Question
the program needs to be done with C# language , please help For today's lab you will be creating the start of a terminal application
the program needs to be done with C# language, please help
For today's lab you will be creating the start of a terminal application that might be used by a school to keep track of their courses, who is teaching them, and the students in the class. You will create a Person class that serves as the parent class for a Teacher class and a Student class. You will create a Course class that contains a Teacher and an array of Students. The program will feature a menu that has the option of creating a Course, creating a Teacher that will be assigned to the current course, creating students to fill the courses array of students, display the course, and exit the program. The program only has to keep track of a single Course.
Classes
Create a Person class
Person must have the following fields
a string for the persons name.
a string for the persons description.
an int for the persons age.
Create a Student class that inherits from Person
Student must have the following fields
an int for the Students grade
Create a Teacher class that inherits from Person
Teacher must have the following field
a string array for the Teachers knowledge
Create a Course class
Course must have the following fields
a string for the course title
a string for the course description
a Teacher for the teacher assigned to the course
a Student array for the students in the course
Constructors
Person needs a constructor that takes parameters to fill out all of its fields with
Teacher needs a constructor that takes in a parameter to fill its knowledge array
This constructor can take other parameters as well.
Student needs a constructor that takes in a parameter to fill its grade field
This constructor can take other parameters as well.
Course needs a constructor that takes in parameters to fill out the title, description, and number of students.
The number of students should be used to create the array to be held in the Student array field.
Menu
The menu must have the following options:
Create course - this option needs to prompt the user for the information needed to create a new Course object and assign that object to the currentCourse variable.
Create teacher - this option needs to prompt the user for the information needed to create a new Teacher object and assign that object to be the currentCourses teacher.
Add students - this option needs to prompt the user for the information needed to create enough students to fill the currentCourses Student array, create those students, and assign them to the currentCourses Student array.
Display - this option needs to display all of the information about the course, the teacher, and each of the students.
Exit - stop the program.
Main
In main before anything else you will need a Course variable to use for the currentCourse.
Program runs until the user chooses to exit.
Input Validation
The menu must handle the user typing the text of a selection or the number
Input should be handled in a case insensitive format
Ex: 1. Create course
The user should be able to choose the option 1, create course, or even CrEaTe CoUrSe.
Users input must be validated
The user must not be able to crash your program by entering invalid input
Step by Step Solution
There are 3 Steps involved in it
Step: 1
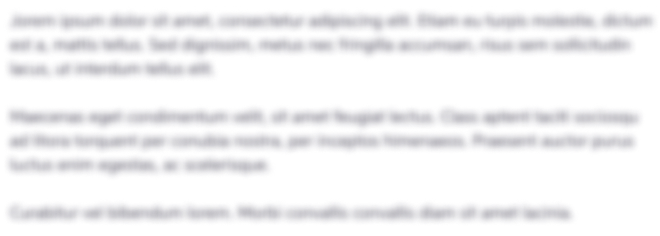
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started