Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The parseDouble method of class double may be a little too strict in the rules to convert a string to a double. Consider web
The parseDouble method of class double may be a little too strict in the rules to convert a string to a double. Consider web pages where a user can enter a value into a form. We may want to allow entry of numbers where the rules are more relaxed. We would like to enable a user to enter a numeric number (a sequence of digits) into the form allowing: Leading or trailing white space (any amout) Embedded single space (but not two spaces in a row) Optional '+' or '-', but if used must be the first non-white space character ('-' indicates a negative number) An optional decimal point (but only one decimal point - more than one would be invalid) The optional entry of commas (but only every third position to the left of the decimal point or end of the string if no decimal point is given, and must have at least one digit before a comma) . . Any other character than digits or those listed as valid above, is an error. Furthermore, not having at least one digit is an error. Write a method prepDouble in class StringUtils which return a String that can be parsed successfully by parseDouble. That is remove allowed white space and commas. If the string does not abide by the specified options then returns an "Invalid number" message as shown below. String "1995" "+3.99" "2,999,888.99" "- 6, 001. 02" "1 1 2 3 +1 2 3 4" "1 11 - 11 11 +"" "4.5.2" "452+" "23, 345, 12.34" "2345, 120" ", 356,120" "2,3456,120" "345.345, 560" "34A9" Value Returned "1995" "3.99" "2999888.99" "-6001.02" "123" "12" "Invalid number - embedded sequence of more than one space" "Invalid number - no value provided" "Invalid number - no value provided" "Invalid number - no value provided" "Invalid number - more that one decimal point" "Invalid number - misplaced + or "Invalid number - misplaced comma" "Invalid number - misplaced comma" "Invalid number - misplaced comma" "Invalid number - misplaced comma" "Invalid number - misplaced comma" "Invalid number - encountered A" The Main class provided receives a line of input and calls prepDouble. If the return string begins with "Invalid", the returned string is printed. Otherwise, parseDouble is used to convert the return string to a double and the returned doulbe is fromatted and printed with two decimal plactions below the decimal point. Suggested strategy - approach this problem incrementally. That is writing a small section of code and run the tests. Keep writing small sections of code and run the tests after coding each section. This should be the first section of code implemented and tested. Then: Add code to trim the leading and trailing whitespace. Add code to check if the input string is empty. If so, return the appropriate invalid number message. See if input string begins with a '-'. If so set a variable neg to "-". If not set neg to an empty string. Make sure to prepend the neg to the return string when then input passes all checks. Check If the input string begins with '-' or '+', remove this sign from the beginning of input sting. Recheck if the input string is now empty and if so return the appropriate invalid number message. Check if the input string has any '+' or '- signs remaining. If so return the appropriate invalid number message. Check if the input string has a sequence of two spaces. If so return the appropriate invalid number message. Remove all spaces from the input string. Now check for appropriate use of commas and decimal point. To do this first, break the input string into two strings: one containing all characters before a decimal point and another for characters after. If there is no decimal point consider all characters before the decimal point and no characters after. Then: Check if the after string contains a decimal point. If so, return the appropriate invalid number message. Check if the after string contains a comma. If so, return the appropriate invalid number message. Check to see if the before string matches a pattern that begins with one, two, or three digits followed by a comma and three digits where the pattern of a comma followed by three digits can be repeated one or more times. If so, remove all commas from the input string. . Check if the input string has a comma. If so, return the appropriate invalid number message. Check if the remaining input string contains a character other than digits or one decimal. If a non-digit or decimal point is found, return the appropriate invalid number message. The above can be solved using the String methods: contains trim .indexOf substring replaceAll length toCharArray matches Be careful to code the statements that modify the input string. Keep in mind that Strings are immutable. Methods like trim and replace all do not modify a String. Instead, they return a String. These methods when used should be in the context of an assignment statement where the variable referencing the input string is assign the returned String. File is marked as read only 1 import java.util.Scanner; 2 3 public class Main { 4 5 6 7 8 9 10 11 12 13 14 15 16 17 } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String line = scnr.nextLine(); String preppedLine StringUtils.prepNumber(line); if (preppedLine.startsWith("Invalid")) { System.out.println(preppedLine); } else { } Current file: Main.java } System.out.printf("%,. 2f", Double.parseDouble(preppedLine)); 1234567 00 1 public class StringUtils { // Add your code here 7} 8 Current file: StringUtils.java Load default template..
Step by Step Solution
★★★★★
3.32 Rating (152 Votes )
There are 3 Steps involved in it
Step: 1
public class StringUtils public static String prepDoubleString str String stripped strreplaceAlls replaceAll int len strippedlength if len 0 return In...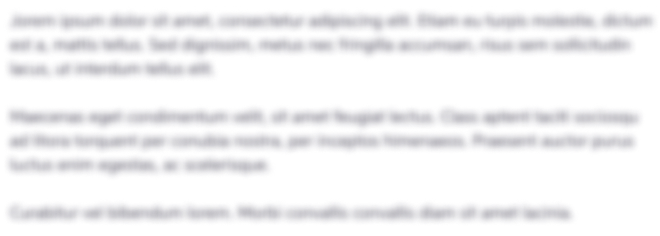
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started