Question
The next exercise is based on this implemetation for an UnorderedArrayList of integers: //Interface: ArrayListADT //works for int public interface ArrayListADT { public boolean isEmpty();
The next exercise is based on this implemetation for an UnorderedArrayList of integers: //Interface: ArrayListADT //works for int public interface ArrayListADT { public boolean isEmpty(); //Method to determine whether the list is empty. public boolean isFull(); //Method to determine whether the list is full. public int listSize(); //Method to return the number of elements in the list. public int maxListSize(); //Method to return the maximum size of the list. public void print(); //Method to output the elements of the list. public boolean isItemAtEqual(int location, int item); //Method to determine whether item is the same as the item in the list at location. public void insertAt(int location, int insertItem); //Method to insert insertItem in the list at the position public void insertEnd(int insertItem); //Method to insert insertItem at the end of the list. public void removeAt(int location); //Method to remove the item from the list at location. public int retrieveAt(int location); //Method to retrieve the element from the list at location. public void replaceAt(int location, int repItem); //Method to replace the element in the list at location with repItem. public void clearList(); //Method to remove all the elements from the list. public int search(int searchItem); //Method to determine whether searchItem is in the list. public void remove(int removeItem); //Method to remove an item from the list. }
//Class: ArrayListClass implements //Interface: ArrayListADT public abstract class ArrayListClass implements ArrayListADT { protected int length; //to store the length of the list protected int maxSize; //to store the maximum size of the list protected int[] list; //array to hold the list elements
//Default constructor public ArrayListClass() { maxSize = 100; length = 0; list = new int[maxSize]; }
//Alternate Constructor public ArrayListClass(int size) { if(size <= 0) { System.err.println("The array size must be positive. Creating an array of size 100."); maxSize = 100; } else maxSize = size; length = 0; list = new int[maxSize]; }
public boolean isEmpty() { return (length == 0); }
public boolean isFull() { return (length == maxSize); }
public int listSize() { return length; }
public int maxListSize() { return maxSize; }
public void print() { for (int i = 0; i < length; i++) System.out.print(list[i] + " "); System.out.println(); }
public boolean isItemAtEqual(int location, int item) { if (location < 0 || location >= length) { System.err.println("The location of the item to be compared is out of range."); return false; } return list[location]== item; }
public void clearList() { for (int i = 0; i < length; i++) list[i] = 0; length = 0; System.gc(); //invoke the Java garbage collector }
public void removeAt(int location) { if (location < 0 || location >= length) System.err.println("The location of the item to be removed is out of range."); else { for(int i = location; i < length - 1; i++) list[i] = list[i + 1]; length--; } }
public int retrieveAt(int location) { if (location < 0 || location >= length) { System.err.println("The location of the item to be retrieved is out of range."); return 0; } else return list[location]; }
public abstract void insertAt(int location, int insertItem); public abstract void insertEnd(int insertItem); public abstract void replaceAt(int location, int repItem); public abstract int search(int searchItem); public abstract void remove(int removeItem); }
//Class: UnorderedArrayList extends //Super class: ArrayListClass public class UnorderedArrayList extends ArrayListClass { public UnorderedArrayList() { super(); }
public UnorderedArrayList(int size) { super(size); }
//Bubble Sort public void bubbleSort() { for (int pass = 0; pass < length - 1; pass++) { for (int i = 0; i < length - 1; i++) { if (list[i] > list[i + 1]) { int temp = list[i]; list[i] = list[i + 1]; list[i + 1] = temp; } } } }
//implementation for abstract methods defined in ArrayListClass //unordered list --> linear search public int search(int searchItem) { for(int i = 0; i < length; i++) if(list[i] == searchItem) return i; return -1; }
public void insertAt(int location, int insertItem) { if (location < 0 || location >= maxSize) System.err.println("The position of the item to be inserted is out of range."); else if (length >= maxSize) System.err.println("Cannot insert in a full list."); else { for (int i = length; i > location; i--) list[i] = list[i - 1]; //shift right list[location] = insertItem; length++; } }
public void insertEnd(int insertItem) { if (length >= maxSize) System.err.println("Cannot insert in a full list."); else { list[length] = insertItem; length++; } }
public void replaceAt(int location, int repItem) { if (location < 0 || location >= length) System.err.println("The location of the item to be replaced is out of range."); else list[location] = repItem; }
public void remove(int removeItem) { int i; if (length == 0) System.err.println("Cannot delete from an empty list."); else { i = search(removeItem); if (i != -1) removeAt(i); else System.out.println("Cannot delete! The item to be deleted is not in the list."); } } }
1.1Add to class a new method called that should replace every integer of value with copies of itself. For example, if the list is: before the method is invoked, it should be after the method executes. Note that the method should remove from the list all 0s and negative values. Test the method using this client:
//Testing the method scaleByK added to the user created UnorderedArrayList class public class Lab8_1 { public static final int SIZE = 100; public static void main(String[] args) { UnorderedArrayList list = new UnorderedArrayList(SIZE); list.insertEnd(2); list.insertEnd(4); list.insertEnd(-2); list.insertEnd(5); list.insertEnd(3); list.insertEnd(0); list.insertEnd(7); System.out.println("The original list is: "); list.print(); System.out.println("The list after method call is: "); list.scaleByK(); list.print(); } }
1.2. Same problem. This time use the ArrayList class in Java. Write scaleByK as a client method and use the print method provided.
//Testing the method scaleByK using the Java ArrayList class import java.util.ArrayList; public class Lab8_2 { public static void main(String[] args) { ArrayList
public static ArrayList
ArrayList
...
list = tmpList;
return list;
}
public static void print(ArrayList
Step by Step Solution
There are 3 Steps involved in it
Step: 1
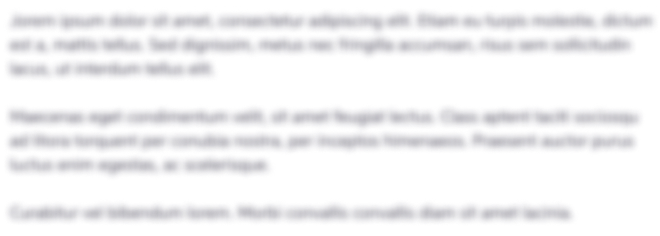
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started