Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The Logical Imitation Game For the next sequence of tasks, we'll be implementing, in stages, a version of Turing Machine. As with the previous sequence
The Logical Imitation Game
For the next sequence of tasks, we'll be implementing, in stages, a version of "Turing Machine". As with the previous sequence Parts & we'll start in Java to explore the structural components, then move to Python for the interaction components.
This task will also step us a little closer to realistic software development though still at a fair distance we have a clear idea of what we're doing for a start
The Starting Point
The scaffold contains a Runner class, and nothing else. Runner is to facilitate you running any testdebugging code you need to complete the task, however it is not part of the tests.
You will have to create public classes, the Machine class, IncorrectDataException, the interface Verifier and its four implementing classes Verifier Verifier Verifier and Verifier
Unlike with Part you will need code that compiles before the tests will run this means that you will not only have to create the appropriate classes, you will need to implement method stubs for all the required methods a method stub is just the correct method header and a functioning return statement if necessary, it doesn't have other code let alone working code One way to do this is to practice reading the error messages...
The IncorrectDataException
We'll cover the exception first because it's simple. You do not need to implement any of the complicated functions of exceptions, and can rely on inheritance to do the work needed for this task. This of course means that when you create it it must inherit from the correct parent class. The obvious choice is the correct one here.
The Verifier interface and its implementations
The Verifier interface should have the following public method:
check that takes two int arrays guess and answer, in that order and returns true if the guess matches the criteria specified.
Each class that implements Verifier should also override the toString method to return the description of the verifier.
For the task, you will only need to implement of the verifiers. The test cases may include other verifiers. The four implementing classes and their logical functionality are:
Verifier "Compares the nd digit to the value is it or
For example, if the answer is then a guess of should return false because and thus the guess must match this criteria because A guess of should return true for this answer as
Verifier "Number of s in the code".
This verifier compares the number of s in the answer to the number in the guess. Eg an answer of no s compared to a guess of also no s returns true, whereas an answer of compared to a guess of should return false as the number of s in the answer is but in the guess is
Verifier "Checks the st digit compared to the nd ie xy
eg if the answer is and the guess is the verifier should respond false, as but
VerifierIf there is a number present exactly twice".
Eg and don't have any pairs has three s not exactly two s however does. So an answer of and a guess of should answer true, but a guess of instead would give the response false.
The Machine
The Machine class should have the following methods:
a public constructor that takes an int array for the game answer and an array of Verifiers.
a public constructor that takes an int array for game answer and a List of Verifiers.
Both constructors must check that the int array is a valid answer digits, all in the range from inclusive and invalid answers must throw an IncorrectDataException.
A toString method that overrides the standard toString and returns a String representing the list of available Verifiers. Details on the format of this are given below.
a public turn method that takes an int array as a guess and an array of char verifier choices, and returns a String describing the results of the guess. It throws an IncorrectDataException if the verifier does not exist, or the guess is out of bounds, or if the number of verifier checks isn't equal to Details on the format of this are given below.
a public turn method that takes an int array and a String of commaseparated verifiers and uses the same logic as turn described above.
a public method finalGuess that takes an int array, and returns true if the input matches the answer. It should throw an IncorrectDataException if the guess is out of bounds.
You will need to add private fields to help you complete these methods, particularly a data structure that holds the answer, and one to store the Verifiers.
Notice again that the verifier chars should be the first
n letters from A B C etc., where
n is the number of verifiers, Your cod
Step by Step Solution
There are 3 Steps involved in it
Step: 1
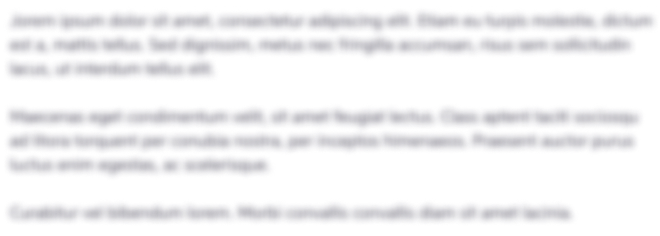
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started