Question
The most obvious is that the ADT will use nodes and they can transverse in two directions (forward and backward). To do that, youll notice
The most obvious is that the ADT will use nodes and they can transverse in two directions (forward and backward). To do that, youll notice in node.h it has a pointer data member called prev that will point to the previous node if it exists (nullptr otherwise). Furthermore, youll notice in clinkedlist.h has a pointer private data member called tailPtr. That specific pointer will always point to the end of the list, just like headPtr always points to the top of the list. To implement these new data members, you will have to revise most of your functions.
Lastly, to test your updated implementation, you will also notice that in main, it will display your list in ascending or descending order. You can view your list in ascending or descending. Therefore, youll have two new functions to implement: DisplayListAscending and DisplayListDescending.
Your objective is to implement the functions in clinkedlist.h onto clinkedlist.cpp and node.h onto node.cpp. You wont need to change anything in main.cpp, clinkedlist.h, or node.h. Your code should mimic the output from the sample executable file.
//MAIN.CPP
#include
// global constants const int MAX_ITEMS = 4;
// ==== main ================================================================== // // ============================================================================
int main() { CLinkedList list; int numToAdd[MAX_ITEMS] = {10, 12, 15, 5};
// The following steps will display, add, and remove items in the list to // test all the functions.
// 1) Display an empty list cout << " 1) Display the number of items and an empty list: "; cout << "Number of items: " << list.GetCurrentSize() << endl; list.DisplayListAscending();
// 2) Clear an empty list cout << " 2) Clearing an empty list: "; if (!list.Clear()) { cerr << " Error in clearing list! "; // 3) Add some numbers cout << " 3) Adding " << MAX_ITEMS << " numbers to fill the list: "; for (int i = 0; i < MAX_ITEMS; ++i) { cout << "Adding " << numToAdd[i] << "... ";
if (!list.Add(numToAdd[i])) { cerr << " Error in adding " << numToAdd[i] << " to the list! "; }
}
// 4) Display the current list in ascending and descending order cout << " 4) Display the number of items, an ascending ordered list, " << "and a descending ordered list: "; cout << "Number of items: " << list.GetCurrentSize() << endl << endl; list.DisplayListAscending(); cout << endl << endl; list.DisplayListDescending();
// 5) Delete the first item value (5 and notice how the items get rearranged) cout << " 5) Delete first item (value 5): "; if (!list.Remove(5)) { cerr << " Error in removing item value " << 5 << " from the list! "; }
// 6) Display list cout << " 6) Displaying list: "; list.DisplayListAscending();
// 7) Delete a middle item value (12 and notice how the items get rearranged) cout << " 7) Delete a middle item (value 12): "; if (!list.Remove(12)) { cerr << " Error in removing item value " << 12 << " from the list! "; }
// 8) Display list cout << " 8) Displaying list: "; list.DisplayListAscending();
// 9) Delete the last item (value 15 and notice how the items get rearranged) cout << " 9) Delete the last item (value 15): "; if (!list.Remove(15)) { cerr << " Error in removing item value " << 15 << " from the list! "; }
// 10) Display list cout << " 10) Displaying list: "; list.DisplayListAscending();
// 11) Check if item value 333 exists in the list cout << " 11) Check if item value 333 exists in the list: "; if (!list.Contains(333)) { cerr << " Item value 333 doesn't exist in the list! "; }
// 12) Delete item value 333, which is not in the list cout << " 12) Delete item value 333, which is not in the list: "; if (!list.Remove(333)) { cerr << " Error in removing item value 333 from the list! "; }
// 13) Clear the list cout << " 13) Clearing the list: "; if (!list.Clear()) { cerr << " Error in clearing list! "; }
// 14) Display list cout << " 14) Displaying an empty list: "; list.DisplayListAscending();
return 0;
} // end of "main"
//clinkedlist.h
#include "node.h"
#ifndef CLinkedLIST_HEADER #define CLinkedLIST_HEADER
class CLinkedList { public: // constructor and destructor CLinkedList(); ~CLinkedList();
// member functions bool DestroyList(); bool GetItem(int index, ListItemType &item) const; int GetCurrentSize() const; bool Add(const ListItemType &newItem); bool IsEmpty() const; bool Remove(const ListItemType &value); bool Clear(); bool Contains(const ListItemType &item) const; void DisplayListAscending(); void DisplayListDescending();
private: // data members Node *headPtr; // head pointer Node *tailPtr; // tail pointer
// member functions char NewItemLocation(const ListItemType &newItem); bool AddInFront(const ListItemType &newItem); bool AddInEnd(const ListItemType &newItem); bool AddInMiddle(const ListItemType &newItem);
};
#endif // CLinkedLIST_HEADER
//node.h #ifndef NODE_HEADER #define NODE_HEADER
// type definitions typedef int ListItemType;
class Node { public: // constructors Node(); Node(const ListItemType &item); Node(const ListItemType &item, Node *nextNodePtr, Node *prevNodePtr);
// member functions void SetItem(const ListItemType &item); void SetNext(Node *nextNodePtr); void SetPrev(Node *prevNodePtr); ListItemType GetItem() const; Node* GetNext() const; Node* GetPrev() const;
private: ListItemType m_item; Node *m_next; Node *m_prev; };
#endif // NODE_HEADER
// clinkedlist.cpp
https://pastebin.com/gYtHCnwu
//node.cpp
https://pastebin.com/mbwwg9Gw
Step by Step Solution
There are 3 Steps involved in it
Step: 1
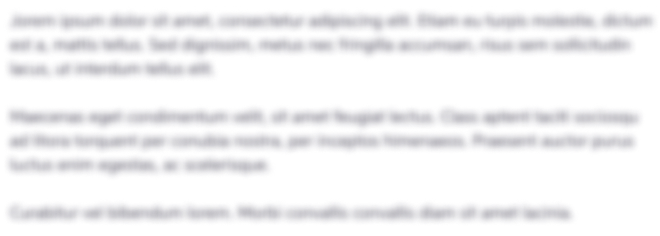
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started