Question
***The Node class and the Stack class are pre-written, and I've written the Queue class. Thus, all I need is the Main function.*** Node.h: template
***The Node class and the Stack class are pre-written, and I've written the Queue class. Thus, all I need is the Main function.***
Node.h:
templateclass Node { private: Object item; Node* next; public: Node(Object newItem) { item = newItem; next = nullptr; } Node(Object newItem, Node* nextNode) { item = newItem; next = nextNode; } void setItem(Object newItem) { item = newItem; } Object getItem() const { return item; } void setNext(Node* nextNode) { next = nextNode; } Node* getNext() const { return next; } };
Stack.h:
#ifndef NODEB_STACK_H #define NODEB_STACK_H #include "Node.h" #include using namespace std; templateclass Stack { private: Node* top; public: Stack() { top = nullptr; } ~Stack() { while (!isEmpty()) { pop(); } } bool isEmpty() const { return (top == nullptr); } void push(Object item) { if (isEmpty()) { Node* newNode = new Node(item); top = newNode; } else { Node* newNode = new Node(item, top); top = newNode; } } Object pop() { if (isEmpty()) { return Object(); } else { Object copy = top->getItem(); Node* topCopy = top; top = top->getNext(); delete topCopy; // Return the Object return copy; } } bool exists(Object item) const { Node* curr = top; while (curr != nullptr) { if (curr->getItem() == item) { return true; } curr = curr->getNext(); } return false; } void printStack() const { Node* curr = top; while (curr != nullptr) { cout getItem() getNext(); } } };
Queue.h:
#ifndef NODEB_QUEUE_H #define NODEB_QUEUE_H
/* * Queue class * Functionality: push, pop, peek, isEmpty, print */
#include "Node.h" #include
template
public: // Constructor Queue() { // Queue is empty. Top doesn't point at anything. top = nullptr; down = nullptr;
}
// Deconstructor / Destructor ~Queue() { // Pop all the Nodes left in the Queue while (!isEmpty()) { pop(); } }
bool isEmpty() const { return (top== nullptr); }
void push(Object item) { if (isEmpty()) { // This is the first Node in the Queue // Allocate memory in the heap to store the new Node Node
Object pop() { if (isEmpty()) { // There is no Node to pop. Return the default Object. // Note: This assumes the Object has a default constructor. return Object(); } else { // There is at least one Node in the Queue. // Store a copy of the Object to be returned Object copy = top->getItem(); // Store a copy of top Node
bool exists(Object item) const { Node
void printQueue() const { Node
#endif //NODEB_QUEUE_H
Now, the following code is from the "Project 1" that the directions reference. Basically, I wrote code that takes a text file containing information on different football players and their combine results and stores those players as a vector of objects of the class. Finally, I wrote a function that calculates the average weight of the players.
Combine.h:
#include #include #include #include #include #include using namespace std; class Combine { private: string name, college, pos; int height, weight; float dash, bench; public: Combine() { name = "John Smith"; college = "University"; pos = "player"; height = 0; weight = 0; dash = 0; bench = 0; } Combine(string name, string college, string pos, int height, int weight, float dash, float bench) { this->name = name; this->college = college; this->pos = pos; setHeight(height); setWeight(weight); setDash(dash); setBench(bench); } string getName() const { return name; } string getCollege() const { return college; } string getPos() const { return pos; } int getHeight() const { return height; } int getWeight() const { return weight; } float getDash() const { return dash; } float getBench() const { return bench; } void setName(string name) { this->name = name; } void setCollege(string college) { this->college = college; } void setPos(string pos) { this->pos = pos; } void setHeight(int height) { if (height //Default value this->height = 0; } else { this->height = height; } } void setWeight(int weight) { if (weight this->weight = 0; } else { this->weight = weight; } } void setDash(float dash) { if (dash this->dash = 0; } else { this->dash = dash; } } void setBench(float bench) { if (bench //Default value this->bench = 0; } else { this->bench = bench; } } friend ostream& operator void getPlayersFromFile(string filename, vector &players) { ifstream inFile; inFile.open("../" + filename); string name = "", college = "", pos = "", header = ""; string temp; int height = 0, weight = 0; float dash = 0, bench = 0; char comma = ','; if (inFile) { getline(inFile, header); while (inFile && inFile.peek() != EOF) { getline(inFile,temp); stringstream ss(temp); getline(ss,name,','); getline(ss,college,','); getline(ss,pos,','); getline(ss,temp,','); height = atoi(temp.c_str()); getline(ss,temp,','); weight = atoi(temp.c_str()); if(ss.good()) { getline(ss,temp,','); dash = atof(temp.c_str()); } else{ dash=0; } if(ss.good()) { getline(ss,temp,','); bench = atof(temp.c_str()); } else{ bench=0; } players.push_back(Combine(name, college, pos, height, weight, dash, bench)); } } else { cerr float avgWeight(vector &players) { float total = 0; getPlayersFromFile("CombinePlayers.txt", players); for (int i = 0; i } coutCombinePlayers.txt:
Name, College, POS, Height (in), Weight (lbs), 40 Yard, Bench Press Johnathan Abram, Mississippi State, S, 71, 205, 4.45, 0 Paul Adams, Missouri, OT, 78, 317, 5.18, 16 Nasir Adderley, Delaware, S, 72, 206, , 0 Azeez Al-Shaair, Florida Atlantic, LB, 73, 234, , 16 Otaro Alaka, Texas A&M, LB, 75, 239, 4.82, 20 Dakota Allen, Texas Tech, LB, 73, 232, 4.77, 23 Josh Allen, Kentucky, EDG, 77, 262, 4.63, 28 Zach Allen, Boston College, DE, 76, 281, 5, 24Stack Class Start with the Stack class from lecture. What is the Big-Oh complexity of the methods? Queue Class Create a Queue class that uses the Node class from lecture to create a functioning queue data structure. Your Queue should be able to push and pop Objects and determine if an Object is in the Queue. What is the complexity of each method? . Your Queue must be able to be used with any data type. . Your Nodes must be stored in heap memory. . Your program must not have any memory leaks. Main function Create three Queue objects: one of integers, one of strings, and one of a custom data type (ideally the type you created in Project 1). Demonstrate that the Queue methods work correctly by calling methods on the objects and printing out to the console when appropriate. Perform the following operations: Create a Queue object and a Stack object, both of the type you created in Project 1. Print and push the first 10 objects from your vector (from Project 1) onto the Queue. Pop the 10 objects off the Queue and push them onto the Stack. Pop and print the 10 objects off the Stack. What is the order of the objects before and after adding them to the Queue and Stack? When and why did it change? Design Consider the following questions: - Using the Node class, will the links point from the front to the back of the Queue or from the back to the front? - How will you make sure there are no memory leaks? - Which Nodes in the Queue do you need to keep track of? Is there still a top Node pointer? - How will you print the objects in the main function? Should you implement an operator? Stack Class Start with the Stack class from lecture. What is the Big-Oh complexity of the methods? Queue Class Create a Queue class that uses the Node class from lecture to create a functioning queue data structure. Your Queue should be able to push and pop Objects and determine if an Object is in the Queue. What is the complexity of each method? . Your Queue must be able to be used with any data type. . Your Nodes must be stored in heap memory. . Your program must not have any memory leaks. Main function Create three Queue objects: one of integers, one of strings, and one of a custom data type (ideally the type you created in Project 1). Demonstrate that the Queue methods work correctly by calling methods on the objects and printing out to the console when appropriate. Perform the following operations: Create a Queue object and a Stack object, both of the type you created in Project 1. Print and push the first 10 objects from your vector (from Project 1) onto the Queue. Pop the 10 objects off the Queue and push them onto the Stack. Pop and print the 10 objects off the Stack. What is the order of the objects before and after adding them to the Queue and Stack? When and why did it change? Design Consider the following questions: - Using the Node class, will the links point from the front to the back of the Queue or from the back to the front? - How will you make sure there are no memory leaks? - Which Nodes in the Queue do you need to keep track of? Is there still a top Node pointer? - How will you print the objects in the main function? Should you implement an operator
Step by Step Solution
There are 3 Steps involved in it
Step: 1
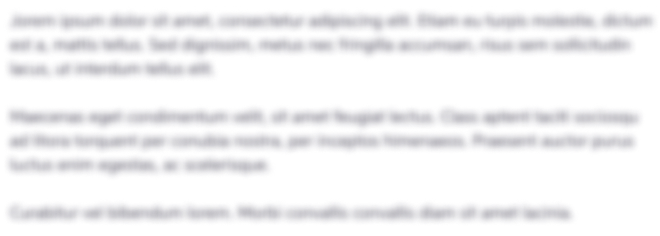
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started