it is inserted in position pos void insert(int pos, T val); //printList(): prints all nodes in the list void printList(); private: IntSLLNode *head; //A pointer to the first node IntSLLNode *tail; //A pointer to the last node }; /**************** Default constructor: creates an empty list head and tail point null *****************/ template IntSLList ::IntSLList() { tail = head = 0; } /*********************** Destructor: deallocate memory removing each node from the list *****************/ template IntSLList ::~IntSLList() { //Declare a pointer prtNode IntSLLNode *prtNode; //prtNode points head prtNode=head; //While there is a node in the list, remove it while(prtNode != 0) { //prtNode points head->next prtNode = head->getNext(); //delete head delete head; //the new head is prtNode head=prtNode; } } //other methods here
then we have:
IntSLLNode.h
Singly linked node This class implements the singly linked node using templates Each nodes two attributes: -info: stores the actual value -next: a pointer to the next node in the list ******************/ #includeusing namespace std; /********************************** Class Declaration ***********************/ template class IntSLLNode{ public: IntSLLNode(); //Default constructor IntSLLNode(T, IntSLLNode *); //Special constructor T getInfo(); //getInfo IntSLLNode * getNext(); // getNext void setInfo(T); //setInfo void setNext(IntSLLNode *); //Set next private: T info; //Actual data IntSLLNode *next; //Points to the next node in the list }; /***** Default constructor Creates a empty node *****/ template IntSLLNode ::IntSLLNode() { next=0; } /****** Special constructor: Creates a node with value i in the info attribute and next pointing to null @params: i: actual value to be stored *n: pointer to the next node *******/ template IntSLLNode ::IntSLLNode(T i, IntSLLNode *n = 0) { info=i; next=n; } /******** getInfo returns the info value *********/ template T IntSLLNode ::getInfo() {return info;} /******** getNext returns a point to next node the list **********/ template IntSLLNode *IntSLLNode ::getNext() {return next;} /******** setInfo Set i as the node info *******/ template void IntSLLNode ::setInfo(T i) { info = i;} /******** setNext Points next attribute to the next list node ********/ template void IntSLLNode ::setNext(IntSLLNode *n = 0) { next = n;}
and finally the main:
singleLinkedList.cpp
#include#include "IntSLList.h" // singleLinkedList.cpp : Defines the entry point for the console application. int main(int argc, char* argv[]) { IntSLList list; int i, k; cout<
Step by Step Solution
There are 3 Steps involved in it
Step: 1
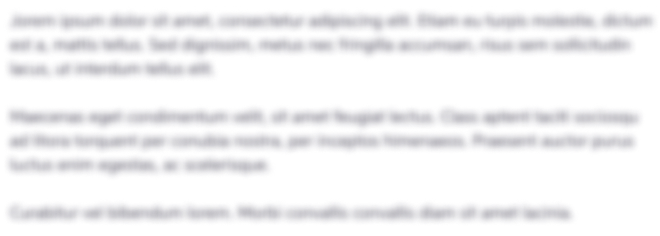
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started