Question
The Problem and Requirements In this project you implement a computer model for transactions on a debit card bank account. The transactions are carried out
The Problem and Requirements
In this project you implement a computer model for transactions on a debit card bank account. The transactions are carried out at an ATM machine. The program must perform the following functions.
Repeatedly asks if service is required until the answer is no; if so, the process terminates, otherwise:
A PIN is required from the client
If PIN is wrong, two more PIN input is offered: in case of all failed PIN input is closed and service required is asked
If PIN accepted:
The client is offered three options
Obtain a statement on the current balance
Deposit money to the account
Withdraw money from the account
In cases of (ii) and (iii) a statement is issued on the updated balance as well.
In order to initiate a process a client must input a PIN code. Having the transaction closed by a client, it is assumed that another client with another PIN code will be served next.
There has to be a Wallet folder in the C: drive or in your thumb drive such that it contains a file namedpin.txt. Every time a client requires transaction
A PIN number is generated and written into the pin.txt file. The PIN is a random selection between 1000 and 9999 (a four digit number)
A balance is generated and saved in a variable; balance is a random selection between 0 and 1000
When needed, the client must directly open the pin.txt file and read the PIN code; the program is not allowed to display the PIN
The dollar amount to be withdrawn is limited up to the current account balance.
Deposit can be made by inserting dollar bills of denominations 1, 2, 5, 10, 20, 50, 100.
Analysis and Design
Input values include
the selections of various options
the dollar bill values at a deposit
PIN
initial balance
Output values
messages as a result of a selected option the dollar value of the account balance
Pseudo-code
-- while service required (Figure 1) generate a PIN (Figure 2) generate balance
if transaction required (Figure 3) ask for PIN input (Figure 4)
if PIN correct
ask for a selection from options (i), (ii), (iii) (Figure 5)
issue balance statement (Figure 6)
repeatedly ask bills to deposit, input dollar values on the console update balance
issue a deposit statement
(Figures 7, 8, 9, 10)
ask the withdrawal amount (Figures 11, 12, 13, 14, 15) update balance
issue a withdraw statement
else
ask for PIN again, two more attempts allowed
if correct, do as above (Figures 16, 17) all attempts failed: new client (Figure 1)
--terminate the program (Figure 18)
Design of input output
Design of classes and methods
The Applications class contains the main method which in turn controls the logic of the program as described by the pseudo-code above. See the details about the main method below.
The Account class represents the bank account. The class contains all the data and operations necessary for the account in case of transactions
The ATM class represents the ATM machine. This class communicates with the client when a transaction is carried out. In order to execute the transactions this class must have access to the Account class methods
See the detailed descriptions below.
Account | |
DATA FIELDS | |
balance | Type: double; stores the current dollar value of the account |
pin | Type: int; stores the PIN code of the account, a four digit number randomly selected (in the main) |
METHODS | |
getPin() | Returns pin |
setPin() | void; takes an int parameter; assigns pin the parameter value |
setBalance() | void; takes a parameter; assigns balance the parameter value |
showBalance() | Return type String; returns a message for a balance statement (see Figure 6) This method does NOT print or display the balance. |
deposit( ) | void; takes a parameter for the deposit value; if the parameter is not negative, the method updates the balance; otherwise the balance not changed |
withdraw() | Return type String; takes a parameter for the amount of withdrawal; updates balance; returns a message of Figure 13 or Figure 15 depending on the size of the balance value relative to the requested withdraw. This method does NOT print or display any value. |
Account( ) | Default constructor |
ATM | |
DATA FIELDS | |
acc | an Account type reference; declare but do not instantiate |
keyboard | a Scanner reference; instantiated at declaration such as to read console data |
METHODS | |
ATM( ) | constructor; takes an Account type parameter to initialize the acc field |
select( ) transaction ( ) | void; private helper method called only in the transaction( ) method; declares two local variables of type String named selection and input; selection is assigned the message on the window of Figure 7; the method displays Figure 7 and the return value is stored in input; if input is null, the method calls itself, that is, the Cancel button cannot abandon this window; otherwise the method runs a switch structure controlled by input; the cases are balance, deposit, withdraw and default; case balance displays Figure 6, the message on Figure 6 window is the return value of the showBalance( ) method of the Account class, the method is called with the prefix acc; case deposit displays Figure 8, runs a while loop to solicit several dollar bills (see Figure 9); the loop runs as long as the input is admissible and it accumulates the dollar values; loop finished the deposit( ) method of the Account class is called to which the accumulated total is passed as a parameter value, then Figure 10 displays, the balance message is obtained by calling the showBalance( ) method of the Account class case withdraw declares a local variable money initialized to 0; displays Figure 12 and saves the return value in a String moneyString; if the string is null or empty, money assigned 0; otherwise money is assigned the parsed moneyString value; calls the withdraw( ) method of the Account class to which money is passed as a parameter value, the return value is saved in a String; displays Figure 15 (or 13) where the message is composed of the return value of withdraw( ) and the return value of showBalance( ) case default calls select( ), another self call. void; public; declares a local variable of type int named pinCounter initialized to 0; displays Figure 3 and saves the return value in a local variable confirm; if confirm is yes, runs a while loop (or do-while loop) controlled by pinCounter or empty or the parsed value is not the correct PIN, the method increments pinCounter and displays Figure 16 else break the loop; if pinCounter = =3, Figure 17 is displayed and a return terminates the method else selection( ) is called | |
Applications | ||
NO DATA FIELDS | ||
METHODS | ||
main( ) | declares and instantiates local reference variables rd of type Random, default constructor acc of type Account, default constructor atm of type ATM, constructor takes acc for parameter; declares a PrintWriter reference writer, not instantiated here; declares a boolean variable namedclient initialized to true; runs a while loop controlled by client, within the loop displays Figure 1 and saves the return value in a local int variable. if YES_OPTION calls setBalance( ) of the Account class with prefix acc and for parameter generates a random integer between 0 and 1000; generates a random PIN and saves it in a local variable pin; calls setPin( ) of the Account class; instantiates the PrintWriter variable writer with the file path; writer prints pin to the file and closes the file; Figure 2 displayed; the transaction( ) method of the ATM class is called with prefix atm else displays Figure 18 on the console; assigns client false to stop the loop and exit the process |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
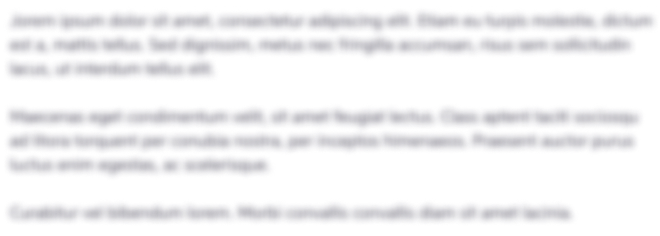
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started