Question
The objective of this assignment is for you to learn to correctly define a class, implement methods, and and test the defined class. The correctly
The objective of this assignment is for you to learn to correctly define a class, implement methods, and and test the defined class. The "correctly" is the catch to the assignment. You must have multiple constructors, set and get methods for every data member, and an appropriate class definition. Please closely examing the assessment sheet!
Define a class named Treadmill that contains the appropriate data members and methods. The class should have data members (what should they each be?) for:
Speed (0 - 12 MPH)
Incline (0 - 40%)
Time Exercised (upto one hour based on minutes and seconds)
Calories Burned
Use global constants to set the mins and maxes. The class should have mutator and accessor methods for EVERY data member listed above. The mutator method should ensure that the data members are "legally" set. In addition, these methods must be provided:
a mutator method to reset all data members to 0
a mutator method to calculate calories burned (assume a burn rate of 4 calories per minute or 4/60 per second)
an accessor method to print all data members
multiple constructors:Two parameters to set speed and inclinefour parameters to set all 4default initializer sets the speed to 3 and Incline to 0;
You must also write an application that uses this object (i.e, a main program that instantiates three treadmill objects and thoroughly tests the methods.) In order to test your class, you will create objects, show that every set and get works, the calculations work, and the print works The application must:
Define 3 treadmills in order to test all constructorsprint the values for each of the three treadmills to "prove" the instiantiation works
Test all set Methods by setting the datamamber then printing the data member useng the accessor method
Calculate the calories burned on all 3 treadsmills
Invoke the print method for all three teadmills at the end
Output should include:
Speed
Incline
Calories Burned
For example: Treadmill with maximum speed of 7.5 mph and maximum incline of 12.5 Client has exercised for 25 minutes and burned 100 calories
End of project question.
I worked a little bit on the c++ code, but I am having trouble with the output. t3 output works, but t1 and t2 are not.
here is my code so far: #include
#include
using namespace std;
struct timems
{
int min;
int seconds;
};
class treadmill
{
float speed;
float incline;
timems t;
float calories;
public:
treadmill()
{
speed = 3;
incline = 0;
}
//default initializer sets the speed to 3 and Incline to 0;
//Two parameters to set speed and incline
treadmill(float s, float i)
{
speed = s;
incline = i;
}
//Two parameters to set speed and incline
treadmill(float s, float i, float c, timems t1)
{
speed = s;
incline = i;
calories = c;
t.min = t1.min;
t.seconds = t1.seconds;
}
//four parameters to set all 4
//mutators
void mut_speed(float s)
{
if (s != 0)
this->speed = s;
}
void mut_incline(float s)
{
if (s != 0)
this->incline = s;
}
void mut_time(timems t1)
{
if (t1.min != 0 && t1.seconds != 0)
{
this->t.min = t1.min;
this->t.seconds = t1.seconds;
}
}
void mut_calories(float s)
{
if (s != 0)
calories = s;
}
//gets
float get_speed()
{
return this->speed;
}
float get_incline()
{
return this->incline;
}
timems get_time()
{
return this->t;
}
float get_calories()
{
return this->calories;
}
void setallzero()
{
this->speed = 0;
this->calories = 0;
this->incline = 0;
this->t.min = 0;
this->t.seconds = 0;
//set all data members to zero (a mutator method to reset all data members to 0)
}
float caloriesburned()
{
float def = 4.0 / 60.0;
//cout
int sec = (this->t.min) * 60 + this->t.seconds;
float a = (float)(sec*(def));
//cout
return a;
//a mutator method to calculate calories burned (assume a burn rate of 4 calories per minute or 4/60 per second)
}
void display()
{
cout speed
cout incline
cout calories
cout minutes:" t.min
cout seconds:" t.seconds
}
//an accessor method to print all data members
};
int main()
{
treadmill t1; // for default constructor
treadmill t2(3.0, 12); // for constructor with 2 parameters
timems ob;
ob.min = 23;
ob.seconds = 40;
treadmill t3(3.2, 12, 100, ob); //for constructor with 4 parameters
// test for display method for t1,t2,t3 objects to prove instantiation works
t1.display();
cout
t2.display();
cout
t3.display();
cout
//t1
// test for set methods for t1 object by calling all mutator functions
ob.min = 12;
ob.seconds = 34;
t1.mut_speed(4);
t1.mut_calories(100);
t1.mut_incline(22);
t1.mut_time(ob);
// test for get methods for object t1 by calling all accessor function
cout
cout
cout
cout
timems ob1 = t1.get_time();
cout
cout
// calling display function for object t1 after using set and get methods
t1.display();
// test for setallzero method for object t1
t1.setallzero();
// again printing to check if setallzero worked??
t1.display();
/*
//t2
// test for set methods for t2 object by calling all mutator functions
ob.min = 12;
ob.seconds = 34;
t2.mut_speed(4);
t2.mut_calories(100);
t2.mut_incline(22);
t2.mut_time(ob);
// test for get methods for object t2 by calling all accessor function
cout
cout
cout
cout
timems ob2 = t2.get_time();
cout
cout
// calling display function for object t1 after using set and get methods
t2.display();
// test for setallzero method for object t1
t2.setallzero();
// again printing to check if setallzero worked??
t2.display();
//t3
// test for set methods for t3 object by calling all mutator functions
ob.min = 12;
ob.seconds = 34;
t3.mut_speed(4);
t3.mut_calories(100);
t3.mut_incline(22);
t3.mut_time(ob);
// test for get methods for object t3 by calling all accessor function
cout
cout
cout
cout
timems ob3 = t3.get_time();
cout
cout
// calling display function for object t1 after using set and get methods
t3.display();
// test for setallzero method for object t3
t3.setallzero();
// again printing to check if setallzero worked??
t3.display();
*/
}
treadmill ine: s this->incline t.mint.seconds ninutes:-858993460 tine->secons:-858993460 2 2 2 speed:3 incline :12 calories:-1.07374e+08 time->ninutes:-858993460 tine->seconds:-88993460 2 9 speed:3.2 incline :12 calories:100 time->ninutes:23 tine-seconds:40 peed for ti is 4 incline for ti is 22 calories for t1 is 100 exercised time for t1 is 12 ninutes 34 seconds total calories burned is-50.2667 peed:4 incline :22Step by Step Solution
There are 3 Steps involved in it
Step: 1
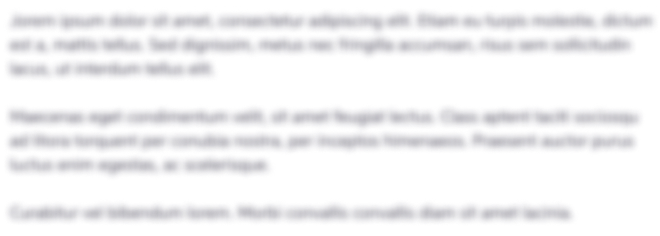
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started